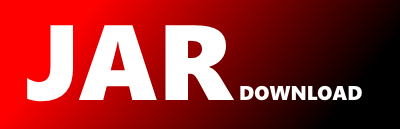
com.ctaiot.print.PrintClient Maven / Gradle / Ivy
package com.ctaiot.print;
import com.ctaiot.CloudPrintConfig;
import com.ctaiot.bean.PrintPayLoad;
import com.ctaiot.bean.PrintResult;
import com.ctaiot.connection.HttpProxy;
import com.ctaiot.connection.IHttpClient;
import com.ctaiot.connection.NativeHttpClient;
import com.ctaiot.resp.APIConnectionException;
import com.ctaiot.resp.APIRequestException;
import com.ctaiot.resp.BaseResult;
import com.ctaiot.resp.ResponseWrapper;
import com.ctaiot.utils.FileUtil;
import com.ctaiot.utils.Md5Util;
import com.google.gson.JsonParser;
import io.netty.util.internal.StringUtil;
import java.io.IOException;
import java.util.*;
public class PrintClient {
private IHttpClient httpClient;
private String appKey;
private String appSecret;
private String hostUrl;
private String sdkTokenUrl;
private String brandListUrl;
private String brandSaveUrl;
private String folderLabelListUrl;
private String clientIdInfoUrl;
private String sendApiPrintUrl;
private String sendPdfPrintUrl;
private String sendPicPrintUrl;
private String printUrl;
private String listPrintTask;
private String operatePrintTask;
private String preUploadResource;
private String sendPicResourcePrint;
private String sendDataPrint;
private String dataPrintView;
private JsonParser _jsonParser = new JsonParser();
private PrintClient() {
}
public PrintClient(String appKey, String appSecret) {
this(null, appKey, appSecret, null, CloudPrintConfig.getInstance());
}
public PrintClient(String hostUrl, String appKey, String appSecret) {
this(hostUrl, appKey, appSecret, null, CloudPrintConfig.getInstance());
}
public PrintClient(String hostUrl, String appKey, String appSecret, CloudPrintConfig clientConfig) {
this(hostUrl, appKey, appSecret, null, clientConfig);
}
public PrintClient(String hostUrl, String appKey, String appSecret, HttpProxy proxy, CloudPrintConfig clientConfig) {
this.appKey = appKey;
this.appSecret = appSecret;
if (StringUtil.isNullOrEmpty(hostUrl)) {
this.hostUrl = (String) clientConfig.get(CloudPrintConfig.HOST_NAME);
} else {
this.hostUrl = hostUrl;
}
this.hostUrl = this.hostUrl + (String) clientConfig.get(CloudPrintConfig.HOST_NAME_PATH);
this.sdkTokenUrl = (String) clientConfig.get(CloudPrintConfig.SDK_TOKEN);
this.brandListUrl = (String) clientConfig.get(CloudPrintConfig.BRAND_LIST);
this.brandSaveUrl = (String) clientConfig.get(CloudPrintConfig.BRAND_SAVE);
this.folderLabelListUrl = (String) clientConfig.get(CloudPrintConfig.FOLDER_LABEL_LIST);
this.clientIdInfoUrl = (String) clientConfig.get(CloudPrintConfig.CLIENT_ID_INFO);
this.sendApiPrintUrl = (String) clientConfig.get(CloudPrintConfig.SEND_API_PRINT);
this.sendPdfPrintUrl = (String) clientConfig.get(CloudPrintConfig.SEND_PDF_PRINT);
this.sendPicPrintUrl = (String) clientConfig.get(CloudPrintConfig.SEND_PIC_PRINT);
this.printUrl = (String) clientConfig.get(CloudPrintConfig.PRINT_URL);
this.listPrintTask = (String) clientConfig.get(CloudPrintConfig.LIST_PRINT_TASK);
this.operatePrintTask = (String) clientConfig.get(CloudPrintConfig.OPERATE_PRINT_TASK);
this.preUploadResource = (String) clientConfig.get(CloudPrintConfig.PRE_UPLOAD_RESOURCE);
this.sendPicResourcePrint = (String) clientConfig.get(CloudPrintConfig.SEND_PIC_RESOURCE_PRINT);
this.sendDataPrint = (String) clientConfig.get(CloudPrintConfig.SEND_DATA_PRINT);
this.dataPrintView = (String) clientConfig.get(CloudPrintConfig.DATA_PRINT_VIEW);
this.httpClient = new NativeHttpClient(null, clientConfig);
}
public PrintClient(String appKey, String appSecret, HttpProxy proxy, CloudPrintConfig clientConfig) {
this.appKey = appKey;
this.appSecret = appSecret;
this.hostUrl = (String) clientConfig.get(CloudPrintConfig.HOST_NAME) + (String) clientConfig.get(CloudPrintConfig.HOST_NAME_PATH);
this.sdkTokenUrl = (String) clientConfig.get(CloudPrintConfig.SDK_TOKEN);
this.brandListUrl = (String) clientConfig.get(CloudPrintConfig.BRAND_LIST);
this.brandSaveUrl = (String) clientConfig.get(CloudPrintConfig.BRAND_SAVE);
this.folderLabelListUrl = (String) clientConfig.get(CloudPrintConfig.FOLDER_LABEL_LIST);
this.clientIdInfoUrl = (String) clientConfig.get(CloudPrintConfig.CLIENT_ID_INFO);
this.sendApiPrintUrl = (String) clientConfig.get(CloudPrintConfig.SEND_API_PRINT);
this.sendPdfPrintUrl = (String) clientConfig.get(CloudPrintConfig.SEND_PDF_PRINT);
this.sendPicPrintUrl = (String) clientConfig.get(CloudPrintConfig.SEND_PIC_PRINT);
this.printUrl = (String) clientConfig.get(CloudPrintConfig.PRINT_URL);
this.listPrintTask = (String) clientConfig.get(CloudPrintConfig.LIST_PRINT_TASK);
this.operatePrintTask = (String) clientConfig.get(CloudPrintConfig.OPERATE_PRINT_TASK);
this.preUploadResource = (String) clientConfig.get(CloudPrintConfig.PRE_UPLOAD_RESOURCE);
this.sendPicResourcePrint = (String) clientConfig.get(CloudPrintConfig.SEND_PIC_RESOURCE_PRINT);
this.sendDataPrint = (String) clientConfig.get(CloudPrintConfig.SEND_DATA_PRINT);
this.httpClient = new NativeHttpClient(null, clientConfig);
}
/**
* get authorization token
*
* @throws APIConnectionException
* @throws APIRequestException
*/
public PrintResult getSdkToken(String userId) throws APIConnectionException, APIRequestException {
Map map = new HashMap<>();
map.put("appKey", appKey);
if (Objects.equals(appKey, "demo-app-key")) {
String str = String.valueOf(System.nanoTime());
int len = str.length();
FileUtil fileUtil = new FileUtil();
try {
userId = fileUtil.getUserId(userId + "_" + str.substring(len - 5, len));
} catch (IOException e) {
userId = userId + "_" + str.substring(len - 5, len);
}
}
map.put("userId", userId);
map.put("timestamp", Calendar.getInstance().getTimeInMillis());
TreeMap treeMap = new TreeMap<>();
treeMap.put("sequenceCode", "");
treeMap.put("companyId", "");
treeMap.put("appKey", map.get("appKey"));
treeMap.put("userId", userId);
treeMap.put("timestamp", map.get("timestamp"));
String sign = Md5Util.sign(Md5Util.formatSignContent(treeMap), appSecret);
map.put("sign", sign);
PrintPayLoad payLoad = new PrintPayLoad();
payLoad.setParams(map);
ResponseWrapper response = httpClient.sendPost(this.hostUrl + this.sdkTokenUrl, payLoad.getParams()
, payLoad.getHeaders());
return BaseResult.fromResponse(response, PrintResult.class);
}
/**
* get printer brand list
*
* payLoad
*
* @throws APIConnectionException
* @throws APIRequestException
*/
public PrintResult getBrandList(PrintPayLoad payLoad) throws APIConnectionException, APIRequestException {
checkPrintPayLoad(payLoad);
ResponseWrapper response = httpClient.sendGet(this.hostUrl + this.brandListUrl + "?" + payLoad.toParams()
, payLoad.getHeaders());
return BaseResult.fromResponse(response, PrintResult.class);
}
/**
* save printer brand
*
* payLoad
*
* @throws APIConnectionException
* @throws APIRequestException
*/
public PrintResult saveBrand(PrintPayLoad payLoad) throws APIConnectionException, APIRequestException {
checkPrintPayLoad(payLoad);
ResponseWrapper response = httpClient.sendPost(this.hostUrl + this.brandSaveUrl,
payLoad.getParams(), payLoad.getHeaders());
return BaseResult.fromResponse(response, PrintResult.class);
}
/**
* get folder label list
*
* payLoad
*
* @throws APIConnectionException
* @throws APIRequestException
*/
public PrintResult getFolderLabelList(PrintPayLoad payLoad) throws APIConnectionException, APIRequestException {
checkPrintPayLoad(payLoad);
ResponseWrapper response = httpClient.sendPost(this.hostUrl + this.folderLabelListUrl,
payLoad.getParams(), payLoad.getHeaders());
return BaseResult.fromResponse(response, PrintResult.class);
}
/**
* get client id
*
* payLoad
*
* @throws APIConnectionException
* @throws APIRequestException
*/
@Deprecated
public PrintResult getClientIdInfo(PrintPayLoad payLoad) throws APIConnectionException, APIRequestException {
checkPrintPayLoad(payLoad);
ResponseWrapper response = httpClient.sendPost(this.hostUrl + this.clientIdInfoUrl,
payLoad.getParams(), payLoad.getHeaders());
return BaseResult.fromResponse(response, PrintResult.class);
}
/**
* send api print
*
* payLoad
*
* @throws APIConnectionException
* @throws APIRequestException
*/
public PrintResult sendApiPrint(PrintPayLoad payLoad) throws APIConnectionException, APIRequestException {
checkPrintPayLoad(payLoad);
ResponseWrapper response = httpClient.sendPost(this.hostUrl + this.sendApiPrintUrl,
payLoad.getParams(), payLoad.getHeaders());
return BaseResult.fromResponse(response, PrintResult.class);
}
/**
* send pdf print
*
* payLoad
*
* @throws APIConnectionException
* @throws APIRequestException
*/
public PrintResult sendPdfPrint(PrintPayLoad payLoad) throws APIConnectionException, APIRequestException {
checkPrintPayLoad(payLoad);
ResponseWrapper response = httpClient.sendPost(this.hostUrl + this.sendPdfPrintUrl,
payLoad.getParams(), payLoad.getHeaders());
return BaseResult.fromResponse(response, PrintResult.class);
}
/**
* send pic print
*
* payLoad
*
* @throws APIConnectionException
* @throws APIRequestException
*/
public PrintResult sendPicPrint(PrintPayLoad payLoad) throws APIConnectionException, APIRequestException {
checkPrintPayLoad(payLoad);
ResponseWrapper response = httpClient.sendPost(this.hostUrl + this.sendPicPrintUrl,
payLoad.getParams(), payLoad.getHeaders());
return BaseResult.fromResponse(response, PrintResult.class);
}
/**
* get print url drives
*
* payLoad
*
* @throws APIConnectionException
* @throws APIRequestException
*/
public PrintResult printUrl(PrintPayLoad payLoad) throws APIConnectionException, APIRequestException {
ResponseWrapper response = httpClient.sendPost(this.hostUrl + this.printUrl,
payLoad.getParams(), payLoad.getHeaders());
return BaseResult.fromResponse(response, PrintResult.class);
}
/**
* get list print task
* payLoad
*
* @throws APIConnectionException
* @throws APIRequestException
*/
public PrintResult listPrintTask(PrintPayLoad payLoad) throws APIConnectionException, APIRequestException {
ResponseWrapper response = httpClient.sendPost(this.hostUrl + this.listPrintTask,
payLoad.getParams(), payLoad.getHeaders());
return BaseResult.fromResponse(response, PrintResult.class);
}
/**
* get operate print task
* payLoad
*
* @throws APIConnectionException
* @throws APIRequestException
*/
public PrintResult operatePrintTask(PrintPayLoad payLoad) throws APIConnectionException, APIRequestException {
ResponseWrapper response = httpClient.sendPost(this.hostUrl + this.operatePrintTask,
payLoad.getParams(), payLoad.getHeaders());
return BaseResult.fromResponse(response, PrintResult.class);
}
/**
* resource preheating
*
* @param payLoad
* @return
* @throws APIConnectionException
* @throws APIRequestException
*/
public PrintResult preUploadResource(PrintPayLoad payLoad) throws APIConnectionException, APIRequestException {
ResponseWrapper response = httpClient.sendPost(this.hostUrl + this.preUploadResource,
payLoad.getParams(), payLoad.getHeaders());
return BaseResult.fromResponse(response, PrintResult.class);
}
/**
* send data print
*
* @param payLoad
* @return
* @throws APIConnectionException
* @throws APIRequestException
*/
public PrintResult sendDataPrint(PrintPayLoad payLoad) throws APIConnectionException, APIRequestException {
ResponseWrapper response = httpClient.sendPost(this.hostUrl + this.sendDataPrint,
payLoad.getParams(), payLoad.getHeaders());
return BaseResult.fromResponse(response, PrintResult.class);
}
/**
* send pic print
*
* @param payLoad
* @return
* @throws APIConnectionException
* @throws APIRequestException
*/
public PrintResult sendPicResourcePrint(PrintPayLoad payLoad) throws APIConnectionException, APIRequestException {
ResponseWrapper response = httpClient.sendPost(this.hostUrl + this.sendPicResourcePrint,
payLoad.getParams(), payLoad.getHeaders());
return BaseResult.fromResponse(response, PrintResult.class);
}
/**
* data print view
*
* @param payLoad
* @return
* @throws APIConnectionException
* @throws APIRequestException
*/
public PrintResult dataPrintView(PrintPayLoad payLoad) throws APIConnectionException, APIRequestException {
ResponseWrapper response = httpClient.sendPost(this.hostUrl + this.dataPrintView,
payLoad.getParams(), payLoad.getHeaders());
return BaseResult.fromResponse(response, PrintResult.class);
}
private void checkPrintPayLoad(PrintPayLoad payLoad) {
if (payLoad == null) {
throw new IllegalArgumentException("printPayLoad should not be null");
}
if (payLoad.getHeaders() == null) {
throw new IllegalArgumentException("headers should not be null");
}
}
}