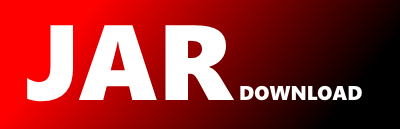
com.crashnote.core.send.impl.Dispatcher2.jav Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of crashnote-appengine Show documentation
Show all versions of crashnote-appengine Show documentation
Reports exceptions from Java apps on Appengine to crashnote.com
///**
// * Copyright (C) 2011 - 101loops.com
// *
// * Licensed under the Apache License, Version 2.0 (the "License");
// * you may not use this file except in compliance with the License.
// * You may obtain a copy of the License at
// *
// * http://www.apache.org/licenses/LICENSE-2.0
// *
// * Unless required by applicable law or agreed to in writing, software
// * distributed under the License is distributed on an "AS IS" BASIS,
// * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// * See the License for the specific language governing permissions and
// * limitations under the License.
// */
//package com.crashnote.core.send.impl;
//
//import com.crashnote.core.config.*;
//import com.crashnote.core.log.LogLog;
//import com.crashnote.core.model.excp.SendException;
//import com.crashnote.core.model.log.LogReport;
//import com.crashnote.core.model.types.LogType;
//
//import javax.net.ssl.*;
//import java.io.*;
//import java.net.*;
//import java.security.cert.X509Certificate;
//import java.util.zip.GZIPOutputStream;
//
///**
// * The Dispatcher is responsible for transmitting the data from the client to the server by
// * using Java's build-in capabilities around {@link java.net.HttpURLConnection}.
// */
//public class Dispatcher2
// implements IConfigChangeListener {
//
// // configuration settings:
// private String key;
// private String url_ping;
// private String url_envData;
// private String url_excpData;
// private String clientSignature;
// private int connectionTimeout;
//
// private final LogLog logger;
//
// // SETUP ======================================================================================
//
// public Dispatcher2(final C config) {
// updateConfig(config);
// this.logger = config.getLogger(this.getClass());
//
// // create and install a trust manager that does not validate certificate chains
// try {
// final SSLContext sc = SSLContext.getInstance("TLS");
// sc.init(null, createTrustManager(), new java.security.SecureRandom());
// HttpsURLConnection.setDefaultSSLSocketFactory(sc.getSocketFactory());
// } catch (Exception e) {
// logger.warn("unable to install custom SSL manager", e);
// }
// }
//
// public void updateConfig(final C config) {
// config.addListener(this);
// this.key = config.getKey();
// this.url_ping = config.getPingUrl();
// this.url_envData = config.getDataUrl(LogType.ENV);
// this.url_excpData = config.getDataUrl(LogType.ERR);
// this.clientSignature = config.getClientInfo();
// this.connectionTimeout = config.getConnectionTimeout() * 1000;
// }
//
// // INTERFACE ==================================================================================
//
// public void ping() throws SendException {
// final String url = getUrlPing();
// try {
// final int res = GET(url);
// if (res != 200) throw new SendException("http status code: " + res);
// } catch (Exception e) {
// throw new SendException(e);
// }
// }
//
// public void send(final LogReport report) throws SendException {
// try {
// final String url = (report.getTypeOf() == LogType.ENV) ? getUrlEnvData() : getUrlExcpData();
// POST(url, report);
// } catch (Exception e) {
// throw new SendException(e);
// }
// }
//
// /*
// public void validate() throws SendException, ValidateException {
// try {
// final int res = GET(getUrlValidate());
// if (res != 200) {
// if (res == 401)
// throw new ValidateException("the provided API Key [" + getKey() + "] is invalid");
// else if (res >= 500)
// throw new ValidateException("there was an unexpected error on the server");
// else
// throw new ValidateException("unable to let server validate the API Key");
// }
// } catch (IOException e) {
// throw new SendException(e);
// }
// }
// */
//
// // SHARED =====================================================================================
//
// protected int GET(final String url) throws IOException {
// int res = 0;
// logger.debug("GET {}", url);
// HttpURLConnection conn = null;
//
// try {
// conn = createConnection(url, "GET"); // prepare
// res = conn.getResponseCode(); // execute
// } finally {
// if (conn != null) conn.disconnect();
// }
// return res;
// }
//
// protected void POST(final String url, final LogReport report) throws IOException {
// logger.debug("POST {}", url);
//
// Writer writer = null;
// OutputStream out = null;
// HttpURLConnection conn = null;
//
// try {
// final byte[] data = report.toString().getBytes("UTF-8");
//
// // prepare connection
// conn = createConnection(url, "POST");
// //conn.setFixedLengthStreamingMode(data.length);
//
// // stream data to server
// out = createStream(conn);
// {
// out.write(data);
// out.flush();
// out.close();
//
// /* TODO: consider streaming directly from JSON Object
// if (!report.isRaw()) {
// //conn.setChunkedStreamingMode(0);
// writer = createWriter(out);
// report.getDataObj().streamTo(writer);
// writer.flush();
// writer.close();
// }*/
// }
// conn.getResponseCode();
//
// } catch (IOException e) {
// logger.error("POST failed", e);
// throw e; // re-throw
// } finally {
// // close connection
// //if (conn != null) conn.disconnect();
// }
// }
//
// protected HttpURLConnection createConnection(final String url, final String typeOf) throws IOException {
//
// HttpURLConnection.setFollowRedirects(true);
// final HttpURLConnection conn = createConnection(url);
// {
// conn.setDoOutput(true);
// conn.setUseCaches(false);
// conn.setRequestMethod(typeOf);
// conn.setAllowUserInteraction(false);
// conn.setReadTimeout(getConnectionTimeout());
// conn.setConnectTimeout(getConnectionTimeout());
// if (getClientSignature() != null)
// conn.setRequestProperty("User-Agent", getClientSignature());
// }
// return conn;
// }
//
// // FACTORY ====================================================================================
//
// protected HttpURLConnection createConnection(final String url) throws IOException {
// return (HttpURLConnection) new URL(url).openConnection();
// }
//
// protected OutputStream createStream(final HttpURLConnection conn) throws IOException {
//
// // data is gzipped JSON by default, so add the necessary request properties
// conn.setRequestProperty("Accept", "application/x-gzip");
// conn.setRequestProperty("Content-Type", "application/json");
// conn.setRequestProperty("Content-Encoding", "gzip");
//
// return new GZIPOutputStream(conn.getOutputStream());
// }
//
// protected Writer createWriter(final OutputStream stream) {
// return new OutputStreamWriter(stream);
// }
//
// protected TrustManager[] createTrustManager() {
// return new TrustManager[]{
// new X509TrustManager() {
// public X509Certificate[] getAcceptedIssuers() {
// return null;
// }
//
// public void checkClientTrusted(final X509Certificate[] certs, final String typeOf) {
// }
//
// public void checkServerTrusted(final X509Certificate[] certs, final String typeOf) {
// }
// }
// };
// }
//
// // GET ========================================================================================
//
// public String getKey() {
// return key;
// }
//
// public String getUrlPing() {
// return url_ping;
// }
//
// public String getUrlEnvData() {
// return url_envData;
// }
//
// public String getUrlExcpData() {
// return url_excpData;
// }
//
// public int getConnectionTimeout() {
// return connectionTimeout;
// }
//
// public LogLog getLogger() {
// return logger;
// }
//
// public String getClientSignature() {
// return clientSignature;
// }
//}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy