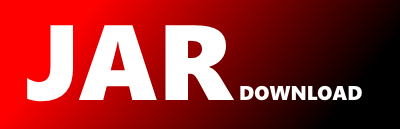
com.crashnote.core.config.helper.ConfigRenderOptions Maven / Gradle / Ivy
Show all versions of crashnote-servlet Show documentation
/**
* Copyright (C) 2011-2012 Typesafe Inc.
*/
package com.crashnote.core.config.helper;
/**
*
* A set of options related to rendering a {@link ConfigValue}. Passed to
* {@link ConfigValue#render(ConfigRenderOptions)}.
*
*
* Here is an example of creating a {@code ConfigRenderOptions}:
*
*
* ConfigRenderOptions options =
* ConfigRenderOptions.defaults().setComments(false)
*
*/
public final class ConfigRenderOptions {
private final boolean originComments;
private final boolean comments;
private final boolean formatted;
private ConfigRenderOptions(final boolean originComments, final boolean comments, final boolean formatted) {
this.originComments = originComments;
this.comments = comments;
this.formatted = formatted;
}
/**
* Returns the default render options which are verbose (commented and
* formatted). See {@link ConfigRenderOptions#concise} for stripped-down
* options. This rendering will not be valid JSON since it has comments.
*
* @return the default render options
*/
public static ConfigRenderOptions defaults() {
return new ConfigRenderOptions(true, true, true);
}
/**
* Returns concise render options (no whitespace or comments). For a
* resolved {@link Config}, the concise rendering will be valid JSON.
*
* @return the concise render options
*/
public static ConfigRenderOptions concise() {
return new ConfigRenderOptions(false, false, false);
}
/**
* Returns options with comments toggled. This controls human-written
* comments but not the autogenerated "origin of this setting" comments,
* which are controlled by {@link ConfigRenderOptions#setOriginComments}.
*
* @param value
* true to include comments in the render
* @return options with requested setting for comments
*/
public ConfigRenderOptions setComments(final boolean value) {
if (value == comments)
return this;
else
return new ConfigRenderOptions(originComments, value, formatted);
}
/**
* Returns whether the options enable comments. This method is mostly used
* by the config lib internally, not by applications.
*
* @return true if comments should be rendered
*/
public boolean getComments() {
return comments;
}
/**
* Returns options with origin comments toggled. If this is enabled, the
* library generates comments for each setting based on the
* {@link ConfigValue#origin} of that setting's value. For example these
* comments might tell you which file a setting comes from.
*
*
* {@code setOriginComments()} controls only these autogenerated
* "origin of this setting" comments, to toggle regular comments use
* {@link ConfigRenderOptions#setComments}.
*
* @param value
* true to include autogenerated setting-origin comments in the
* render
* @return options with origin comments toggled
*/
public ConfigRenderOptions setOriginComments(final boolean value) {
if (value == originComments)
return this;
else
return new ConfigRenderOptions(value, comments, formatted);
}
/**
* Returns whether the options enable automated origin comments. This method
* is mostly used by the config lib internally, not by applications.
*
* @return true if origin comments should be rendered
*/
public boolean getOriginComments() {
return originComments;
}
/**
* Returns options with formatting toggled. Formatting means indentation and
* whitespace, enabling formatting makes things prettier but larger.
*
* @param value
* true to include comments in the render
* @return options with requested setting for formatting
*/
public ConfigRenderOptions setFormatted(final boolean value) {
if (value == formatted)
return this;
else
return new ConfigRenderOptions(originComments, comments, value);
}
/**
* Returns whether the options enable formatting. This method is mostly used
* by the config lib internally, not by applications.
*
* @return true if comments should be rendered
*/
public boolean getFormatted() {
return formatted;
}
}