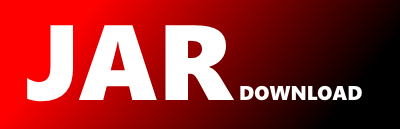
com.cryptoregistry.tweet.pepper.Block Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tweetpepper Show documentation
Show all versions of tweetpepper Show documentation
Built on the nucleus of TweetNaCl, TweetPepper provides contemporary key formats, key protection using SCrypt/SecretBox, digital signature support scheme featuring CubeHash, key encapsulation using Salsa20, and other useful features you probably want anyway.
The newest version!
/*
Copyright 2016, David R. Smith, All Rights Reserved
This file is part of TweetPepper.
TweetPepper is free software: you can redistribute it and/or modify
it under the terms of the GNU General Public License as published by
the Free Software Foundation, either version 3 of the License, or
(at your option) any later version.
TweetPepper is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU General Public License for more details.
You should have received a copy of the GNU General Public License
along with TweetPepper. If not, see .
*/
package com.cryptoregistry.tweet.pepper;
import java.util.Base64;
import java.util.LinkedHashMap;
import java.util.Map;
import java.util.UUID;
import com.cryptoregistry.tweet.url.BijectiveEncoder;
/**
* A Block is a linked hash map with a unique identifier and a type:
*
*
* - C = Contact data
* - D = arbitrary string keys and values, Data
* - E = encrypted data
* - P = key, for-publication part only
* - U = key contents, unsecured
* - X = key contents, secured/encrypted
* - S = Signature block
* - T = Transaction block
*
*
*
* @author Dave
*
*/
public class Block extends LinkedHashMap {
private static final long serialVersionUID = 1L;
public String name; // canonical name - should be globally unique such as a UUID
public Block(BlockType type) {
super();
BijectiveEncoder bj = new BijectiveEncoder();
name = bj.encode(UUID.randomUUID())+"-"+type.toString();
}
public Block(String base, BlockType type) {
super();
name = base+"-"+type.toString();
}
/**
* Used with the full Base-BlockType value
*
* @param dName
*/
public Block(String dName) {
super();
name = dName;
}
public BlockType getBlockType() {
final char val = name.charAt(name.length()-1);
switch(val){
case 'C': return BlockType.C;
case 'D': return BlockType.D;
case 'E': return BlockType.E;
case 'P': return BlockType.P;
case 'U': return BlockType.U;
case 'X': return BlockType.X;
case 'S': return BlockType.S;
case 'T': return BlockType.T;
default: throw new RuntimeException("Unknown category: "+val);
}
}
public boolean isP(){
return getBlockType()==BlockType.P;
}
public boolean isU(){
return getBlockType()==BlockType.U;
}
public boolean isX(){
return getBlockType()==BlockType.X;
}
public boolean isSigner(){
return this.containsKey("KeyUsage") && this.get("KeyUsage").equals("Signing");
}
public boolean isBoxing(){
return this.containsKey("KeyUsage") && this.get("KeyUsage").equals("Boxing");
}
public boolean isSecretBox(){
return this.containsKey("KeyUsage") && this.get("KeyUsage").equals("SecretBox");
}
public String toString() {
return name;
}
public String baseName() {
return name.substring(0, name.length()-2);
}
/**
* Return a traditional UUID
*
* @return
*/
public UUID baseToUUID() {
BijectiveEncoder bj = new BijectiveEncoder();
return bj.decode(baseName());
}
/**
* loads our contents into a map which is used for signature validation
*
* @param scopeMap
*/
public void loadToSignatureScope(Map scopeMap){
for(String key: keySet()) {
String value = get(key);
scopeMap.put(name+":"+key, value);
}
}
public byte [] getBytesFromBase64urlString(String key){
if(!this.containsKey(key))throw new RuntimeException("key not present: "+key);
return Base64.getUrlDecoder().decode(get(key));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy