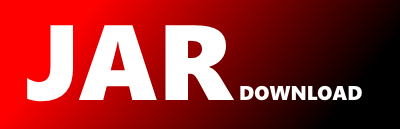
com.ctaiot.bean.FolderPlugCloudFileResult Maven / Gradle / Ivy
/**
*
* 作 者:黄晓明
* 创建日期:2022-4-25
*
*/
package com.ctaiot.bean;
import java.io.Serializable;
import java.util.Date;
public class FolderPlugCloudFileResult implements Serializable {
private Long id;
private String sequenceCode;
private Long companyId;
private String appId;
private String userId;
private String fileName;
private String filePath;
private String img;
private Integer width;
private Integer height;
private String ossFilePath;
private String ossFileUrl;
private Integer status;
private String ext;
private Double size;
private Integer type;
private String version;
private String hashValue;
private Integer fileType;
private Long folderId;
private Integer sequence;
private String keyWords;
private String content;
private Integer dataType;
private String byteObject;
private String cloudDbIds;
private String cloudPicIds;
private String cloudFontIds;
private String cloudPicUrls;
private Integer docType;
private String docNo;
private Integer rfid;
private Date createTime;
private Date addTime;
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getSequenceCode() {
return sequenceCode;
}
public void setSequenceCode(String sequenceCode) {
this.sequenceCode = sequenceCode;
}
public Long getCompanyId() {
return companyId;
}
public void setCompanyId(Long companyId) {
this.companyId = companyId;
}
public String getAppId() {
return appId;
}
public void setAppId(String appId) {
this.appId = appId;
}
public String getUserId() {
return userId;
}
public void setUserId(String userId) {
this.userId = userId;
}
public String getFileName() {
return fileName;
}
public void setFileName(String fileName) {
this.fileName = fileName;
}
public String getFilePath() {
return filePath;
}
public void setFilePath(String filePath) {
this.filePath = filePath;
}
public String getImg() {
return img;
}
public void setImg(String img) {
this.img = img;
}
public Integer getWidth() {
return width;
}
public void setWidth(Integer width) {
this.width = width;
}
public Integer getHeight() {
return height;
}
public void setHeight(Integer height) {
this.height = height;
}
public String getOssFilePath() {
return ossFilePath;
}
public void setOssFilePath(String ossFilePath) {
this.ossFilePath = ossFilePath;
}
public String getOssFileUrl() {
return ossFileUrl;
}
public void setOssFileUrl(String ossFileUrl) {
this.ossFileUrl = ossFileUrl;
}
public Integer getStatus() {
return status;
}
public void setStatus(Integer status) {
this.status = status;
}
public String getExt() {
return ext;
}
public void setExt(String ext) {
this.ext = ext;
}
public Double getSize() {
return size;
}
public void setSize(Double size) {
this.size = size;
}
public Integer getType() {
return type;
}
public void setType(Integer type) {
this.type = type;
}
public String getVersion() {
return version;
}
public void setVersion(String version) {
this.version = version;
}
public String getHashValue() {
return hashValue;
}
public void setHashValue(String hashValue) {
this.hashValue = hashValue;
}
public Integer getFileType() {
return fileType;
}
public void setFileType(Integer fileType) {
this.fileType = fileType;
}
public Long getFolderId() {
return folderId;
}
public void setFolderId(Long folderId) {
this.folderId = folderId;
}
public Integer getSequence() {
return sequence;
}
public void setSequence(Integer sequence) {
this.sequence = sequence;
}
public String getKeyWords() {
return keyWords;
}
public void setKeyWords(String keyWords) {
this.keyWords = keyWords;
}
public String getContent() {
return content;
}
public void setContent(String content) {
this.content = content;
}
public Integer getDataType() {
return dataType;
}
public void setDataType(Integer dataType) {
this.dataType = dataType;
}
public String getByteObject() {
return byteObject;
}
public void setByteObject(String byteObject) {
this.byteObject = byteObject;
}
public String getCloudDbIds() {
return cloudDbIds;
}
public void setCloudDbIds(String cloudDbIds) {
this.cloudDbIds = cloudDbIds;
}
public String getCloudPicIds() {
return cloudPicIds;
}
public void setCloudPicIds(String cloudPicIds) {
this.cloudPicIds = cloudPicIds;
}
public String getCloudFontIds() {
return cloudFontIds;
}
public void setCloudFontIds(String cloudFontIds) {
this.cloudFontIds = cloudFontIds;
}
public String getCloudPicUrls() {
return cloudPicUrls;
}
public void setCloudPicUrls(String cloudPicUrls) {
this.cloudPicUrls = cloudPicUrls;
}
public Integer getDocType() {
return docType;
}
public void setDocType(Integer docType) {
this.docType = docType;
}
public String getDocNo() {
return docNo;
}
public void setDocNo(String docNo) {
this.docNo = docNo;
}
public Integer getRfid() {
return rfid;
}
public void setRfid(Integer rfid) {
this.rfid = rfid;
}
public Date getCreateTime() {
return createTime;
}
public void setCreateTime(Date createTime) {
this.createTime = createTime;
}
public Date getAddTime() {
return addTime;
}
public void setAddTime(Date addTime) {
this.addTime = addTime;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy