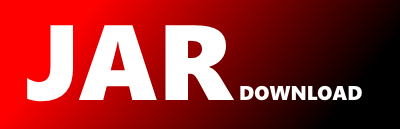
com.criteo.marketing.model.GoogleProduct Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of marketing.java-client Show documentation
Show all versions of marketing.java-client Show documentation
Criteo Marketing SDK for Java
/*
* Marketing API v.1.0
* IMPORTANT: This swagger links to Criteo production environment. Any test applied here will thus impact real campaigns.
*
* The version of the OpenAPI document: v.1.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.criteo.marketing.model;
import java.util.Objects;
import java.util.Arrays;
import com.criteo.marketing.model.Installment;
import com.criteo.marketing.model.LoyatyPoints;
import com.criteo.marketing.model.Price;
import com.criteo.marketing.model.Shipping;
import com.criteo.marketing.model.ShippingSize;
import com.criteo.marketing.model.Tax;
import com.criteo.marketing.model.UnitMeasure;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
/**
* GoogleProduct
*/
public class GoogleProduct {
public static final String SERIALIZED_NAME_ID = "id";
@SerializedName(SERIALIZED_NAME_ID)
private String id;
public static final String SERIALIZED_NAME_ADDITIONAL_IMAGE_LINK = "additionalImageLink";
@SerializedName(SERIALIZED_NAME_ADDITIONAL_IMAGE_LINK)
private String additionalImageLink;
public static final String SERIALIZED_NAME_ADULT = "adult";
@SerializedName(SERIALIZED_NAME_ADULT)
private Boolean adult;
public static final String SERIALIZED_NAME_ADWORD_REDIRECT = "adwordRedirect";
@SerializedName(SERIALIZED_NAME_ADWORD_REDIRECT)
private String adwordRedirect;
public static final String SERIALIZED_NAME_AGE_GROUP = "ageGroup";
@SerializedName(SERIALIZED_NAME_AGE_GROUP)
private String ageGroup;
public static final String SERIALIZED_NAME_AVAILABILITY = "availability";
@SerializedName(SERIALIZED_NAME_AVAILABILITY)
private String availability;
public static final String SERIALIZED_NAME_AVAILABILITY_DATE = "availabilityDate";
@SerializedName(SERIALIZED_NAME_AVAILABILITY_DATE)
private String availabilityDate;
public static final String SERIALIZED_NAME_BRAND = "brand";
@SerializedName(SERIALIZED_NAME_BRAND)
private String brand;
public static final String SERIALIZED_NAME_COLOR = "color";
@SerializedName(SERIALIZED_NAME_COLOR)
private String color;
public static final String SERIALIZED_NAME_CUSTOM_LABEL0 = "customLabel0";
@SerializedName(SERIALIZED_NAME_CUSTOM_LABEL0)
private String customLabel0;
public static final String SERIALIZED_NAME_CUSTOM_LABEL1 = "customLabel1";
@SerializedName(SERIALIZED_NAME_CUSTOM_LABEL1)
private String customLabel1;
public static final String SERIALIZED_NAME_CUSTOM_LABEL2 = "customLabel2";
@SerializedName(SERIALIZED_NAME_CUSTOM_LABEL2)
private String customLabel2;
public static final String SERIALIZED_NAME_CUSTOM_LABEL3 = "customLabel3";
@SerializedName(SERIALIZED_NAME_CUSTOM_LABEL3)
private String customLabel3;
public static final String SERIALIZED_NAME_CUSTOM_LABEL4 = "customLabel4";
@SerializedName(SERIALIZED_NAME_CUSTOM_LABEL4)
private String customLabel4;
public static final String SERIALIZED_NAME_DESCRIPTION = "description";
@SerializedName(SERIALIZED_NAME_DESCRIPTION)
private String description;
public static final String SERIALIZED_NAME_DISPLAY_ADS_ID = "displayAdsId";
@SerializedName(SERIALIZED_NAME_DISPLAY_ADS_ID)
private String displayAdsId;
public static final String SERIALIZED_NAME_DISPLAY_ADS_LINK = "displayAdsLink";
@SerializedName(SERIALIZED_NAME_DISPLAY_ADS_LINK)
private String displayAdsLink;
public static final String SERIALIZED_NAME_DISPLAY_ADS_SIMILAR_IDS = "displayAdsSimilarIds";
@SerializedName(SERIALIZED_NAME_DISPLAY_ADS_SIMILAR_IDS)
private List displayAdsSimilarIds = null;
public static final String SERIALIZED_NAME_DISPLAY_ADS_TITLE = "displayAdsTitle";
@SerializedName(SERIALIZED_NAME_DISPLAY_ADS_TITLE)
private String displayAdsTitle;
public static final String SERIALIZED_NAME_DISPLAY_ADS_VALUE = "displayAdsValue";
@SerializedName(SERIALIZED_NAME_DISPLAY_ADS_VALUE)
private String displayAdsValue;
public static final String SERIALIZED_NAME_ENERGY_EFFICIENCY_CLASS = "energyEfficiencyClass";
@SerializedName(SERIALIZED_NAME_ENERGY_EFFICIENCY_CLASS)
private String energyEfficiencyClass;
public static final String SERIALIZED_NAME_EXCLUDED_DESTINATION = "excludedDestination";
@SerializedName(SERIALIZED_NAME_EXCLUDED_DESTINATION)
private String excludedDestination;
public static final String SERIALIZED_NAME_EXPIRATION_DATE = "expirationDate";
@SerializedName(SERIALIZED_NAME_EXPIRATION_DATE)
private String expirationDate;
public static final String SERIALIZED_NAME_FILTERS = "filters";
@SerializedName(SERIALIZED_NAME_FILTERS)
private String filters;
public static final String SERIALIZED_NAME_GENDER = "gender";
@SerializedName(SERIALIZED_NAME_GENDER)
private String gender;
public static final String SERIALIZED_NAME_GOOGLE_PRODUCT_CATEGORY = "googleProductCategory";
@SerializedName(SERIALIZED_NAME_GOOGLE_PRODUCT_CATEGORY)
private String googleProductCategory;
public static final String SERIALIZED_NAME_GTIN = "gtin";
@SerializedName(SERIALIZED_NAME_GTIN)
private String gtin;
public static final String SERIALIZED_NAME_IDENTIFIER_EXISTS = "identifierExists";
@SerializedName(SERIALIZED_NAME_IDENTIFIER_EXISTS)
private Boolean identifierExists;
public static final String SERIALIZED_NAME_IMAGE_LINK = "imageLink";
@SerializedName(SERIALIZED_NAME_IMAGE_LINK)
private String imageLink;
public static final String SERIALIZED_NAME_INSTALLMENT = "installment";
@SerializedName(SERIALIZED_NAME_INSTALLMENT)
private Installment installment;
public static final String SERIALIZED_NAME_IS_BUNDLE = "isBundle";
@SerializedName(SERIALIZED_NAME_IS_BUNDLE)
private Boolean isBundle;
public static final String SERIALIZED_NAME_ITEM_GROUP_ID = "itemGroupId";
@SerializedName(SERIALIZED_NAME_ITEM_GROUP_ID)
private String itemGroupId;
public static final String SERIALIZED_NAME_LINK = "link";
@SerializedName(SERIALIZED_NAME_LINK)
private String link;
public static final String SERIALIZED_NAME_LOYATY_POINTS = "loyatyPoints";
@SerializedName(SERIALIZED_NAME_LOYATY_POINTS)
private LoyatyPoints loyatyPoints;
public static final String SERIALIZED_NAME_MATERIAL = "material";
@SerializedName(SERIALIZED_NAME_MATERIAL)
private String material;
public static final String SERIALIZED_NAME_MAX_HANDLING_TIME = "maxHandlingTime";
@SerializedName(SERIALIZED_NAME_MAX_HANDLING_TIME)
private Long maxHandlingTime;
public static final String SERIALIZED_NAME_MIN_ADVERTISER_PRICE = "minAdvertiserPrice";
@SerializedName(SERIALIZED_NAME_MIN_ADVERTISER_PRICE)
private Boolean minAdvertiserPrice;
public static final String SERIALIZED_NAME_MIN_HANDLING_TIME = "minHandlingTime";
@SerializedName(SERIALIZED_NAME_MIN_HANDLING_TIME)
private Long minHandlingTime;
public static final String SERIALIZED_NAME_MOBILE_LING = "mobileLing";
@SerializedName(SERIALIZED_NAME_MOBILE_LING)
private String mobileLing;
public static final String SERIALIZED_NAME_MODEL_NUMBER = "modelNumber";
@SerializedName(SERIALIZED_NAME_MODEL_NUMBER)
private String modelNumber;
public static final String SERIALIZED_NAME_MPN = "mpn";
@SerializedName(SERIALIZED_NAME_MPN)
private String mpn;
public static final String SERIALIZED_NAME_MULTIPACK = "multipack";
@SerializedName(SERIALIZED_NAME_MULTIPACK)
private Integer multipack;
public static final String SERIALIZED_NAME_NUMBER_OF_REVIEWS = "numberOfReviews";
@SerializedName(SERIALIZED_NAME_NUMBER_OF_REVIEWS)
private Integer numberOfReviews;
public static final String SERIALIZED_NAME_PATTERN = "pattern";
@SerializedName(SERIALIZED_NAME_PATTERN)
private String pattern;
public static final String SERIALIZED_NAME_PRICE = "price";
@SerializedName(SERIALIZED_NAME_PRICE)
private Price price;
public static final String SERIALIZED_NAME_PRODUCT_RATING = "productRating";
@SerializedName(SERIALIZED_NAME_PRODUCT_RATING)
private String productRating;
public static final String SERIALIZED_NAME_PRODUCT_TYPE = "productType";
@SerializedName(SERIALIZED_NAME_PRODUCT_TYPE)
private String productType;
public static final String SERIALIZED_NAME_PRODUCT_TYPE_KEY = "productTypeKey";
@SerializedName(SERIALIZED_NAME_PRODUCT_TYPE_KEY)
private String productTypeKey;
public static final String SERIALIZED_NAME_PROMO_TEXT = "promoText";
@SerializedName(SERIALIZED_NAME_PROMO_TEXT)
private String promoText;
public static final String SERIALIZED_NAME_PROMOTION_ID = "promotionId";
@SerializedName(SERIALIZED_NAME_PROMOTION_ID)
private String promotionId;
public static final String SERIALIZED_NAME_SALE_PRICE = "salePrice";
@SerializedName(SERIALIZED_NAME_SALE_PRICE)
private Price salePrice;
public static final String SERIALIZED_NAME_SALE_PRICE_EFFECTIVE_DATE = "salePriceEffectiveDate";
@SerializedName(SERIALIZED_NAME_SALE_PRICE_EFFECTIVE_DATE)
private String salePriceEffectiveDate;
public static final String SERIALIZED_NAME_SHIPPING = "shipping";
@SerializedName(SERIALIZED_NAME_SHIPPING)
private List shipping = null;
public static final String SERIALIZED_NAME_SHIPPING_HEIGHT = "shippingHeight";
@SerializedName(SERIALIZED_NAME_SHIPPING_HEIGHT)
private ShippingSize shippingHeight;
public static final String SERIALIZED_NAME_SHIPPING_LABEL = "shippingLabel";
@SerializedName(SERIALIZED_NAME_SHIPPING_LABEL)
private String shippingLabel;
public static final String SERIALIZED_NAME_SHIPPING_LENGTH = "shippingLength";
@SerializedName(SERIALIZED_NAME_SHIPPING_LENGTH)
private ShippingSize shippingLength;
public static final String SERIALIZED_NAME_SHIPPING_WEIGHT = "shippingWeight";
@SerializedName(SERIALIZED_NAME_SHIPPING_WEIGHT)
private UnitMeasure shippingWeight;
public static final String SERIALIZED_NAME_SHIPPING_WIDTH = "shippingWidth";
@SerializedName(SERIALIZED_NAME_SHIPPING_WIDTH)
private ShippingSize shippingWidth;
public static final String SERIALIZED_NAME_SIZE = "size";
@SerializedName(SERIALIZED_NAME_SIZE)
private String size;
public static final String SERIALIZED_NAME_SIZE_SYSTEM = "sizeSystem";
@SerializedName(SERIALIZED_NAME_SIZE_SYSTEM)
private String sizeSystem;
public static final String SERIALIZED_NAME_SIZE_TYPE = "sizeType";
@SerializedName(SERIALIZED_NAME_SIZE_TYPE)
private String sizeType;
public static final String SERIALIZED_NAME_TAX = "tax";
@SerializedName(SERIALIZED_NAME_TAX)
private List tax = null;
public static final String SERIALIZED_NAME_TITLE = "title";
@SerializedName(SERIALIZED_NAME_TITLE)
private String title;
public static final String SERIALIZED_NAME_UNIT_PRICING_BASE_MEASURE = "unitPricingBaseMeasure";
@SerializedName(SERIALIZED_NAME_UNIT_PRICING_BASE_MEASURE)
private UnitMeasure unitPricingBaseMeasure;
public static final String SERIALIZED_NAME_UNIT_PRICING_MEASURE = "unitPricingMeasure";
@SerializedName(SERIALIZED_NAME_UNIT_PRICING_MEASURE)
private UnitMeasure unitPricingMeasure;
public GoogleProduct id(String id) {
this.id = id;
return this;
}
/**
* Get id
* @return id
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public GoogleProduct additionalImageLink(String additionalImageLink) {
this.additionalImageLink = additionalImageLink;
return this;
}
/**
* Get additionalImageLink
* @return additionalImageLink
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getAdditionalImageLink() {
return additionalImageLink;
}
public void setAdditionalImageLink(String additionalImageLink) {
this.additionalImageLink = additionalImageLink;
}
public GoogleProduct adult(Boolean adult) {
this.adult = adult;
return this;
}
/**
* Get adult
* @return adult
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public Boolean getAdult() {
return adult;
}
public void setAdult(Boolean adult) {
this.adult = adult;
}
public GoogleProduct adwordRedirect(String adwordRedirect) {
this.adwordRedirect = adwordRedirect;
return this;
}
/**
* Get adwordRedirect
* @return adwordRedirect
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getAdwordRedirect() {
return adwordRedirect;
}
public void setAdwordRedirect(String adwordRedirect) {
this.adwordRedirect = adwordRedirect;
}
public GoogleProduct ageGroup(String ageGroup) {
this.ageGroup = ageGroup;
return this;
}
/**
* Get ageGroup
* @return ageGroup
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getAgeGroup() {
return ageGroup;
}
public void setAgeGroup(String ageGroup) {
this.ageGroup = ageGroup;
}
public GoogleProduct availability(String availability) {
this.availability = availability;
return this;
}
/**
* Get availability
* @return availability
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getAvailability() {
return availability;
}
public void setAvailability(String availability) {
this.availability = availability;
}
public GoogleProduct availabilityDate(String availabilityDate) {
this.availabilityDate = availabilityDate;
return this;
}
/**
* Get availabilityDate
* @return availabilityDate
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getAvailabilityDate() {
return availabilityDate;
}
public void setAvailabilityDate(String availabilityDate) {
this.availabilityDate = availabilityDate;
}
public GoogleProduct brand(String brand) {
this.brand = brand;
return this;
}
/**
* Get brand
* @return brand
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getBrand() {
return brand;
}
public void setBrand(String brand) {
this.brand = brand;
}
public GoogleProduct color(String color) {
this.color = color;
return this;
}
/**
* Get color
* @return color
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getColor() {
return color;
}
public void setColor(String color) {
this.color = color;
}
public GoogleProduct customLabel0(String customLabel0) {
this.customLabel0 = customLabel0;
return this;
}
/**
* Get customLabel0
* @return customLabel0
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getCustomLabel0() {
return customLabel0;
}
public void setCustomLabel0(String customLabel0) {
this.customLabel0 = customLabel0;
}
public GoogleProduct customLabel1(String customLabel1) {
this.customLabel1 = customLabel1;
return this;
}
/**
* Get customLabel1
* @return customLabel1
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getCustomLabel1() {
return customLabel1;
}
public void setCustomLabel1(String customLabel1) {
this.customLabel1 = customLabel1;
}
public GoogleProduct customLabel2(String customLabel2) {
this.customLabel2 = customLabel2;
return this;
}
/**
* Get customLabel2
* @return customLabel2
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getCustomLabel2() {
return customLabel2;
}
public void setCustomLabel2(String customLabel2) {
this.customLabel2 = customLabel2;
}
public GoogleProduct customLabel3(String customLabel3) {
this.customLabel3 = customLabel3;
return this;
}
/**
* Get customLabel3
* @return customLabel3
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getCustomLabel3() {
return customLabel3;
}
public void setCustomLabel3(String customLabel3) {
this.customLabel3 = customLabel3;
}
public GoogleProduct customLabel4(String customLabel4) {
this.customLabel4 = customLabel4;
return this;
}
/**
* Get customLabel4
* @return customLabel4
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getCustomLabel4() {
return customLabel4;
}
public void setCustomLabel4(String customLabel4) {
this.customLabel4 = customLabel4;
}
public GoogleProduct description(String description) {
this.description = description;
return this;
}
/**
* Get description
* @return description
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public GoogleProduct displayAdsId(String displayAdsId) {
this.displayAdsId = displayAdsId;
return this;
}
/**
* Get displayAdsId
* @return displayAdsId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getDisplayAdsId() {
return displayAdsId;
}
public void setDisplayAdsId(String displayAdsId) {
this.displayAdsId = displayAdsId;
}
public GoogleProduct displayAdsLink(String displayAdsLink) {
this.displayAdsLink = displayAdsLink;
return this;
}
/**
* Get displayAdsLink
* @return displayAdsLink
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getDisplayAdsLink() {
return displayAdsLink;
}
public void setDisplayAdsLink(String displayAdsLink) {
this.displayAdsLink = displayAdsLink;
}
public GoogleProduct displayAdsSimilarIds(List displayAdsSimilarIds) {
this.displayAdsSimilarIds = displayAdsSimilarIds;
return this;
}
public GoogleProduct addDisplayAdsSimilarIdsItem(String displayAdsSimilarIdsItem) {
if (this.displayAdsSimilarIds == null) {
this.displayAdsSimilarIds = new ArrayList<>();
}
this.displayAdsSimilarIds.add(displayAdsSimilarIdsItem);
return this;
}
/**
* Get displayAdsSimilarIds
* @return displayAdsSimilarIds
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public List getDisplayAdsSimilarIds() {
return displayAdsSimilarIds;
}
public void setDisplayAdsSimilarIds(List displayAdsSimilarIds) {
this.displayAdsSimilarIds = displayAdsSimilarIds;
}
public GoogleProduct displayAdsTitle(String displayAdsTitle) {
this.displayAdsTitle = displayAdsTitle;
return this;
}
/**
* Get displayAdsTitle
* @return displayAdsTitle
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getDisplayAdsTitle() {
return displayAdsTitle;
}
public void setDisplayAdsTitle(String displayAdsTitle) {
this.displayAdsTitle = displayAdsTitle;
}
public GoogleProduct displayAdsValue(String displayAdsValue) {
this.displayAdsValue = displayAdsValue;
return this;
}
/**
* Get displayAdsValue
* @return displayAdsValue
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getDisplayAdsValue() {
return displayAdsValue;
}
public void setDisplayAdsValue(String displayAdsValue) {
this.displayAdsValue = displayAdsValue;
}
public GoogleProduct energyEfficiencyClass(String energyEfficiencyClass) {
this.energyEfficiencyClass = energyEfficiencyClass;
return this;
}
/**
* Get energyEfficiencyClass
* @return energyEfficiencyClass
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getEnergyEfficiencyClass() {
return energyEfficiencyClass;
}
public void setEnergyEfficiencyClass(String energyEfficiencyClass) {
this.energyEfficiencyClass = energyEfficiencyClass;
}
public GoogleProduct excludedDestination(String excludedDestination) {
this.excludedDestination = excludedDestination;
return this;
}
/**
* Get excludedDestination
* @return excludedDestination
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getExcludedDestination() {
return excludedDestination;
}
public void setExcludedDestination(String excludedDestination) {
this.excludedDestination = excludedDestination;
}
public GoogleProduct expirationDate(String expirationDate) {
this.expirationDate = expirationDate;
return this;
}
/**
* Get expirationDate
* @return expirationDate
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getExpirationDate() {
return expirationDate;
}
public void setExpirationDate(String expirationDate) {
this.expirationDate = expirationDate;
}
public GoogleProduct filters(String filters) {
this.filters = filters;
return this;
}
/**
* Get filters
* @return filters
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getFilters() {
return filters;
}
public void setFilters(String filters) {
this.filters = filters;
}
public GoogleProduct gender(String gender) {
this.gender = gender;
return this;
}
/**
* Get gender
* @return gender
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getGender() {
return gender;
}
public void setGender(String gender) {
this.gender = gender;
}
public GoogleProduct googleProductCategory(String googleProductCategory) {
this.googleProductCategory = googleProductCategory;
return this;
}
/**
* Get googleProductCategory
* @return googleProductCategory
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getGoogleProductCategory() {
return googleProductCategory;
}
public void setGoogleProductCategory(String googleProductCategory) {
this.googleProductCategory = googleProductCategory;
}
public GoogleProduct gtin(String gtin) {
this.gtin = gtin;
return this;
}
/**
* Get gtin
* @return gtin
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getGtin() {
return gtin;
}
public void setGtin(String gtin) {
this.gtin = gtin;
}
public GoogleProduct identifierExists(Boolean identifierExists) {
this.identifierExists = identifierExists;
return this;
}
/**
* Get identifierExists
* @return identifierExists
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public Boolean getIdentifierExists() {
return identifierExists;
}
public void setIdentifierExists(Boolean identifierExists) {
this.identifierExists = identifierExists;
}
public GoogleProduct imageLink(String imageLink) {
this.imageLink = imageLink;
return this;
}
/**
* Get imageLink
* @return imageLink
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getImageLink() {
return imageLink;
}
public void setImageLink(String imageLink) {
this.imageLink = imageLink;
}
public GoogleProduct installment(Installment installment) {
this.installment = installment;
return this;
}
/**
* Get installment
* @return installment
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public Installment getInstallment() {
return installment;
}
public void setInstallment(Installment installment) {
this.installment = installment;
}
public GoogleProduct isBundle(Boolean isBundle) {
this.isBundle = isBundle;
return this;
}
/**
* Get isBundle
* @return isBundle
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public Boolean getIsBundle() {
return isBundle;
}
public void setIsBundle(Boolean isBundle) {
this.isBundle = isBundle;
}
public GoogleProduct itemGroupId(String itemGroupId) {
this.itemGroupId = itemGroupId;
return this;
}
/**
* Get itemGroupId
* @return itemGroupId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getItemGroupId() {
return itemGroupId;
}
public void setItemGroupId(String itemGroupId) {
this.itemGroupId = itemGroupId;
}
public GoogleProduct link(String link) {
this.link = link;
return this;
}
/**
* Get link
* @return link
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getLink() {
return link;
}
public void setLink(String link) {
this.link = link;
}
public GoogleProduct loyatyPoints(LoyatyPoints loyatyPoints) {
this.loyatyPoints = loyatyPoints;
return this;
}
/**
* Get loyatyPoints
* @return loyatyPoints
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public LoyatyPoints getLoyatyPoints() {
return loyatyPoints;
}
public void setLoyatyPoints(LoyatyPoints loyatyPoints) {
this.loyatyPoints = loyatyPoints;
}
public GoogleProduct material(String material) {
this.material = material;
return this;
}
/**
* Get material
* @return material
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getMaterial() {
return material;
}
public void setMaterial(String material) {
this.material = material;
}
public GoogleProduct maxHandlingTime(Long maxHandlingTime) {
this.maxHandlingTime = maxHandlingTime;
return this;
}
/**
* Get maxHandlingTime
* @return maxHandlingTime
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public Long getMaxHandlingTime() {
return maxHandlingTime;
}
public void setMaxHandlingTime(Long maxHandlingTime) {
this.maxHandlingTime = maxHandlingTime;
}
public GoogleProduct minAdvertiserPrice(Boolean minAdvertiserPrice) {
this.minAdvertiserPrice = minAdvertiserPrice;
return this;
}
/**
* Get minAdvertiserPrice
* @return minAdvertiserPrice
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public Boolean getMinAdvertiserPrice() {
return minAdvertiserPrice;
}
public void setMinAdvertiserPrice(Boolean minAdvertiserPrice) {
this.minAdvertiserPrice = minAdvertiserPrice;
}
public GoogleProduct minHandlingTime(Long minHandlingTime) {
this.minHandlingTime = minHandlingTime;
return this;
}
/**
* Get minHandlingTime
* @return minHandlingTime
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public Long getMinHandlingTime() {
return minHandlingTime;
}
public void setMinHandlingTime(Long minHandlingTime) {
this.minHandlingTime = minHandlingTime;
}
public GoogleProduct mobileLing(String mobileLing) {
this.mobileLing = mobileLing;
return this;
}
/**
* Get mobileLing
* @return mobileLing
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getMobileLing() {
return mobileLing;
}
public void setMobileLing(String mobileLing) {
this.mobileLing = mobileLing;
}
public GoogleProduct modelNumber(String modelNumber) {
this.modelNumber = modelNumber;
return this;
}
/**
* Get modelNumber
* @return modelNumber
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getModelNumber() {
return modelNumber;
}
public void setModelNumber(String modelNumber) {
this.modelNumber = modelNumber;
}
public GoogleProduct mpn(String mpn) {
this.mpn = mpn;
return this;
}
/**
* Get mpn
* @return mpn
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getMpn() {
return mpn;
}
public void setMpn(String mpn) {
this.mpn = mpn;
}
public GoogleProduct multipack(Integer multipack) {
this.multipack = multipack;
return this;
}
/**
* Get multipack
* @return multipack
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public Integer getMultipack() {
return multipack;
}
public void setMultipack(Integer multipack) {
this.multipack = multipack;
}
public GoogleProduct numberOfReviews(Integer numberOfReviews) {
this.numberOfReviews = numberOfReviews;
return this;
}
/**
* Get numberOfReviews
* @return numberOfReviews
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public Integer getNumberOfReviews() {
return numberOfReviews;
}
public void setNumberOfReviews(Integer numberOfReviews) {
this.numberOfReviews = numberOfReviews;
}
public GoogleProduct pattern(String pattern) {
this.pattern = pattern;
return this;
}
/**
* Get pattern
* @return pattern
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getPattern() {
return pattern;
}
public void setPattern(String pattern) {
this.pattern = pattern;
}
public GoogleProduct price(Price price) {
this.price = price;
return this;
}
/**
* Get price
* @return price
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public Price getPrice() {
return price;
}
public void setPrice(Price price) {
this.price = price;
}
public GoogleProduct productRating(String productRating) {
this.productRating = productRating;
return this;
}
/**
* Get productRating
* @return productRating
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getProductRating() {
return productRating;
}
public void setProductRating(String productRating) {
this.productRating = productRating;
}
public GoogleProduct productType(String productType) {
this.productType = productType;
return this;
}
/**
* Get productType
* @return productType
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getProductType() {
return productType;
}
public void setProductType(String productType) {
this.productType = productType;
}
public GoogleProduct productTypeKey(String productTypeKey) {
this.productTypeKey = productTypeKey;
return this;
}
/**
* Get productTypeKey
* @return productTypeKey
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getProductTypeKey() {
return productTypeKey;
}
public void setProductTypeKey(String productTypeKey) {
this.productTypeKey = productTypeKey;
}
public GoogleProduct promoText(String promoText) {
this.promoText = promoText;
return this;
}
/**
* Get promoText
* @return promoText
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getPromoText() {
return promoText;
}
public void setPromoText(String promoText) {
this.promoText = promoText;
}
public GoogleProduct promotionId(String promotionId) {
this.promotionId = promotionId;
return this;
}
/**
* Get promotionId
* @return promotionId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getPromotionId() {
return promotionId;
}
public void setPromotionId(String promotionId) {
this.promotionId = promotionId;
}
public GoogleProduct salePrice(Price salePrice) {
this.salePrice = salePrice;
return this;
}
/**
* Get salePrice
* @return salePrice
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public Price getSalePrice() {
return salePrice;
}
public void setSalePrice(Price salePrice) {
this.salePrice = salePrice;
}
public GoogleProduct salePriceEffectiveDate(String salePriceEffectiveDate) {
this.salePriceEffectiveDate = salePriceEffectiveDate;
return this;
}
/**
* Get salePriceEffectiveDate
* @return salePriceEffectiveDate
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getSalePriceEffectiveDate() {
return salePriceEffectiveDate;
}
public void setSalePriceEffectiveDate(String salePriceEffectiveDate) {
this.salePriceEffectiveDate = salePriceEffectiveDate;
}
public GoogleProduct shipping(List shipping) {
this.shipping = shipping;
return this;
}
public GoogleProduct addShippingItem(Shipping shippingItem) {
if (this.shipping == null) {
this.shipping = new ArrayList<>();
}
this.shipping.add(shippingItem);
return this;
}
/**
* Get shipping
* @return shipping
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public List getShipping() {
return shipping;
}
public void setShipping(List shipping) {
this.shipping = shipping;
}
public GoogleProduct shippingHeight(ShippingSize shippingHeight) {
this.shippingHeight = shippingHeight;
return this;
}
/**
* Get shippingHeight
* @return shippingHeight
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public ShippingSize getShippingHeight() {
return shippingHeight;
}
public void setShippingHeight(ShippingSize shippingHeight) {
this.shippingHeight = shippingHeight;
}
public GoogleProduct shippingLabel(String shippingLabel) {
this.shippingLabel = shippingLabel;
return this;
}
/**
* Get shippingLabel
* @return shippingLabel
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getShippingLabel() {
return shippingLabel;
}
public void setShippingLabel(String shippingLabel) {
this.shippingLabel = shippingLabel;
}
public GoogleProduct shippingLength(ShippingSize shippingLength) {
this.shippingLength = shippingLength;
return this;
}
/**
* Get shippingLength
* @return shippingLength
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public ShippingSize getShippingLength() {
return shippingLength;
}
public void setShippingLength(ShippingSize shippingLength) {
this.shippingLength = shippingLength;
}
public GoogleProduct shippingWeight(UnitMeasure shippingWeight) {
this.shippingWeight = shippingWeight;
return this;
}
/**
* Get shippingWeight
* @return shippingWeight
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public UnitMeasure getShippingWeight() {
return shippingWeight;
}
public void setShippingWeight(UnitMeasure shippingWeight) {
this.shippingWeight = shippingWeight;
}
public GoogleProduct shippingWidth(ShippingSize shippingWidth) {
this.shippingWidth = shippingWidth;
return this;
}
/**
* Get shippingWidth
* @return shippingWidth
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public ShippingSize getShippingWidth() {
return shippingWidth;
}
public void setShippingWidth(ShippingSize shippingWidth) {
this.shippingWidth = shippingWidth;
}
public GoogleProduct size(String size) {
this.size = size;
return this;
}
/**
* Get size
* @return size
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getSize() {
return size;
}
public void setSize(String size) {
this.size = size;
}
public GoogleProduct sizeSystem(String sizeSystem) {
this.sizeSystem = sizeSystem;
return this;
}
/**
* Get sizeSystem
* @return sizeSystem
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getSizeSystem() {
return sizeSystem;
}
public void setSizeSystem(String sizeSystem) {
this.sizeSystem = sizeSystem;
}
public GoogleProduct sizeType(String sizeType) {
this.sizeType = sizeType;
return this;
}
/**
* Get sizeType
* @return sizeType
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getSizeType() {
return sizeType;
}
public void setSizeType(String sizeType) {
this.sizeType = sizeType;
}
public GoogleProduct tax(List tax) {
this.tax = tax;
return this;
}
public GoogleProduct addTaxItem(Tax taxItem) {
if (this.tax == null) {
this.tax = new ArrayList<>();
}
this.tax.add(taxItem);
return this;
}
/**
* Get tax
* @return tax
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public List getTax() {
return tax;
}
public void setTax(List tax) {
this.tax = tax;
}
public GoogleProduct title(String title) {
this.title = title;
return this;
}
/**
* Get title
* @return title
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public GoogleProduct unitPricingBaseMeasure(UnitMeasure unitPricingBaseMeasure) {
this.unitPricingBaseMeasure = unitPricingBaseMeasure;
return this;
}
/**
* Get unitPricingBaseMeasure
* @return unitPricingBaseMeasure
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public UnitMeasure getUnitPricingBaseMeasure() {
return unitPricingBaseMeasure;
}
public void setUnitPricingBaseMeasure(UnitMeasure unitPricingBaseMeasure) {
this.unitPricingBaseMeasure = unitPricingBaseMeasure;
}
public GoogleProduct unitPricingMeasure(UnitMeasure unitPricingMeasure) {
this.unitPricingMeasure = unitPricingMeasure;
return this;
}
/**
* Get unitPricingMeasure
* @return unitPricingMeasure
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public UnitMeasure getUnitPricingMeasure() {
return unitPricingMeasure;
}
public void setUnitPricingMeasure(UnitMeasure unitPricingMeasure) {
this.unitPricingMeasure = unitPricingMeasure;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
GoogleProduct googleProduct = (GoogleProduct) o;
return Objects.equals(this.id, googleProduct.id) &&
Objects.equals(this.additionalImageLink, googleProduct.additionalImageLink) &&
Objects.equals(this.adult, googleProduct.adult) &&
Objects.equals(this.adwordRedirect, googleProduct.adwordRedirect) &&
Objects.equals(this.ageGroup, googleProduct.ageGroup) &&
Objects.equals(this.availability, googleProduct.availability) &&
Objects.equals(this.availabilityDate, googleProduct.availabilityDate) &&
Objects.equals(this.brand, googleProduct.brand) &&
Objects.equals(this.color, googleProduct.color) &&
Objects.equals(this.customLabel0, googleProduct.customLabel0) &&
Objects.equals(this.customLabel1, googleProduct.customLabel1) &&
Objects.equals(this.customLabel2, googleProduct.customLabel2) &&
Objects.equals(this.customLabel3, googleProduct.customLabel3) &&
Objects.equals(this.customLabel4, googleProduct.customLabel4) &&
Objects.equals(this.description, googleProduct.description) &&
Objects.equals(this.displayAdsId, googleProduct.displayAdsId) &&
Objects.equals(this.displayAdsLink, googleProduct.displayAdsLink) &&
Objects.equals(this.displayAdsSimilarIds, googleProduct.displayAdsSimilarIds) &&
Objects.equals(this.displayAdsTitle, googleProduct.displayAdsTitle) &&
Objects.equals(this.displayAdsValue, googleProduct.displayAdsValue) &&
Objects.equals(this.energyEfficiencyClass, googleProduct.energyEfficiencyClass) &&
Objects.equals(this.excludedDestination, googleProduct.excludedDestination) &&
Objects.equals(this.expirationDate, googleProduct.expirationDate) &&
Objects.equals(this.filters, googleProduct.filters) &&
Objects.equals(this.gender, googleProduct.gender) &&
Objects.equals(this.googleProductCategory, googleProduct.googleProductCategory) &&
Objects.equals(this.gtin, googleProduct.gtin) &&
Objects.equals(this.identifierExists, googleProduct.identifierExists) &&
Objects.equals(this.imageLink, googleProduct.imageLink) &&
Objects.equals(this.installment, googleProduct.installment) &&
Objects.equals(this.isBundle, googleProduct.isBundle) &&
Objects.equals(this.itemGroupId, googleProduct.itemGroupId) &&
Objects.equals(this.link, googleProduct.link) &&
Objects.equals(this.loyatyPoints, googleProduct.loyatyPoints) &&
Objects.equals(this.material, googleProduct.material) &&
Objects.equals(this.maxHandlingTime, googleProduct.maxHandlingTime) &&
Objects.equals(this.minAdvertiserPrice, googleProduct.minAdvertiserPrice) &&
Objects.equals(this.minHandlingTime, googleProduct.minHandlingTime) &&
Objects.equals(this.mobileLing, googleProduct.mobileLing) &&
Objects.equals(this.modelNumber, googleProduct.modelNumber) &&
Objects.equals(this.mpn, googleProduct.mpn) &&
Objects.equals(this.multipack, googleProduct.multipack) &&
Objects.equals(this.numberOfReviews, googleProduct.numberOfReviews) &&
Objects.equals(this.pattern, googleProduct.pattern) &&
Objects.equals(this.price, googleProduct.price) &&
Objects.equals(this.productRating, googleProduct.productRating) &&
Objects.equals(this.productType, googleProduct.productType) &&
Objects.equals(this.productTypeKey, googleProduct.productTypeKey) &&
Objects.equals(this.promoText, googleProduct.promoText) &&
Objects.equals(this.promotionId, googleProduct.promotionId) &&
Objects.equals(this.salePrice, googleProduct.salePrice) &&
Objects.equals(this.salePriceEffectiveDate, googleProduct.salePriceEffectiveDate) &&
Objects.equals(this.shipping, googleProduct.shipping) &&
Objects.equals(this.shippingHeight, googleProduct.shippingHeight) &&
Objects.equals(this.shippingLabel, googleProduct.shippingLabel) &&
Objects.equals(this.shippingLength, googleProduct.shippingLength) &&
Objects.equals(this.shippingWeight, googleProduct.shippingWeight) &&
Objects.equals(this.shippingWidth, googleProduct.shippingWidth) &&
Objects.equals(this.size, googleProduct.size) &&
Objects.equals(this.sizeSystem, googleProduct.sizeSystem) &&
Objects.equals(this.sizeType, googleProduct.sizeType) &&
Objects.equals(this.tax, googleProduct.tax) &&
Objects.equals(this.title, googleProduct.title) &&
Objects.equals(this.unitPricingBaseMeasure, googleProduct.unitPricingBaseMeasure) &&
Objects.equals(this.unitPricingMeasure, googleProduct.unitPricingMeasure);
}
@Override
public int hashCode() {
return Objects.hash(id, additionalImageLink, adult, adwordRedirect, ageGroup, availability, availabilityDate, brand, color, customLabel0, customLabel1, customLabel2, customLabel3, customLabel4, description, displayAdsId, displayAdsLink, displayAdsSimilarIds, displayAdsTitle, displayAdsValue, energyEfficiencyClass, excludedDestination, expirationDate, filters, gender, googleProductCategory, gtin, identifierExists, imageLink, installment, isBundle, itemGroupId, link, loyatyPoints, material, maxHandlingTime, minAdvertiserPrice, minHandlingTime, mobileLing, modelNumber, mpn, multipack, numberOfReviews, pattern, price, productRating, productType, productTypeKey, promoText, promotionId, salePrice, salePriceEffectiveDate, shipping, shippingHeight, shippingLabel, shippingLength, shippingWeight, shippingWidth, size, sizeSystem, sizeType, tax, title, unitPricingBaseMeasure, unitPricingMeasure);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class GoogleProduct {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" additionalImageLink: ").append(toIndentedString(additionalImageLink)).append("\n");
sb.append(" adult: ").append(toIndentedString(adult)).append("\n");
sb.append(" adwordRedirect: ").append(toIndentedString(adwordRedirect)).append("\n");
sb.append(" ageGroup: ").append(toIndentedString(ageGroup)).append("\n");
sb.append(" availability: ").append(toIndentedString(availability)).append("\n");
sb.append(" availabilityDate: ").append(toIndentedString(availabilityDate)).append("\n");
sb.append(" brand: ").append(toIndentedString(brand)).append("\n");
sb.append(" color: ").append(toIndentedString(color)).append("\n");
sb.append(" customLabel0: ").append(toIndentedString(customLabel0)).append("\n");
sb.append(" customLabel1: ").append(toIndentedString(customLabel1)).append("\n");
sb.append(" customLabel2: ").append(toIndentedString(customLabel2)).append("\n");
sb.append(" customLabel3: ").append(toIndentedString(customLabel3)).append("\n");
sb.append(" customLabel4: ").append(toIndentedString(customLabel4)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" displayAdsId: ").append(toIndentedString(displayAdsId)).append("\n");
sb.append(" displayAdsLink: ").append(toIndentedString(displayAdsLink)).append("\n");
sb.append(" displayAdsSimilarIds: ").append(toIndentedString(displayAdsSimilarIds)).append("\n");
sb.append(" displayAdsTitle: ").append(toIndentedString(displayAdsTitle)).append("\n");
sb.append(" displayAdsValue: ").append(toIndentedString(displayAdsValue)).append("\n");
sb.append(" energyEfficiencyClass: ").append(toIndentedString(energyEfficiencyClass)).append("\n");
sb.append(" excludedDestination: ").append(toIndentedString(excludedDestination)).append("\n");
sb.append(" expirationDate: ").append(toIndentedString(expirationDate)).append("\n");
sb.append(" filters: ").append(toIndentedString(filters)).append("\n");
sb.append(" gender: ").append(toIndentedString(gender)).append("\n");
sb.append(" googleProductCategory: ").append(toIndentedString(googleProductCategory)).append("\n");
sb.append(" gtin: ").append(toIndentedString(gtin)).append("\n");
sb.append(" identifierExists: ").append(toIndentedString(identifierExists)).append("\n");
sb.append(" imageLink: ").append(toIndentedString(imageLink)).append("\n");
sb.append(" installment: ").append(toIndentedString(installment)).append("\n");
sb.append(" isBundle: ").append(toIndentedString(isBundle)).append("\n");
sb.append(" itemGroupId: ").append(toIndentedString(itemGroupId)).append("\n");
sb.append(" link: ").append(toIndentedString(link)).append("\n");
sb.append(" loyatyPoints: ").append(toIndentedString(loyatyPoints)).append("\n");
sb.append(" material: ").append(toIndentedString(material)).append("\n");
sb.append(" maxHandlingTime: ").append(toIndentedString(maxHandlingTime)).append("\n");
sb.append(" minAdvertiserPrice: ").append(toIndentedString(minAdvertiserPrice)).append("\n");
sb.append(" minHandlingTime: ").append(toIndentedString(minHandlingTime)).append("\n");
sb.append(" mobileLing: ").append(toIndentedString(mobileLing)).append("\n");
sb.append(" modelNumber: ").append(toIndentedString(modelNumber)).append("\n");
sb.append(" mpn: ").append(toIndentedString(mpn)).append("\n");
sb.append(" multipack: ").append(toIndentedString(multipack)).append("\n");
sb.append(" numberOfReviews: ").append(toIndentedString(numberOfReviews)).append("\n");
sb.append(" pattern: ").append(toIndentedString(pattern)).append("\n");
sb.append(" price: ").append(toIndentedString(price)).append("\n");
sb.append(" productRating: ").append(toIndentedString(productRating)).append("\n");
sb.append(" productType: ").append(toIndentedString(productType)).append("\n");
sb.append(" productTypeKey: ").append(toIndentedString(productTypeKey)).append("\n");
sb.append(" promoText: ").append(toIndentedString(promoText)).append("\n");
sb.append(" promotionId: ").append(toIndentedString(promotionId)).append("\n");
sb.append(" salePrice: ").append(toIndentedString(salePrice)).append("\n");
sb.append(" salePriceEffectiveDate: ").append(toIndentedString(salePriceEffectiveDate)).append("\n");
sb.append(" shipping: ").append(toIndentedString(shipping)).append("\n");
sb.append(" shippingHeight: ").append(toIndentedString(shippingHeight)).append("\n");
sb.append(" shippingLabel: ").append(toIndentedString(shippingLabel)).append("\n");
sb.append(" shippingLength: ").append(toIndentedString(shippingLength)).append("\n");
sb.append(" shippingWeight: ").append(toIndentedString(shippingWeight)).append("\n");
sb.append(" shippingWidth: ").append(toIndentedString(shippingWidth)).append("\n");
sb.append(" size: ").append(toIndentedString(size)).append("\n");
sb.append(" sizeSystem: ").append(toIndentedString(sizeSystem)).append("\n");
sb.append(" sizeType: ").append(toIndentedString(sizeType)).append("\n");
sb.append(" tax: ").append(toIndentedString(tax)).append("\n");
sb.append(" title: ").append(toIndentedString(title)).append("\n");
sb.append(" unitPricingBaseMeasure: ").append(toIndentedString(unitPricingBaseMeasure)).append("\n");
sb.append(" unitPricingMeasure: ").append(toIndentedString(unitPricingMeasure)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy