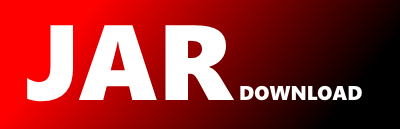
com.crashnote.core.send.Sender2.jav Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of crashnote-appengine Show documentation
Show all versions of crashnote-appengine Show documentation
Reports exceptions from Java apps on Appengine to crashnote.com
///**
// * Copyright (C) 2011 - 101loops.com
// *
// * Licensed under the Apache License, Version 2.0 (the "License");
// * you may not use this file except in compliance with the License.
// * You may obtain a copy of the License at
// *
// * http://www.apache.org/licenses/LICENSE-2.0
// *
// * Unless required by applicable law or agreed to in writing, software
// * distributed under the License is distributed on an "AS IS" BASIS,
// * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// * See the License for the specific language governing permissions and
// * limitations under the License.
// */
//package com.crashnote.core.send;
//
//import com.crashnote.core.Lifecycle;
//import com.crashnote.core.cache.Cache;
//import com.crashnote.core.config.*;
//import com.crashnote.core.log.LogLog;
//import com.crashnote.core.model.log.LogReport;
//import com.crashnote.core.model.types.*;
//import com.crashnote.core.send.impl.Connector;
//import com.crashnote.core.util.SystemUtil;
//
//import java.util.Date;
//
///**
// * This class handles is responsible for transmitting the crash report to the cloud server.
// *
// * To complete this task it uses a {@link com.crashnote.core.send.impl.Connector} that handles the connection to the
// * server and a {@link com.crashnote.core.cache.Cache} that holds reports that could not be transmitted yet/right now.
// */
//public class Sender2
// implements Lifecycle, IConfigChangeListener {
//
// private Date lastFlush;
// private boolean started;
//
// // configuration settings:
// private boolean async;
// private boolean retryOnFailure;
// private ApplicationType appType;
//
// private final LogLog logger;
// private final Cache cache;
// private final SystemUtil sysUtil;
// private final Connector connector;
//
// // SETUP ======================================================================================
//
// public Sender2(final C config) {
// updateConfig(config);
// this.cache = config.getCache();
// this.sysUtil = config.getSystemUtil();
// this.connector = createConnector(config);
// this.logger = config.getLogger(this.getClass());
// }
//
// public void updateConfig(final C config) {
// config.addListener(this);
// this.async = config.isAsync();
// this.appType = config.getApplicationType();
// this.retryOnFailure = config.getFailMode() == FailMode.RETRY;
// }
//
// // LIFECYCLE ==================================================================================
//
// public boolean start() {
// if (!started) {
// started = true;
// logger.debug("starting module [sender]");
//
// cache.start();
// connector.start();
// }
// return started;
// }
//
// public boolean stop() {
// if (started) {
// started = false;
// logger.debug("stopping module [sender]");
//
// cache.stop();
// connector.stop();
// }
// return started;
// }
//
// // INTERFACE ==================================================================================
//
// public void send(final LogReport report) {
// if (isAsync())
// cache(report); // ASYNC? -> move straight to cache
// else
// tryToSend(report); // SYNC? -> try to send
// }
//
// /*
// TODO: must be called regularly by async processor
// public void flush() {
// if (async && connector.isEnabled()) { // only flush cache if: async mode
// lastFlush = new Date();
//
// // just get up to 5 reports and transmit them
// final LogReport[] reports = cache.retrieve(5);
// if (reports != null)
// for (final LogReport r : reports)
// tryToSend(r);
// }
// }
// */
//
// // SHARED =====================================================================================
//
// protected void tryToSend(final LogReport report) {
// if (report != null) {
// // on the client: persist it as fast as possible 'cause it might be turned off any time
// if (getAppType() == ApplicationType.CLIENT) cache(report);
//
// if (sysUtil.isOffline()) {
// cache(report); // offline? then cache it directly, no need to try!
// } else {
// transmit(report); // is/might be online? transmit it!
// }
// }
// }
//
// protected final boolean transmit(final LogReport report) {
// final boolean success = connector.transmit(report);
// if (success) {
// uncache(report);
// } else {
// if (isRetryOnFailure())
// cache(report); // ... if retry is 'on': move data to cache
// else
// uncache(report); // ... otherwise: remove data from cache
// }
// return success;
// }
//
// protected final boolean uncache(final LogReport report) {
// return cache.remove(report);
// }
//
// protected final boolean cache(final LogReport report) {
// return cache.store(report);
// }
//
// // FACTORY ====================================================================================
//
// /**
// * Create a {@link com.crashnote.core.send.impl.Connector}
// *
// * @param config the configuration object
// * @return a new instance of a Connector
// */
// protected Connector createConnector(final C config) {
// return new Connector(config);
//
// /*
// if (getAppType() == ApplicationType.SERVER) {
// return new ConnectorSmart(config);
// } else {
// return new Connector(config);
// }
// */
// }
//
// // GET ========================================================================================
//
// public Date getLastFlush() {
// return lastFlush;
// }
//
// public Connector getConnector() {
// return connector;
// }
//
// public ApplicationType getAppType() {
// return appType;
// }
//
// public Boolean isRetryOnFailure() {
// return retryOnFailure;
// }
//
// public LogLog getLogger() {
// return logger;
// }
//
// public boolean isAsync() {
// return async;
// }
//}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy