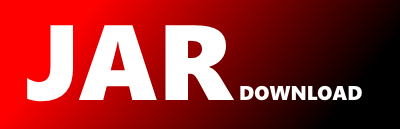
com.crashnote.core.util.FileUtil.jav Maven / Gradle / Ivy
///**
// * Copyright (C) 2011 - 101loops.com
// *
// * Licensed under the Apache License, Version 2.0 (the "License");
// * you may not use this file except in compliance with the License.
// * You may obtain a copy of the License at
// *
// * http://www.apache.org/licenses/LICENSE-2.0
// *
// * Unless required by applicable law or agreed to in writing, software
// * distributed under the License is distributed on an "AS IS" BASIS,
// * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// * See the License for the specific language governing permissions and
// * limitations under the License.
// */
//package com.crashnote.core.util;
//
//import com.crashnote.core.model.data.DataObject;
//
//import java.io.*;
//import java.util.Date;
//
///**
// * Utility class to execute basic file system operations like reading and writing
// */
//public class FileUtil2 {
//
// // INTERFACE ==================================================================================
//
// public String newChronoRandName() {
// return (new Date().getTime()) + "-" + Math.round(Math.random() * 100);
// }
//
// public File checked(final String path) {
// final File fileRef = new File(path);
// return fileRef.exists() ? fileRef : null;
// }
//
// public File getOrCreate(final File f) {
// if (!f.exists()) f.mkdirs();
// return f;
// }
//
// public boolean isValid(final File f) {
// return f.exists() || f.mkdir();
// }
//
// public boolean delete(final File f) {
// return f.delete();
// }
//
// // ======== PATH
//
// public static File getTmpFolder() {
// return new File(getTmpFolderPath());
// }
//
// public static String getTmpFolderPath() {
// return makeFolderPath(System.getProperty("java.io.tmpdir"));
// }
//
// private static String makeFolderPath(final String path) {
// if (!(path.endsWith("/") || path.endsWith("\\")))
// return path + System.getProperty("file.separator");
// else
// return path;
// }
//
// // ======== LISTING
//
// /**
// * Return a list of absolute file paths inside a directory -
// * the result can be null if file is not a directory!
// */
// public String[] listFiles(final File dir) {
// return dir.list();
// }
//
// public int countFiles(final File dir) {
// final String[] files = listFiles(dir);
// return (files != null) ? files.length : 0;
// }
//
// // ======== READ / WRITE
//
// public void writeToFile(final String path, final String data) throws IOException {
// writeToFile(new File(path), data);
// }
//
// public void writeToFile(final File file, final String data) throws IOException {
// streamToFile(new FileOutputStream(file), data);
// }
//
// public void writeToFile(final String path, final DataObject data) throws IOException {
// writeToFile(new File(path), data);
// }
//
// public void writeToFile(final File file, final DataObject data) throws IOException {
// streamToFile(new FileOutputStream(file), data);
// }
//
// public String readFromFile(final String path) throws IOException {
// return readFromFile(new File(path));
// }
//
// public String readFromFile(final File file) throws IOException {
// return readFromFile(new FileInputStream(file));
// }
//
// // INTERNALS ==================================================================================
//
// // ======== STREAMING
//
// private String readFromFile(final InputStream stream) throws IOException {
// Reader reader = null;
// final StringBuilder sb = new StringBuilder();
//
// try {
// reader = new BufferedReader(new InputStreamReader(stream));
//
// int len;
// final char[] chars = new char[4096];
// while ((len = reader.read(chars)) >= 0) {
// sb.append(chars, 0, len);
// }
// } finally {
// try {
// if (reader != null)
// reader.close();
// } catch (IOException ignored) {
// }
// }
//
// return sb.toString();
// }
//
// private void streamToFile(final OutputStream output, final String data) throws IOException {
// Writer writer = null;
// try {
// writer = new BufferedWriter(new OutputStreamWriter(output));
// writer.write(data);
// } finally {
// if (output != null) try {
// output.close();
// } catch (Exception ignore) {
// }
// if (writer != null) try {
// writer.flush();
// writer.close();
// } catch (Exception ignore) {
// }
// }
// }
//
// private void streamToFile(final OutputStream stream, final DataObject data) throws IOException {
// Writer writer = null;
// try {
// writer = new BufferedWriter(new OutputStreamWriter(stream));
// data.streamTo(writer);
// writer.flush();
// } finally {
// try {
// if (writer != null) {
// writer.close();
// }
// } catch (Exception ignored) {
// }
// }
// }
//}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy