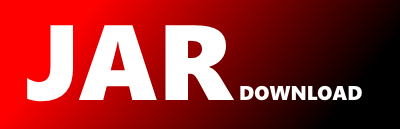
com.crashnote.core.config.Config2.jav Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of crashnote-appengine Show documentation
Show all versions of crashnote-appengine Show documentation
Reports exceptions from Java apps on Appengine to crashnote.com
///**
// * Copyright (C) 2011 - 101loops.com
// *
// * Licensed under the Apache License, Version 2.0 (the "License");
// * you may not use this file except in compliance with the License.
// * You may obtain a copy of the License at
// *
// * http://www.apache.org/licenses/LICENSE-2.0
// *
// * Unless required by applicable law or agreed to in writing, software
// * distributed under the License is distributed on an "AS IS" BASIS,
// * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// * See the License for the specific language governing permissions and
// * limitations under the License.
// */
//package com.crashnote.core.config;
//
//import com.crashnote.core.build.Builder;
//import com.crashnote.core.collect.Collector;
//import com.crashnote.core.log.*;
//import com.crashnote.core.model.types.*;
//import com.crashnote.core.report.Reporter;
//import com.crashnote.core.send.Sender;
//import com.crashnote.core.util.*;
//
//import java.io.File;
//import java.util.Properties;
//
///**
// * Main configuration object, home to all configurable settings of the notifier like
// * authentication and behaviour properties. It is initialized in the according appender/handler,
// * user customizations are applied and then each important class receives a copy.
// *
// * It assumes that each 'set' method receives a String and converts it to the actual data type by
// * manual parsing/converting, thus being independent of the way the concrete logger handles it.
// */
//public abstract class Config2>
// extends ConfigBase {
//
// protected SystemUtil sysUtil;
// private LogLogFactory logFactory;
//
// /**
// * Library settings property names
// */
// public static final String PROP_MODE = "mode";
// public static final String PROP_ASYNC = "async";
// public static final String PROP_DEBUG = "debug";
// public static final String PROP_ENABLED = "enabled";
// public static final String PROP_THREADS = "threads";
//
// public static final String PROP_APP_PROFILE = "app.profile";
// public static final String PROP_APP_VER = "app.version";
// public static final String PROP_APP_TYPE = "app.type";
// public static final String PROP_APP_PCKGS = "app.packages";
//
// public static final String PROP_REP_LEVEL = "report.level";
// public static final String PROP_REP_ENV = "report.env";
// public static final String PROP_REP_ENV_FILTER = "report.env.filter";
//
// public static final String PROP_EXCP_MAXLINES = "excp.maxlines";
// public static final String PROP_EXCP_NOCOMMON = "excp.nocommon";
//
// public static final String PROP_CACHE_DISK = "cache.disk";
// public static final String PROP_CACHE_DISK_DIR = "cache.disk.dir";
// public static final String PROP_CACHE_MEM = "cache.memory";
// public static final String PROP_CACHE_MEM_SIZE = "cache.memory.size";
//
// public static final String PROP_API_KEY = "api.key";
// public static final String PROP_API_HOST = "api.host";
// public static final String PROP_API_PORT = "api.port";
// public static final String PROP_API_PORT_SSL = "api.port.ssl";
// public static final String PROP_API_SECURE = "api.secure";
// public static final String PROP_API_TIMEOUT = "api.timeout";
//
// // SETUP ======================================================================================
//
// public Config2() {
// super();
// }
//
// public Config2(final Config2 c) {
// super(c);
// }
//
// public void initDefaults() {
//
// setAsync(true);
// setLogMode(LogMode.AUTO);
//
// // ==== REPORT
// setLogLevel(LogLevel.ERROR);
//
// //setReportHistoryEnabled(false);
// //setReportHistorySize(20);
// //setReportHistoryLvl(LogLevel.INFO);
//
// setIncludeEnvProperties(true);
// addEnvironmentFilter("PASSWORD");
// addEnvironmentFilter("AWS_SECRET_ACCESS_KEY");
//
// // ==== ERR
// setTraceLineLimit(20);
// setExcludeCommonFrames(true);
//
// // ==== API
// setPort(80);
// setSSLPort(443);
// setSecure(true);
// setHost("api.crashnote.com");
//
// // ==== SEND
// setConnectionTimeout(30);
//
// // ==== REPO
// setDiskCacheEnabled(true);
// setDiskCacheFileDir(new File(FileUtil.getTmpFolder(), Config.LIB_NAME.toLowerCase()));
//
// setMemoryCacheEnabled(true);
// setMemoryCacheSize(20);
// }
//
// // INTERFACE ==================================================================================
//
// public abstract Config2 copy();
//
// public String getClientInfo() {
// final Properties p = new ClassUtil().load("crashnote.about.properties");
// if (p.containsKey("name") && p.containsKey("version")) {
// return p.get("name") + ":" + p.get("version");
// } else
// return null;
// }
//
// // FACTORY ====================================================================================
//
// /**
// * Create an instance of module 'Reporter'
// */
// public Reporter getReporter() {
// return new Reporter(this);
// }
//
// /**
// * Create an instance of module 'Sender'
// */
// public Sender getSender() {
// return new Sender(this);
// }
//
// /**
// * Create an instance of module 'Collector'
// */
// public Collector getCollector() {
// return new Collector(this);
// }
//
// /**
// * Create an instance of module 'Builder'
// */
// public Builder getBuilder() {
// return new Builder();
// }
//
// /**
// * Create an instance of the system utility (share same instance, saves memory)
// */
// public SystemUtil getSystemUtil() {
// if (sysUtil == null) sysUtil = new SystemUtil();
// return sysUtil;
// }
//
// /**
// * Create an instance of a log factory
// */
// protected LogLogFactory getLogFactory() {
// if (logFactory == null) logFactory = new LogLogFactory(this);
// return logFactory;
// }
//
// public LogLog getLogger(final String name) {
// return getLogFactory().getLogger(name);
// }
//
// public LogLog getLogger(final Class clazz) {
// return getLogFactory().getLogger(clazz);
// }
//
// // INTERNALS ==================================================================================
//
// private String getBaseUrl() {
// final boolean ssl = isSecure();
// return (ssl ? "https://" : "http://") + getHost() + ":" + (ssl ? getSslPort() : getPort());
// }
//
// // GET+ =======================================================================================
//
// public String getValidateUrl() {
// return getBaseUrl() + "/validate/" + getKey();
// }
//
// public String getDataUrl(final LogType type) {
// return getBaseUrl() + "/" + type.getCode() + "?key=" + getKey();
// }
//
// public String getPingUrl() {
// return getBaseUrl();
// }
//
// public boolean sendToCloud() {
// return getLogMode() != LogMode.MANUAL;
// }
//
// public LogLevel getLogLevel() {
// final LogLevel maxLvl = LogLevel.DEBUG;
// final LogLevel reportLvl = getReportLogLevel();
// //final LogLevel historyLvl = getReportHistoryLevel();
// return LogLevel.getMaxLevel(maxLvl, reportLvl);
// }
//
// // GET ========================================================================================
//
// public boolean isAsync() {
// return getBoolSetting(PROP_ASYNC);
// }
//
// public boolean isDiskCacheEnabled() {
// return getBoolSetting(PROP_CACHE_DISK);
// }
//
// public boolean isMemoryCacheEnabled() {
// return getBoolSetting(PROP_CACHE_MEM);
// }
//
// public LogLevel getReportLogLevel() {
// return (LogLevel) getSetting(PROP_REP_LEVEL);
// }
//
// public String getKey() {
// return getStringSetting(PROP_API_KEY);
// }
//
// public boolean isEnabled() {
// return getBoolSetting(PROP_ENABLED);
// }
//
// public boolean getIncludeEnvProperties() {
// return getBoolSetting(PROP_REP_ENV);
// }
//
// public String[] getEnvironmentFilters() {
// final String filters = getStringSetting(PROP_REP_ENV_FILTER);
// if (filters == null || filters.length() == 0) return new String[0];
// else return filters.split(":");
// }
//
// public String getAppProfile() {
// return getStringSetting(PROP_APP_PROFILE);
// }
//
// public String getAppBasePackages() {
// return getStringSetting(PROP_APP_PCKGS);
// }
//
// public String getAppVersion() {
// return getStringSetting(PROP_APP_VER);
// }
//
// public int getConnectionTimeout() {
// return getIntSetting(PROP_API_TIMEOUT);
// }
//
// public Boolean getExcludeCommonFrames() {
// return getBoolSetting(PROP_EXCP_NOCOMMON);
// }
//
// public int getPort() {
// return getIntSetting(PROP_API_PORT);
// }
//
// public int getSslPort() {
// return getIntSetting(PROP_API_PORT_SSL);
// }
//
// public Boolean isSecure() {
// return getBoolSetting(PROP_API_SECURE);
// }
//
// public String getHost() {
// return getStringSetting(PROP_API_HOST);
// }
//
// public boolean isDebug() {
// return getBoolSetting(PROP_DEBUG);
// }
//
// public int getTraceLineLimit() {
// final int res = getIntSetting(PROP_EXCP_MAXLINES);
// return (res == -1) ? Integer.MAX_VALUE : res;
// }
//
// public LogMode getLogMode() {
// return (LogMode) getSetting(PROP_MODE);
// }
//
// public File getDiskCacheFileDir() {
// return (File) getSetting(PROP_CACHE_DISK_DIR);
// }
//
// public int getMemoryFileSize() {
// return getIntSetting(PROP_CACHE_MEM_SIZE);
// }
//
// public ApplicationType getApplicationType() {
// return (ApplicationType) getSetting(PROP_APP_TYPE);
// }
// // SET+ =======================================================================================
//
// public void addEnvironmentFilter(final String filter) {
// String filters = getStringSetting(PROP_REP_ENV_FILTER);
// if (filters == null || filters.length() == 0) filters = filter;
// else filters += ":" + filter;
// addSetting(PROP_REP_ENV_FILTER, filters);
// }
//
// // SET ========================================================================================
//
// public void setAsync(final String async) {
// addBoolSetting(PROP_ASYNC, async);
// }
//
// private void setAsync(final boolean async) {
// addSetting(PROP_ASYNC, async);
// }
//
// public void setIncludeEnvProperties(final boolean include) {
// addSetting(PROP_REP_ENV, include);
// }
//
// public void setEnabled(final String enabled) {
// addBoolSetting(PROP_ENABLED, enabled);
// }
//
// public void setSSLPort(final String sslPort) {
// addIntSetting(PROP_API_PORT_SSL, sslPort);
// }
//
// public void setSecure(final String secure) {
// addBoolSetting(PROP_API_SECURE, secure);
// }
//
// public void setAppProfile(final String profile) {
// addSetting(PROP_APP_PROFILE, profile);
// }
//
// public void setAppType(final ApplicationType type) {
// addSetting(PROP_APP_TYPE, type);
// }
//
// public void setPort(final int port) {
// addSetting(PROP_API_PORT, port);
// }
//
// public void setSSLPort(final int sslPort) {
// addSetting(PROP_API_PORT_SSL, sslPort);
// }
//
// public void setHost(final String host) {
// addSetting(PROP_API_HOST, host);
// }
//
// public void setDebug(final Boolean debug) {
// addSetting(PROP_DEBUG, debug);
// }
//
// public void setEnabled(final boolean enabled) {
// addSetting(PROP_ENABLED, enabled);
// }
//
// public void setExcludeCommonFrames(final boolean yes) {
// addSetting(PROP_EXCP_NOCOMMON, yes);
// }
//
// public void setSecure(final Boolean secure) {
// addSetting(PROP_API_SECURE, secure);
// }
//
// public void setKey(final String key) {
// addSetting(PROP_API_KEY, key);
// }
//
// public void setPort(final String port) {
// addIntSetting(PROP_API_PORT, port);
// }
//
// public void setDebug(final String debug) {
// addBoolSetting(PROP_DEBUG, debug);
// }
//
// public void setAppVersion(final String appVersion) {
// addSetting(PROP_APP_VER, appVersion);
// }
//
// public void setConnectionTimeout(final String timeout) {
// addIntSetting(PROP_API_TIMEOUT, timeout);
// }
//
// public void setConnectionTimeout(final int timeout) {
// addSetting(PROP_API_TIMEOUT, timeout);
// }
//
// public void setLogMode(final LogMode mode) {
// addSetting(PROP_MODE, mode);
// }
//
// public void setAppPackages(final String pckgs) {
// addSetting(PROP_APP_PCKGS, pckgs);
// }
//
// public void setLogLevel(final LogLevel level) {
// addSetting(PROP_REP_LEVEL, level);
// }
//
// public void setTraceLineLimit(final int lines) {
// addSetting(PROP_EXCP_MAXLINES, lines);
// }
//
// public void setDiskCacheEnabled(final String enabled) {
// addBoolSetting(PROP_CACHE_DISK, enabled);
// }
//
// public void setDiskCacheFileDir(final File dir) {
// addSetting(PROP_CACHE_DISK_DIR, dir);
// }
//
// public void setMemoryCacheEnabled(final String enabled) {
// addBoolSetting(PROP_CACHE_MEM, enabled);
// }
//
// public void setMemoryCacheSize(final String size) {
// addIntSetting(PROP_CACHE_MEM_SIZE, size);
// }
//
// public void setMemoryCacheSize(final int size) {
// addSetting(PROP_CACHE_MEM_SIZE, size);
// }
//
// public void setDiskCacheEnabled(final boolean enabled) {
// addSetting(PROP_CACHE_DISK, enabled);
// }
//
// public void setMemoryCacheEnabled(final boolean enabled) {
// addSetting(PROP_CACHE_MEM, enabled);
// }
//}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy