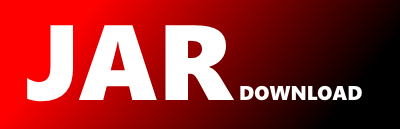
bali.notary.Notarization Maven / Gradle / Ivy
/************************************************************************
* Copyright (c) Crater Dog Technologies(TM). All Rights Reserved. *
************************************************************************
* DO NOT ALTER OR REMOVE COPYRIGHT NOTICES OR THIS FILE HEADER. *
* *
* This code is free software; you can redistribute it and/or modify it *
* under the terms of The MIT License (MIT), as published by the Open *
* Source Initiative. (See http://opensource.org/licenses/MIT) *
************************************************************************/
package bali.notary;
import java.net.URI;
import java.util.Map;
/**
* This interface defines the methods that must be implemented by all based digital notaries.
*
* @author Derk Norton
*/
public interface Notarization {
/**
* This method generates a new notary key consisting of an asymmetric (public/private) key pair
* based on the algorithm implemented by the specific notary implementation.
*
* @param passphrase The secret pass phrase used to unlock the private key.
* @return The newly generated notary key.
*/
NotaryKey generateKey(char[] passphrase);
/**
* This method enables the private notary key using the pass phrase
* that was used to generate the notary key.
*
* @param notaryKey The notary key to be enabled.
* @param passphrase The pass phrase used to generate the notary key.
* @return Whether or not the notary key was successfully enabled.
*/
boolean enableKey(NotaryKey notaryKey, char[] passphrase);
/**
* This method disables the private notary key so that it cannot be used
* to sign or decrypt documents.
*
* @param notaryKey The notary key to be disabled.
*/
void disableKey(NotaryKey notaryKey);
/**
* This method renews an existing notary key.
*
* @param key The notary key to be renewed.
* @param passphrase The secret pass phrase used to unlock the private key.
* @return The newly generated notary key.
*/
NotaryKey renewKey(NotaryKey key, char[] passphrase);
/**
* This method generates a notary seal from the specified new document using the specified
* notary key.
*
* @param The type of document to be notarized.
* @param document The document to be notarized.
* @param notaryKey The notary key used to notarize the document.
* @return The notarized document.
*/
Notarized notarizeNewDocument(D document, NotaryKey notaryKey);
/**
* This method generates a notary seal from the specified new version of a document using
* the specified notary key.
*
* @param The type of document to be notarized.
* @param document The new version of the document to be notarized.
* @param previousCitation A citation of the previous version of the document.
* @param notaryKey The notary key used to notarize the document.
* @return The notarized document.
*/
Notarized notarizeNextVersion(D document, Citation previousCitation, NotaryKey notaryKey);
/**
* This method generates a notary seal from the specified new version of a document using
* the specified notary key.
*
* @param The type of document to be notarized.
* @param document The new version of the document to be notarized.
* @param versionLevel The level of the version number to be incremented.
* @param previousCitation A citation of the previous version of the document.
* @param notaryKey The notary key used to notarize the document.
* @return The notarized document.
*/
Notarized notarizeNewSubVersion(D document, int versionLevel, Citation previousCitation, NotaryKey notaryKey);
/**
* This method uses the specified public verification key to verify that the specified
* digital seal is valid for the specified document.
*
* @param document The notarized document to be verified.
* @param certificate The verification certificate of the notary that signed the document.
* @param errors A map containing any errors that were found.
*/
void validateDocument(Notarized extends Document> document, Certificate certificate, Map errors);
/**
* This method generates a new citation using the document identification attributes and
* notarized document.
*
* @param reference A reference to the cited document.
* @param document The notarized document being cited.
* @return A new citation referring to the document.
*/
Citation generateCitation(URI reference, Notarized extends Document> document);
/**
* This method checks to see if the specified citation is valid.
*
* @param document The notarized document referenced by the citation.
* @param citation The citation to be validated.
* @param errors A map containing any errors that were found.
*/
void validateCitation(Notarized extends Document> document, Citation citation, Map errors);
/**
* This method checks to see if there are any errors and throws a validation exception
* containing the errors if there are.
*
* @param messageTag The message resource tag for the validation exception.
* @param errors The map of errors (and empty map means no errors).
* @throws ValidationException There were errors in the errors map.
*/
void throwExceptionOnErrors(String messageTag, Map errors) throws ValidationException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy