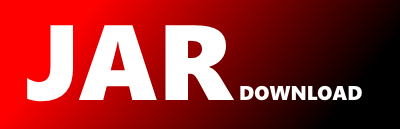
com.couchbase.client.java.manager.user.ReactiveUserManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-client Show documentation
Show all versions of java-client Show documentation
The official Couchbase Java SDK
/*
* Copyright 2019 Couchbase, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.couchbase.client.java.manager.user;
import com.couchbase.client.core.Reactor;
import reactor.core.publisher.Flux;
import reactor.core.publisher.Mono;
import static java.util.Objects.requireNonNull;
public class ReactiveUserManager {
private final AsyncUserManager async;
public ReactiveUserManager(AsyncUserManager async) {
this.async = requireNonNull(async);
}
public Mono getUser(AuthDomain domain, String username) {
return Reactor.toMono(() -> async.getUser(domain, username));
}
public Mono getUser(AuthDomain domain, String username, GetUserOptions options) {
return Reactor.toMono(() -> async.getUser(domain, username, options));
}
public Flux getAllUsers() {
return Reactor.toFlux(() -> async.getAllUsers());
}
public Flux getAllUsers(GetAllUsersOptions options) {
return Reactor.toFlux(() -> async.getAllUsers(options));
}
public Flux getRoles() {
return Reactor.toFlux(() -> async.getRoles());
}
public Flux getRoles(GetRolesOptions options) {
return Reactor.toFlux(() -> async.getRoles(options));
}
/**
* Changes the password of the currently authenticated user.
* SDK must be re-started and a new connection established after running, as the previous credentials will no longer
* be valid.
* @param newPassword String to replace the previous password with.
* @param options Common options (timeout, retry...)
*/
public Mono changePassword(String newPassword, ChangePasswordOptions options) {
return Reactor.toMono(() -> async.changePassword(newPassword, options));
}
/**
* Changes the password of the currently authenticated user.
* SDK must be re-started and a new connection established after running, as the previous credentials will no longer
* be valid.
* @param newPassword String to replace the previous password with.
*/
public Mono changePassword(String newPassword) { return Reactor.toMono(() -> async.changePassword(newPassword)); }
public Mono upsertUser(User user) {
return Reactor.toMono(() -> async.upsertUser(user));
}
public Mono upsertUser(User user, UpsertUserOptions options) {
return Reactor.toMono(() -> async.upsertUser(user, options));
}
public Mono dropUser(String username) {
return Reactor.toMono(() -> async.dropUser(username));
}
public Mono dropUser(String username, DropUserOptions options) {
return Reactor.toMono(() -> async.dropUser(username, options));
}
public Mono getGroup(String groupName) {
return Reactor.toMono(() -> async.getGroup(groupName));
}
public Mono getGroup(String groupName, GetGroupOptions options) {
return Reactor.toMono(() -> async.getGroup(groupName, options));
}
public Flux getAllGroups() {
return Reactor.toFlux(() -> async.getAllGroups());
}
public Flux getAllGroups(GetAllGroupsOptions options) {
return Reactor.toFlux(() -> async.getAllGroups(options));
}
public Mono upsertGroup(Group group) {
return Reactor.toMono(() -> async.upsertGroup(group));
}
public Mono upsertGroup(Group group, UpsertGroupOptions options) {
return Reactor.toMono(() -> async.upsertGroup(group, options));
}
public Mono dropGroup(String groupName) {
return Reactor.toMono(() -> async.dropGroup(groupName));
}
public Mono dropGroup(String groupName, DropGroupOptions options) {
return Reactor.toMono(() -> async.dropGroup(groupName, options));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy