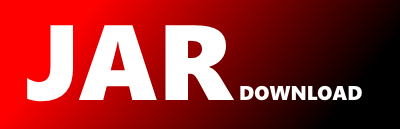
com.crashnote.core.send.ConnectorSmart.jav Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of crashnote-appengine Show documentation
Show all versions of crashnote-appengine Show documentation
Reports exceptions from Java apps on Appengine to crashnote.com
The newest version!
///**
// * Copyright (C) 2012 - 101loops.com
// *
// * Licensed under the Apache License, Version 2.0 (the "License");
// * you may not use this file except in compliance with the License.
// * You may obtain a copy of the License at
// *
// * http://www.apache.org/licenses/LICENSE-2.0
// *
// * Unless required by applicable law or agreed to in writing, software
// * distributed under the License is distributed on an "AS IS" BASIS,
// * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// * See the License for the specific language governing permissions and
// * limitations under the License.
// */
//package com.crashnote.core.send.impl;
//
//import com.crashnote.core.config.Config;
//import com.crashnote.core.util.ThrottleUtil;
//import com.crashnote.core.model.excp.*;
//import com.crashnote.core.model.log.LogReport;
//import com.crashnote.core.model.types.*;
//import com.crashnote.core.send.impl.Connector;
//
///**
// * Modification of the {@link com.crashnote.core.send.impl.Connector} which tries to manage the connection in a smart way.
// *
// * It establishes a connection with the server to validate the key, register the environment and
// * send the log data to it. It's also responsible for handling connection problems and throttle
// * the traffic activity.
// */
//
//public class ConnectorSmart
// extends Connector {
//
// private boolean active;
// private boolean started;
// private ConnectionStatus status;
//
// private LogReport env;
// private final ThrottleUtil throttle;
//
// // SETUP ======================================================================================
//
// public ConnectorSmart(final C config) {
// super(config);
//
// this.throttle = new ThrottleUtil();
// this.status = ConnectionStatus.NEW;
// }
//
// // LIFECYCLE ==================================================================================
//
// @Override
// public boolean start() {
// if (!started) {
// started = true;
// init();
// }
// return started;
// }
//
// // SHARED =====================================================================================
//
// @Override
// protected void doTransmit(final LogReport report) {
// if (isEnabled()) {
// try {
// if (report.getTypeOf() == LogType.ENV) env = report;
// if (init()) super.doTransmit(report);
// } catch (Exception e) {
// // send failed & was active? then add on 'interrupted' state
// if (active) newStatus(ConnectionStatus.INTERRUPTED);
// getLogger().error("sending failed", e);
// }
// }
// }
//
// // INTERNALS ==================================================================================
//
// private boolean init() {
// if (active) return true; // TODO: re-evaluate key after a while (like ~1/day maybe?)
// else {
// // not fully initialized yet, then do it - but throttle requests reasonably
// if (throttle.allow()) {
// throttle.reset();
// if (status == ConnectionStatus.INTERRUPTED) ping();
// if (status == ConnectionStatus.NEW) validateKey();
// if (status == ConnectionStatus.VALIDATED) registerEnv();
// return active;
// }
// return false;
// }
// }
//
// private void ping() {
// try {
// getDispatcher().ping();
// newStatus(ConnectionStatus.ACTIVE); // works? jump directly back to 'active'
// } catch (Exception e) {
// error(e);
// active = false;
// throttle.wait(1);
// }
// }
//
// private void validateKey() {
// try {
// getDispatcher().validate();
// newStatus(ConnectionStatus.VALIDATED);
// } catch (Exception e) {
// error(e);
// active = false;
// throttle.wait(60);
// }
// }
//
// private void registerEnv() {
// try {
// if (env != null) {
// getDispatcher().send(env);
// env = null; // 'delete'
// newStatus(ConnectionStatus.ACTIVE);
// }
// } catch (Exception e) {
// error(e);
// active = false;
// throttle.wait(10);
// }
// }
//
// private void error(final Exception e) {
// if (e instanceof SendException)
// getLogger().error("no connection to the Cloud could be established", e);
// else if (e instanceof ValidateException)
// getLogger().error("key validation failed", e);
// else
// getLogger().error("unexpected error occurred", e);
// }
//
// private void newStatus(final ConnectionStatus status) {
// active = (status == ConnectionStatus.ACTIVE);
// this.status = status;
// }
//
// // GET =======================================================================================
//
// public ConnectionStatus getStatus() {
// return status;
// }
//}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy