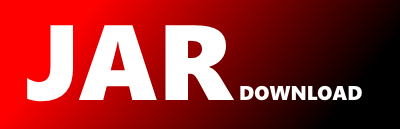
com.couchbase.client.java.manager.bucket.AsyncBucketManager Maven / Gradle / Ivy
Show all versions of java-client Show documentation
/*
* Copyright 2019 Couchbase, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.couchbase.client.java.manager.bucket;
import com.couchbase.client.core.CoreProtostellar;
import com.couchbase.client.core.annotation.Stability;
import com.couchbase.client.core.api.CoreCouchbaseOps;
import com.couchbase.client.core.error.BucketExistsException;
import com.couchbase.client.core.error.BucketNotFlushableException;
import com.couchbase.client.core.error.BucketNotFoundException;
import com.couchbase.client.core.error.CouchbaseException;
import com.couchbase.client.core.manager.CoreBucketManagerOps;
import com.couchbase.client.core.util.PreventsGarbageCollection;
import com.couchbase.client.java.AsyncCluster;
import java.util.Map;
import java.util.concurrent.CompletableFuture;
import static com.couchbase.client.core.util.CbCollections.transformValues;
import static com.couchbase.client.java.manager.bucket.CreateBucketOptions.createBucketOptions;
import static com.couchbase.client.java.manager.bucket.DropBucketOptions.dropBucketOptions;
import static com.couchbase.client.java.manager.bucket.FlushBucketOptions.flushBucketOptions;
import static com.couchbase.client.java.manager.bucket.GetAllBucketOptions.getAllBucketOptions;
import static com.couchbase.client.java.manager.bucket.GetBucketOptions.getBucketOptions;
import static com.couchbase.client.java.manager.bucket.UpdateBucketOptions.updateBucketOptions;
import static java.util.Objects.requireNonNull;
/**
* Performs (async) management operations on Buckets.
*
* All mutating operations on this manager are eventually consistent, which means that as soon as the call returns
* the operation is accepted by the server, but it does not mean that the operation has been applied to all nodes
* in the cluster yet. In the future, APIs will be provided which allow to assert the propagation state.
*/
public class AsyncBucketManager {
/**
* References the core-io bucket manager which abstracts common I/O functionality.
*/
private final CoreBucketManagerOps coreBucketManager;
@PreventsGarbageCollection
private final AsyncCluster cluster;
private final boolean isProtostellar;
/**
* Creates a new {@link AsyncBucketManager}.
*
* This API is not intended to be called by the user directly, use {@link AsyncCluster#buckets()}
* instead.
*/
@Stability.Internal
public AsyncBucketManager(
final CoreCouchbaseOps ops,
final AsyncCluster cluster
) {
isProtostellar = (ops instanceof CoreProtostellar);
this.coreBucketManager = ops.bucketManager();
this.cluster = requireNonNull(cluster);
}
/**
* Creates a new bucket on the server.
*
* The SDK will not perform any logical validation on correct combination of the settings - the server will return
* an error on invalid combinations. As an example, a magma bucket needs at least 1024 MiB of bucket quota - otherwise
* the server will reject it.
*
* @param settings the {@link BucketSettings} describing the properties of the bucket.
* @return a {@link CompletableFuture} completing when the operation is applied or has failed with an error.
* @throws BucketExistsException (async) if the bucket already exists.
* @throws CouchbaseException (async) if any other generic unhandled/unexpected errors.
*/
public CompletableFuture createBucket(final BucketSettings settings) {
return createBucket(settings, createBucketOptions());
}
/**
* Creates a new bucket on the server with custom options.
*
* The SDK will not perform any logical validation on correct combination of the settings - the server will return
* an error on invalid combinations. As an example, a magma bucket needs at least 1024 MiB of bucket quota - otherwise
* the server will reject it.
*
* @param settings the {@link BucketSettings} describing the properties of the bucket.
* @param options the custom options to apply.
* @return a {@link CompletableFuture} completing when the operation is applied or has failed with an error.
* @throws BucketExistsException (async) if the bucket already exists.
* @throws CouchbaseException (async) if any other generic unhandled/unexpected errors.
*/
public CompletableFuture createBucket(final BucketSettings settings, final CreateBucketOptions options) {
// JVMCBC-1395: Ideally we only be sending settings the user has specified.
// But for Classic we have always sent some fields (those in BucketSettings.createDefaults), and cannot change this
// without potential breakage.
// For Protostellar we have a clean slate to implement things correctly.
BucketSettings merged;
if (isProtostellar) {
merged = settings;
}
else {
BucketSettings base = BucketSettings.createDefaults(settings.name());
merged = BucketSettings.merge(base, settings);
}
return coreBucketManager.createBucket(merged.toCore(), options.build());
}
/**
* Updates the settings of a bucket which already exists.
*
* Not all properties of a bucket can be changed on an update. Notably, the following properties are ignored
* by the SDK on update and so will not produce an error but also not change anything on the server side:
*
* - {@link BucketSettings#name()}
* - {@link BucketSettings#bucketType(BucketType)}
* - {@link BucketSettings#conflictResolutionType(ConflictResolutionType)}
* - {@link BucketSettings#replicaIndexes(boolean)}
* - {@link BucketSettings#storageBackend(StorageBackend)}
*
*
* The SDK will not perform any logical validation on correct combination of the settings - the server will return
* an error on invalid combinations. As an example, a magma bucket needs at least 1024 MiB of bucket quota - otherwise
* the server will reject it.
*
* @param settings the {@link BucketSettings} describing the properties of the bucket.
* @return a {@link CompletableFuture} completing when the operation is applied or has failed with an error.
* @throws BucketNotFoundException (async) if the specified bucket does not exist.
* @throws CouchbaseException (async) if any other generic unhandled/unexpected errors.
*/
public CompletableFuture updateBucket(final BucketSettings settings) {
return updateBucket(settings, updateBucketOptions());
}
/**
* Updates the settings of a bucket which already exists with custom options.
*
* Not all properties of a bucket can be changed on an update. Notably, the following properties are ignored
* by the SDK on update and so will not produce an error but also not change anything on the server side:
*
* - {@link BucketSettings#name()}
* - {@link BucketSettings#bucketType(BucketType)}
* - {@link BucketSettings#conflictResolutionType(ConflictResolutionType)}
* - {@link BucketSettings#replicaIndexes(boolean)}
* - {@link BucketSettings#storageBackend(StorageBackend)}
*
*
* The SDK will not perform any logical validation on correct combination of the settings - the server will return
* an error on invalid combinations. As an example, a magma bucket needs at least 1024 MiB of bucket quota - otherwise
* the server will reject it.
*
* @param settings the {@link BucketSettings} describing the properties of the bucket.
* @param options the custom options to apply.
* @return a {@link CompletableFuture} completing when the operation is applied or has failed with an error.
* @throws BucketNotFoundException (async) if the specified bucket does not exist.
* @throws CouchbaseException (async) if any other generic unhandled/unexpected errors.
*/
public CompletableFuture updateBucket(final BucketSettings settings, final UpdateBucketOptions options) {
return coreBucketManager.updateBucket(settings.toCore(), options.build());
}
/**
* Drops ("deletes") a bucket from the cluster.
*
* @param bucketName the name of the bucket to drop.
* @return a {@link CompletableFuture} completing when the operation is applied or has failed with an error.
* @throws BucketNotFoundException (async) if the specified bucket does not exist.
* @throws CouchbaseException (async) if any other generic unhandled/unexpected errors.
*/
public CompletableFuture dropBucket(final String bucketName) {
return dropBucket(bucketName, dropBucketOptions());
}
/**
* Drops ("deletes") a bucket from the cluster with custom options.
*
* @param bucketName the name of the bucket to drop.
* @param options the custom options to apply.
* @return a {@link CompletableFuture} completing when the operation is applied or has failed with an error.
* @throws BucketNotFoundException (async) if the specified bucket does not exist.
* @throws CouchbaseException (async) if any other generic unhandled/unexpected errors.
*/
public CompletableFuture dropBucket(final String bucketName, final DropBucketOptions options) {
return coreBucketManager.dropBucket(bucketName, options.build());
}
/**
* Loads the properties of a bucket from the cluster.
*
* @param bucketName the name of the bucket for which the settings should be loaded.
* @return a {@link CompletableFuture} completing with the bucket settings or if the operation has failed.
* @throws BucketNotFoundException (async) if the specified bucket does not exist.
* @throws CouchbaseException (async) if any other generic unhandled/unexpected errors.
*/
public CompletableFuture getBucket(final String bucketName) {
return getBucket(bucketName, getBucketOptions());
}
/**
* Loads the properties of a bucket from the cluster with custom options.
*
* @param bucketName the name of the bucket for which the settings should be loaded.
* @param options the custom options to apply.
* @return a {@link CompletableFuture} completing with the bucket settings or if the operation has failed.
* @throws BucketNotFoundException (async) if the specified bucket does not exist.
* @throws CouchbaseException (async) if any other generic unhandled/unexpected errors.
*/
public CompletableFuture getBucket(final String bucketName, final GetBucketOptions options) {
return coreBucketManager.getBucket(bucketName, options.build()).thenApply(BucketSettings::new);
}
/**
* Loads the properties of all buckets the current user has access to from the cluster.
*
* @return a {@link CompletableFuture} completing with all bucket settings or if the operation has failed.
* @throws CouchbaseException (async) if any other generic unhandled/unexpected errors.
*/
public CompletableFuture