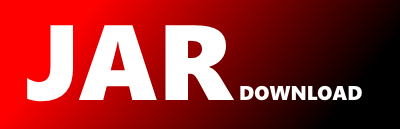
com.couchbase.lite.util.CollectionUtils Maven / Gradle / Ivy
package com.couchbase.lite.util;
import java.util.ArrayList;
import java.util.Collection;
/**
* Created by andy on 15/04/2014.
*/
public class CollectionUtils {
public static Collection filter(Collection target, Predicate predicate) {
Collection result = new ArrayList();
for (T element : target) {
if (predicate.apply(element)) {
result.add(element);
}
}
return result;
}
public static Collection transform(Collection target, Functor functor) {
Collection result = new ArrayList();
for (T element : target) {
U mapped = functor.invoke(element);
if (mapped != null) {
result.add(mapped);
}
}
return result;
}
public interface Predicate {
boolean apply(T type);
}
public interface Functor {
U invoke(T source);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy