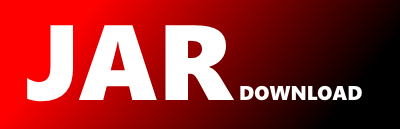
com.crashnote.core.model.data.PackageTrie.jav Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of crashnote-appengine Show documentation
Show all versions of crashnote-appengine Show documentation
Reports exceptions from Java apps on Appengine to crashnote.com
///**
// * Copyright (C) 2011 - 101loops.com
// *
// * Licensed under the Apache License, Version 2.0 (the "License");
// * you may not use this file except in compliance with the License.
// * You may obtain a copy of the License at
// *
// * http://www.apache.org/licenses/LICENSE-2.0
// *
// * Unless required by applicable law or agreed to in writing, software
// * distributed under the License is distributed on an "AS IS" BASIS,
// * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// * See the License for the specific language governing permissions and
// * limitations under the License.
// */
//package com.crashnote.core.model.data;
//
///**
// * FROM WIKIPEDIA:
// * "A trie, or prefix tree, is an ordered tree data structure that is used to store an associative
// * array where the keys are usually strings. Unlike a binary search tree, no node in the tree
// * stores the key associated with that node; instead, its position in the tree shows what key
// * it is associated with. All the descendants of a node have a common prefix of the string
// * associated with that node, and the root is associated with the empty string."
// *
// * This 'package' trie stores each part from a package path (e.g. org - apache - tomcat) in a node,
// * this way a package can be found very quickly.
// */
//public class PackageTrie {
//
// private final Node root;
//
// // SETUP =====================================================================================
//
// public PackageTrie() {
// root = new Node("");
// }
//
// // INTERFACE ==================================================================================
//
// public final void add(final String path) {
// addPckg(path.split("\\."));
// }
//
// public final String find(final String path) {
// return findPckg(path.split("\\."));
// }
//
// public final boolean has(final String path) {
// return find(path) != null;
// }
//
// // INTERNALS ==================================================================================
//
// private void addPckg(final String[] pckgs) {
// int offset = 0;
// Node root = this.root, last = null, next = root.child, parent = null;
// while (root != null) {
// last = null;
// parent = root;
// final String p = pckgs[offset];
// while (next != null) {
// final int comp = next.value.compareTo(p);
// if (comp == 0) { // match found, add remaining word to this node
// root = next;
// next = root.child;
// offset++;
// break;
// } else if (comp < 0) { // not found yet, continue searching
// last = next;
// next = next.next;
// } else { // won't find it anymore
// root = null;
// break;
// }
// }
// if (parent == root) break;
// }
//
// if (offset < pckgs.length) {
// // create a new node
// Node node = new Node(pckgs[offset]);
// if (last == null) { // insert at the beginning of the list
// parent.child = node;
// node.next = next;
// } else { // insert between last and next
// last.next = node;
// node.next = next;
// }
//
// // add remaining items
// for (int i = offset + 1; i < pckgs.length; i++) {
// node.child = new Node(pckgs[i]);
// node = node.child;
// }
// }
// }
//
// private String findPckg(final String[] pckgs) {
// int offset = 0;
// final StringBuilder sb = new StringBuilder();
// Node root = this.root;
// while (root != null && offset < pckgs.length) {
// final String p = pckgs[offset];
// Node next = root.child;
// root = null;
// while (next != null) {
// final int comp = next.value.compareTo(p);
// if (comp < 0) next = next.next;
// else if (comp == 0) {
// sb.append(".").append(next.value);
// root = next;
// next = null;
// offset++;
// } else return null;
// }
// if (root != null && root.isLast()) return sb.toString().substring(1);
// }
// return null;
// }
//
// private final class Node {
//
// public String value;
// public Node child;
// public Node next;
//
// public Node(final String value) {
// this.value = value;
// child = null;
// next = null;
// }
//
// public final boolean isLast() {
// return child == null;
// }
// }
//
//}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy