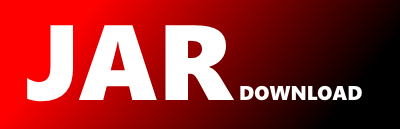
com.crashnote.core.util.ClassUtil.jav Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of crashnote-appengine Show documentation
Show all versions of crashnote-appengine Show documentation
Reports exceptions from Java apps on Appengine to crashnote.com
///**
// * Copyright (C) 2011 - 101loops.com
// *
// * Licensed under the Apache License, Version 2.0 (the "License");
// * you may not use this file except in compliance with the License.
// * You may obtain a copy of the License at
// *
// * http://www.apache.org/licenses/LICENSE-2.0
// *
// * Unless required by applicable law or agreed to in writing, software
// * distributed under the License is distributed on an "AS IS" BASIS,
// * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// * See the License for the specific language governing permissions and
// * limitations under the License.
// */
//package com.crashnote.core.util;
//
//import java.io.File;
//import java.net.URL;
//import java.util.Properties;
//import java.util.regex.Pattern;
//
///**
// * Utility class to execute classpath and package reading/parsing
// */
//public class ClassUtil1 {
//
// // ==== Packages
//
// public String[] splitPath(final String name) {
// final String packageName;
// final String className;
// final int lastDot = name.lastIndexOf('.');
// if (lastDot == -1) {
// packageName = "";
// className = name.substring(name.lastIndexOf('/') + 1);
// } else {
// packageName = name.substring(name.lastIndexOf('/') + 1, lastDot);
// className = name.substring(lastDot + 1);
// }
// return new String[]{packageName, className};
// }
//
// private String[] splitFilePath(final String path) {
// if (path.contains(File.separator))
// return path.split(Pattern.quote(File.separator));
// else {
// final String altSep = ("\\".equals(File.separator)) ? "/" : "\\";
// return path.split(Pattern.quote(altSep));
// }
// }
//
// public String[] getClasspathArray() {
// final String[] paths = System.getProperty("java.class.path", "").split(File.pathSeparator);
// final String[] res = new String[paths.length];
// for (int i = 0; i < paths.length; i++) {
// final String[] fp = splitFilePath(paths[i]); // split by file sep..
// final String fn = fp[fp.length - 1];
// res[i] = fn; // ..and only use last item (actual file name)
// }
// return res;
// }
//
// public String getClasspath() {
// final StringBuilder sb = new StringBuilder();
// for (final String e : getClasspathArray()) sb.append(e).append(":");
// return sb.toString();
// }
//
// // ==== ClassLoader
//
// /**
// * Find a resource of the specified name from the search path used to load classes.
// *
// * @param name The resource name
// * @return a bool indicating whether the resource was found
// */
// public Boolean resourceExists(final String name) {
// return ClassLoader.getSystemResource(name) != null;
// }
//
// /**
// * Load properties from classpath file
// */
// public Properties load(final String fileName) {
// final Properties p = new Properties();
// final URL url = ClassLoader.getSystemResource(fileName);
// try {
// p.load(url.openStream());
// } catch (Exception ignored) {
// }
// return p;
// }
//
// /**
// * Tests whether a class for a given name exists
// */
// public Boolean isClassOnPath(final String className) {
// try {
// Class.forName(className, false, ClassLoader.getSystemClassLoader());
// return true;
// } catch (ClassNotFoundException e) {
// return false;
// }
// }
//}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy