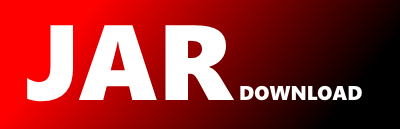
com.couchbase.client.java.query.AsyncN1qlQueryResult Maven / Gradle / Ivy
/*
* Copyright (c) 2016 Couchbase, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.couchbase.client.java.query;
import com.couchbase.client.core.annotations.InterfaceAudience;
import com.couchbase.client.core.annotations.InterfaceStability;
import com.couchbase.client.java.document.json.JsonObject;
import com.couchbase.client.java.document.json.JsonArray;
import rx.Observable;
/**
* A representation of an N1QL query result.
*
* @author Michael Nitschinger
*/
@InterfaceStability.Committed
@InterfaceAudience.Public
public interface AsyncN1qlQueryResult {
/**
* @return an async stream of each row resulting from the query (empty if fatal errors occurred).
*/
Observable rows();
/**
* @return an async single-item representing the signature of the results, that can be used to
* learn about the common structure of each {@link #rows() row}. This signature is usually a
* {@link JsonObject}, but could also be any JSON-valid type like a boolean scalar, {@link JsonArray}...
*/
Observable
© 2015 - 2025 Weber Informatics LLC | Privacy Policy