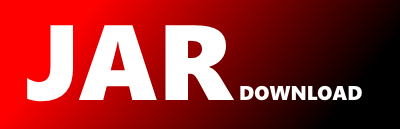
com.coveo.spillway.limit.Limit Maven / Gradle / Ivy
Show all versions of spillway Show documentation
/**
* The MIT License
* Copyright (c) 2016 Coveo
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
*/
package com.coveo.spillway.limit;
import java.time.Duration;
import java.util.List;
import java.util.Optional;
import java.util.Set;
import java.util.function.Function;
import com.coveo.spillway.limit.override.LimitOverride;
import com.coveo.spillway.trigger.LimitTrigger;
/**
* Contains all the the necessary information to enforce a limit
* specified by the user. This also includes the various call back
* when the capacity is reached.
*
* Should always be created using the {@link LimitBuilder}.
*
* @param The type of the context. String if not using a propertyExtractor
* ({@link LimitBuilder#of(String, java.util.function.Function)}).
* @see LimitBuilder
*
* @author Guillaume Simard
* @author Emile Fugulin
* @since 1.0.0
*/
public class Limit {
private LimitDefinition definition;
private boolean distributed;
private Function propertyExtractor;
private Set limitOverrides;
private List limitTriggers;
/*package*/ Limit(
LimitDefinition definition,
boolean distributed,
Function propertyExtractor,
Set limitOverrides,
List limitTriggers) {
this.definition = definition;
this.distributed = distributed;
this.propertyExtractor = propertyExtractor;
this.limitOverrides = limitOverrides;
this.limitTriggers = limitTriggers;
}
/**
* Simple getter for the base {@link LimitDefinition}.
*
* @return The base {@link LimitDefinition}
*/
public LimitDefinition getDefinition() {
return definition;
}
/**
* Getter for the {@link LimitDefinition} considering overrides.
*
* @param context Either the name of the limit OR the object on which the propertyExtractor ({@link LimitBuilder#of(String, java.util.function.Function)})
* will be applied if it was specified
* @return The found {@link LimitDefinition}
*/
public LimitDefinition getDefinition(T context) {
return findLimitOverride(context)
.map(p -> new LimitDefinition(getName(), p.getCapacity(), p.getExpiration()))
.orElse(getDefinition());
}
/**
* Simple getter for the {@link Limit}'s distributed flag.
* This flag is used to indicate if the limit will be shared between nodes.
* This is only relevant if using a storage with a cache.
*
* @return The value of the flag
*/
public boolean isDistributed() {
return distributed;
}
/**
* Simple getter for the base {@link LimitTrigger}s.
*
* @return A list of the {@link LimitTrigger}s
*/
public List getLimitTriggers() {
return limitTriggers;
}
/**
* Getter for the {@link LimitTrigger}s considering overrides.
*
* @param context Either the name of the limit OR the object on which the propertyExtractor ({@link LimitBuilder#of(String, java.util.function.Function)})
* will be applied if it was specified
* @return A list of the {@link LimitTrigger}s
*/
public List getLimitTriggers(T context) {
return findLimitOverride(context).map(p -> p.getLimitTriggers()).orElse(getLimitTriggers());
}
/**
* Getter used to extract the property from the context.
*
* @param context Either the name of the limit OR the object on which the propertyExtractor ({@link LimitBuilder#of(String, java.util.function.Function)})
* will be applied if it was specified
* @return The found property
*/
public String getProperty(T context) {
return propertyExtractor.apply(context);
}
/**
* Simple getter for the {@link Limit}'s name.
*
* @return The name of the {@link Limit}
*/
public String getName() {
return definition.getName();
}
/**
* Simple getter for the base limit's expiration.
*
* @return The expiration {@link Duration} of the limit
*/
public Duration getExpiration() {
return definition.getExpiration();
}
/**
* Getter for the expiration {@link Duration} considering overrides.
*
* @param context Either the name of the limit OR the object on which the propertyExtractor ({@link LimitBuilder#of(String, java.util.function.Function)})
* will be applied if it was specified
* @return The found expiration {@link Duration}
*/
public Duration getExpiration(T context) {
return findLimitOverride(context).map(p -> p.getExpiration()).orElse(getExpiration());
}
/**
* Simple getter for the base {@link Limit}'s capacity.
*
* @return The base capacity of the {@link Limit}
*/
public int getCapacity() {
return definition.getCapacity();
}
/**
* Getter for the capacity considering overrides.
*
* @param context Either the name of the limit OR the object on which the propertyExtractor ({@link LimitBuilder#of(String, java.util.function.Function)})
* will be applied if it was specified
* @return The found capacity
*/
public int getCapacity(T context) {
return findLimitOverride(context).map(p -> p.getCapacity()).orElse(getCapacity());
}
public Set getLimitOverrides() {
return limitOverrides;
}
@Override
public String toString() {
return definition.toString();
}
private Optional findLimitOverride(T context) {
String property = getProperty(context);
return limitOverrides
.stream()
.filter(override -> override.getProperty().equals(property))
.findAny();
}
}