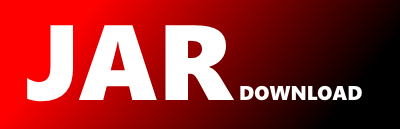
com.couchbase.spark.japi.CouchbaseRDD Maven / Gradle / Ivy
/*
* Copyright (c) 2015 Couchbase, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.couchbase.spark.japi;
import com.couchbase.client.java.document.Document;
import com.couchbase.client.java.document.JsonDocument;
import com.couchbase.spark.RDDFunctions;
import com.couchbase.spark.connection.SubdocLookupResult;
import com.couchbase.spark.connection.SubdocMutationResult;
import com.couchbase.spark.connection.SubdocMutationSpec;
import com.couchbase.spark.rdd.CouchbaseQueryRow;
import com.couchbase.spark.rdd.CouchbaseSpatialViewRow;
import com.couchbase.spark.rdd.CouchbaseViewRow;
import org.apache.spark.api.java.JavaRDD;
import org.apache.spark.rdd.RDD;
import scala.Predef;
import scala.concurrent.duration.Duration;
import scala.reflect.ClassTag;
import java.util.Collections;
import java.util.List;
import java.util.concurrent.TimeUnit;
public class CouchbaseRDD extends JavaRDD {
private final JavaRDD source;
private CouchbaseRDD(JavaRDD source, ClassTag classTag) {
super(source.rdd(), classTag);
this.source = source;
}
public static CouchbaseRDD couchbaseRDD(RDD source) {
return couchbaseRDD(source.toJavaRDD());
}
public static CouchbaseRDD couchbaseRDD(JavaRDD source) {
return new CouchbaseRDD(source, source.classTag());
}
/**
* Loads documents specified by the given document IDs.
*
* Note that this method can only be called from an RDD with type String, where the strings
* are the document IDs. Java is not able to check the instance of the generic type because
* of type erasure the same way as scala does.
*/
@SuppressWarnings({"unchecked"})
public JavaRDD couchbaseGet() {
return couchbaseGet(null, JsonDocument.class);
}
/**
* Loads documents specified by the given document IDs.
*
* Note that this method can only be called from an RDD with type String, where the strings
* are the document IDs. Java is not able to check the instance of the generic type because
* of type erasure the same way as scala does.
*
* @param bucket the name of the bucket.
*/
@SuppressWarnings({"unchecked"})
public JavaRDD couchbaseGet(String bucket) {
return couchbaseGet(bucket, JsonDocument.class);
}
/**
* Loads documents specified by the given document IDs.
*
* Note that this method can only be called from an RDD with type String, where the strings
* are the document IDs. Java is not able to check the instance of the generic type because
* of type erasure the same way as scala does.
*
* @param clazz the target document conversion class.
*/
@SuppressWarnings({"unchecked"})
public JavaRDD couchbaseGet(Class clazz) {
return couchbaseGet(null, clazz);
}
/**
* Loads documents specified by the given document IDs.
*
* Note that this method can only be called from an RDD with type String, where the strings
* are the document IDs. Java is not able to check the instance of the generic type because
* of type erasure the same way as scala does.
*/
@SuppressWarnings({"unchecked"})
public JavaRDD couchbaseGet(long timeout) {
return couchbaseGet(null, JsonDocument.class, timeout);
}
/**
* Loads documents specified by the given document IDs.
*
* Note that this method can only be called from an RDD with type String, where the strings
* are the document IDs. Java is not able to check the instance of the generic type because
* of type erasure the same way as scala does.
*
* @param bucket the name of the bucket.
*/
@SuppressWarnings({"unchecked"})
public JavaRDD couchbaseGet(String bucket, long timeout) {
return couchbaseGet(bucket, JsonDocument.class, timeout);
}
/**
* Loads documents specified by the given document IDs.
*
* Note that this method can only be called from an RDD with type String, where the strings
* are the document IDs. Java is not able to check the instance of the generic type because
* of type erasure the same way as scala does.
*
* @param clazz the target document conversion class.
*/
@SuppressWarnings({"unchecked"})
public JavaRDD couchbaseGet(Class clazz, long timeout) {
return couchbaseGet(null, clazz, timeout);
}
/**
* Loads documents specified by the given document IDs.
*
* Note that this method can only be called from an RDD with type String, where the strings
* are the document IDs. Java is not able to check the instance of the generic type because
* of type erasure the same way as scala does.
*
* @param bucket the name of the bucket.
* @param clazz the target document conversion class.
*/
@SuppressWarnings({"unchecked"})
public JavaRDD couchbaseGet(String bucket, Class clazz) {
return new RDDFunctions(source.rdd()).couchbaseGet(
bucket,
scala.Option.apply(null),
SparkUtil.classTag(clazz),
LCLIdentity.INSTANCE
).toJavaRDD();
}
/**
* Loads documents specified by the given document IDs.
*
* Note that this method can only be called from an RDD with type String, where the strings
* are the document IDs. Java is not able to check the instance of the generic type because
* of type erasure the same way as scala does.
*
* @param bucket the name of the bucket.
* @param clazz the target document conversion class.
*/
@SuppressWarnings({"unchecked"})
public JavaRDD couchbaseGet(String bucket, Class clazz, long timeout) {
return new RDDFunctions(source.rdd()).couchbaseGet(
bucket,
scala.Option.apply(Duration.create(timeout, TimeUnit.MILLISECONDS)),
SparkUtil.classTag(clazz),
LCLIdentity.INSTANCE
).toJavaRDD();
}
@SuppressWarnings({"unchecked"})
public JavaRDD couchbaseSubdocLookup(List get) {
return couchbaseSubdocLookup(get, Collections.emptyList(), null);
}
@SuppressWarnings({"unchecked"})
public JavaRDD couchbaseSubdocLookup(List get, List exists) {
return couchbaseSubdocLookup(get, exists, null);
}
@SuppressWarnings({"unchecked"})
public JavaRDD couchbaseSubdocLookup(List get, List exists, String bucket) {
return new RDDFunctions(source.rdd()).couchbaseSubdocLookup(
SparkUtil.listToSeq(get),
SparkUtil.listToSeq(exists),
bucket,
scala.Option.apply(null),
LCLIdentity.INSTANCE
).toJavaRDD();
}
@SuppressWarnings({"unchecked"})
public JavaRDD couchbaseSubdocLookup(List get, long timeout) {
return couchbaseSubdocLookup(get, Collections.emptyList(), null, timeout);
}
@SuppressWarnings({"unchecked"})
public JavaRDD couchbaseSubdocLookup(List get, List exists, long timeout) {
return couchbaseSubdocLookup(get, exists, null, timeout);
}
@SuppressWarnings({"unchecked"})
public JavaRDD couchbaseSubdocLookup(List get, List exists, String bucket, long timeout) {
return new RDDFunctions(source.rdd()).couchbaseSubdocLookup(
SparkUtil.listToSeq(get),
SparkUtil.listToSeq(exists),
bucket,
scala.Option.apply(Duration.create(timeout, TimeUnit.MILLISECONDS)),
LCLIdentity.INSTANCE
).toJavaRDD();
}
@SuppressWarnings({"unchecked"})
public JavaRDD couchbaseSubdocMutate(List specs) {
return couchbaseSubdocMutate(specs, null);
}
@SuppressWarnings({"unchecked"})
public JavaRDD couchbaseSubdocMutate(List specs, String bucket) {
return new RDDFunctions(source.rdd()).couchbaseSubdocMutate(
SparkUtil.listToSeq(specs),
bucket,
scala.Option.apply(null),
LCLIdentity.INSTANCE
).toJavaRDD();
}
@SuppressWarnings({"unchecked"})
public JavaRDD couchbaseSubdocMutate(List specs, long timeout) {
return couchbaseSubdocMutate(specs, null, timeout);
}
@SuppressWarnings({"unchecked"})
public JavaRDD couchbaseSubdocMutate(List specs, String bucket, long timeout) {
return new RDDFunctions(source.rdd()).couchbaseSubdocMutate(
SparkUtil.listToSeq(specs),
bucket,
scala.Option.apply(Duration.create(timeout, TimeUnit.MILLISECONDS)),
LCLIdentity.INSTANCE
).toJavaRDD();
}
@Override
public RDD rdd() {
return source.rdd();
}
@Override
public ClassTag classTag() {
return source.classTag();
}
@SuppressWarnings({"unchecked"})
public JavaRDD couchbaseView() {
return new RDDFunctions(source.rdd()).couchbaseView(null, scala.Option.apply(null), LCLIdentity.INSTANCE).toJavaRDD();
}
@SuppressWarnings({"unchecked"})
public JavaRDD couchbaseView(String bucket) {
return new RDDFunctions(source.rdd()).couchbaseView(bucket, scala.Option.apply(null), LCLIdentity.INSTANCE).toJavaRDD();
}
@SuppressWarnings({"unchecked"})
public JavaRDD couchbaseSpatialView() {
return new RDDFunctions(source.rdd()).couchbaseSpatialView(null, scala.Option.apply(null), LCLIdentity.INSTANCE).toJavaRDD();
}
@SuppressWarnings({"unchecked"})
public JavaRDD couchbaseSpatialView(String bucket) {
return new RDDFunctions(source.rdd()).couchbaseSpatialView(bucket, scala.Option.apply(null), LCLIdentity.INSTANCE).toJavaRDD();
}
@SuppressWarnings({"unchecked"})
public JavaRDD couchbaseQuery() {
return new RDDFunctions(source.rdd()).couchbaseQuery(null, scala.Option.apply(null), LCLIdentity.INSTANCE).toJavaRDD();
}
@SuppressWarnings({"unchecked"})
public JavaRDD couchbaseQuery(String bucket) {
return new RDDFunctions(source.rdd()).couchbaseQuery(bucket, scala.Option.apply(null), LCLIdentity.INSTANCE).toJavaRDD();
}
@SuppressWarnings({"unchecked"})
public JavaRDD couchbaseAnalytics() {
return new RDDFunctions(source.rdd()).couchbaseAnalytics(null, scala.Option.apply(null), LCLIdentity.INSTANCE).toJavaRDD();
}
@SuppressWarnings({"unchecked"})
public JavaRDD couchbaseAnalytics(String bucket) {
return new RDDFunctions(source.rdd()).couchbaseAnalytics(bucket, scala.Option.apply(null), LCLIdentity.INSTANCE).toJavaRDD();
}
@SuppressWarnings({"unchecked"})
public JavaRDD couchbaseView(long timeout) {
return new RDDFunctions(source.rdd()).couchbaseView(null, scala.Option.apply(Duration.create(timeout, TimeUnit.MILLISECONDS)), LCLIdentity.INSTANCE).toJavaRDD();
}
@SuppressWarnings({"unchecked"})
public JavaRDD couchbaseView(String bucket, long timeout) {
return new RDDFunctions(source.rdd()).couchbaseView(bucket, scala.Option.apply(Duration.create(timeout, TimeUnit.MILLISECONDS)), LCLIdentity.INSTANCE).toJavaRDD();
}
@SuppressWarnings({"unchecked"})
public JavaRDD couchbaseSpatialView(long timeout) {
return new RDDFunctions(source.rdd()).couchbaseSpatialView(null, scala.Option.apply(Duration.create(timeout, TimeUnit.MILLISECONDS)), LCLIdentity.INSTANCE).toJavaRDD();
}
@SuppressWarnings({"unchecked"})
public JavaRDD couchbaseSpatialView(String bucket, long timeout) {
return new RDDFunctions(source.rdd()).couchbaseSpatialView(bucket, scala.Option.apply(Duration.create(timeout, TimeUnit.MILLISECONDS)), LCLIdentity.INSTANCE).toJavaRDD();
}
@SuppressWarnings({"unchecked"})
public JavaRDD couchbaseQuery(long timeout) {
return new RDDFunctions(source.rdd()).couchbaseQuery(null, scala.Option.apply(Duration.create(timeout, TimeUnit.MILLISECONDS)), LCLIdentity.INSTANCE).toJavaRDD();
}
@SuppressWarnings({"unchecked"})
public JavaRDD couchbaseQuery(String bucket, long timeout) {
return new RDDFunctions(source.rdd()).couchbaseQuery(bucket, scala.Option.apply(Duration.create(timeout, TimeUnit.MILLISECONDS)), LCLIdentity.INSTANCE).toJavaRDD();
}
/**
* Calling scala from java is a mess.
*
* We'd be better off implementing the java interfaces from scala, at a later point.
*/
private static class LCLIdentity extends Predef.$less$colon$less {
public static LCLIdentity INSTANCE = new LCLIdentity();
@Override
public Object apply(Object v1) {
return v1;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy