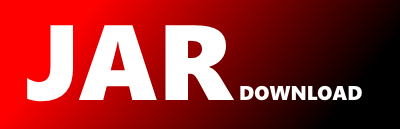
com.coveo.pushapiclient.BatchUpdate Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of push-api-client.java Show documentation
Show all versions of push-api-client.java Show documentation
Coveo Push API client. See more on https://github.com/coveo/push-api-client.java
The newest version!
package com.coveo.pushapiclient;
import com.google.gson.JsonObject;
import java.util.List;
import java.util.Objects;
/**
* See [Manage Batches of Items in a Push Source](https://docs.coveo.com/en/90)
*/
public class BatchUpdate {
private final List addOrUpdate;
private final List delete;
public BatchUpdate(List addOrUpdate, List delete) {
this.addOrUpdate = addOrUpdate;
this.delete = delete;
}
public BatchUpdateRecord marshal() {
return new BatchUpdateRecord(
this.addOrUpdate.stream().map(DocumentBuilder::marshalJsonObject).toArray(JsonObject[]::new),
this.delete.stream().map(DeleteDocument::marshalJsonObject).toArray(JsonObject[]::new)
);
}
public List getAddOrUpdate() {
return addOrUpdate;
}
public List getDelete() {
return delete;
}
@Override
public String toString() {
return "BatchUpdate[" +
"addOrUpdate=" + addOrUpdate +
", delete=" + delete +
']';
}
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null || getClass() != obj.getClass()) return false;
BatchUpdate that = (BatchUpdate) obj;
return addOrUpdate.equals(that.addOrUpdate) && delete.equals(that.delete);
}
@Override
public int hashCode() {
return Objects.hash(addOrUpdate, delete);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy