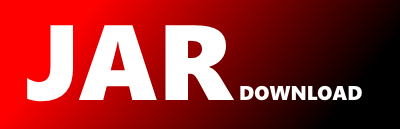
craterdog.identities.V1IdentificationProvider Maven / Gradle / Ivy
/************************************************************************
* Copyright (c) Crater Dog Technologies(TM). All Rights Reserved. *
************************************************************************
* DO NOT ALTER OR REMOVE COPYRIGHT NOTICES OR THIS FILE HEADER. *
* *
* This code is free software; you can redistribute it and/or modify it *
* under the terms of The MIT License (MIT), as published by the Open *
* Source Initiative. (See http://opensource.org/licenses/MIT) *
************************************************************************/
package craterdog.identities;
import craterdog.notary.Notarization;
import craterdog.notary.NotaryCertificate;
import craterdog.notary.NotaryKey;
import craterdog.notary.NotarySeal;
import craterdog.notary.V1NotarizationProvider;
import java.util.LinkedHashMap;
import java.util.Map;
import org.slf4j.ext.XLogger;
import org.slf4j.ext.XLoggerFactory;
/**
* This class implements a version 1 provider of the Identification
interface.
*
* @author Derk Norton
*/
public final class V1IdentificationProvider implements Identification {
static private final XLogger logger = XLoggerFactory.getXLogger(V1IdentificationProvider.class);
static private final Notarization notarizationProvider = new V1NotarizationProvider();
@Override
public DigitalIdentity createIdentity(NotaryKey notaryKey) {
logger.entry(notaryKey);
DigitalIdentity identity = createIdentity(null, notaryKey);
logger.exit(identity);
return identity;
}
@Override
public DigitalIdentity createIdentity(Map additionalAttributes, NotaryKey notaryKey) {
logger.entry(additionalAttributes, notaryKey);
logger.debug("Initializing the new identity attributes...");
IdentityAttributes attributes = new IdentityAttributes();
attributes.myLocation = notaryKey.verificationCertificate.attributes.identityLocation;
if (additionalAttributes != null) {
logger.debug("Adding additional attributes...");
for (Map.Entry attribute : additionalAttributes.entrySet()) {
attributes.put(attribute.getKey(), attribute.getValue());
}
}
attributes.watermark = notarizationProvider.generateWatermark(Notarization.VALID_FOR_FOREVER);
logger.debug("Validating the identity attributes...");
DigitalIdentity identity = new DigitalIdentity();
identity.attributes = attributes;
Map errors = new LinkedHashMap<>();
validateIdentityAttributes(identity, errors);
notarizationProvider.throwExceptionOnErrors("invalid.identity.attributes", errors);
logger.debug("Certifying the new identity using the notary key...");
identity.attributes = attributes;
identity.identitySeal = notarizationProvider.notarizeDocument("Identity Attributes", attributes.toString(), notaryKey);
logger.exit(identity);
return identity;
}
@Override
public void validateIdentity(DigitalIdentity identity, NotaryCertificate certificate, Map errors) {
logger.entry(identity, certificate, errors);
logger.debug("Validating the identity attributes...");
validateIdentityAttributes(identity, errors);
logger.debug("Validating the identity seal using the verification key...");
validateIdentitySeal(identity, certificate, errors);
logger.exit(errors);
}
private void validateIdentityAttributes(DigitalIdentity identity, Map errors) {
IdentityAttributes attributes = identity.attributes;
if (attributes == null) {
logger.error("The identity attributes are missing...");
errors.put("identity.attributes.are.missing", identity);
} else {
if (attributes.myLocation == null) {
logger.error("The identity location is missing...");
errors.put("identity.location.is.missing", identity);
}
if (attributes.watermark == null) {
logger.error("The identity watermark is missing...");
errors.put("identity.watermark.is.missing", identity);
}
}
}
private void validateIdentitySeal(DigitalIdentity identity, NotaryCertificate certificate, Map errors) {
NotarySeal seal = identity.identitySeal;
IdentityAttributes attributes = identity.attributes;
if (seal == null) {
logger.error("The identity seal is missing...");
errors.put("identity.seal.is.missing", identity);
} else if (attributes == null) {
logger.error("The identity attributes are missing...");
errors.put("identity.attributes.are.missing", identity);
} else {
String document = attributes.toString();
notarizationProvider.validateDocument(document, seal, certificate, errors);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy