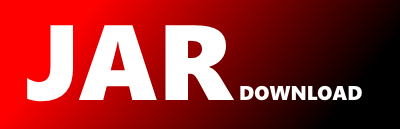
commonMain.com.ctrip.sqllin.dsl.sql.clause.OrderByClause.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sqllin-dsl Show documentation
Show all versions of sqllin-dsl Show documentation
SQL DSL APIs for SQLite on Kotlin Multiplatform
/*
* Copyright (C) 2022 Ctrip.com.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.ctrip.sqllin.dsl.sql.clause
import com.ctrip.sqllin.dsl.sql.statement.*
/**
* SQL 'order by' clause by select statement
* @author yaqiao
*/
public sealed interface OrderByClause : SelectClause
internal class CompleteOrderByClause(private val column2WayMap: Map) : OrderByClause {
override val clauseStr: String
get() {
require(column2WayMap.isNotEmpty()) { "Please provider at least one 'BaseClauseElement' -> 'OrderByWay' entry for 'ORDER BY' clause!!!" }
return buildString {
append(" ORDER BY ")
val iterator = column2WayMap.entries.iterator()
do {
val (element, way) = iterator.next()
append(element.valueName)
append(' ')
append(way.str)
val hasNext = iterator.hasNext()
if (hasNext)
append(',')
} while (hasNext)
}
}
}
public enum class OrderByWay(internal val str: String) {
ASC("ASC"),
DESC("DESC")
}
public fun ORDER_BY(vararg column2Ways: Pair): OrderByClause =
CompleteOrderByClause(mapOf(*column2Ways))
public inline infix fun WhereSelectStatement.ORDER_BY(column2Way: Pair): OrderBySelectStatement =
ORDER_BY(mapOf(column2Way))
public infix fun WhereSelectStatement.ORDER_BY(column2WayMap: Map): OrderBySelectStatement =
appendToOrderBy(CompleteOrderByClause(column2WayMap)).also {
container changeLastStatement it
}
public inline infix fun HavingSelectStatement.ORDER_BY(column2Way: Pair): OrderBySelectStatement =
ORDER_BY(mapOf(column2Way))
public infix fun HavingSelectStatement.ORDER_BY(column2WayMap: Map): OrderBySelectStatement =
appendToOrderBy(CompleteOrderByClause(column2WayMap)).also {
container changeLastStatement it
}
public inline infix fun GroupBySelectStatement.ORDER_BY(column2Way: Pair): OrderBySelectStatement =
ORDER_BY(mapOf(column2Way))
public infix fun GroupBySelectStatement.ORDER_BY(column2WayMap: Map): OrderBySelectStatement =
appendToOrderBy(CompleteOrderByClause(column2WayMap)).also {
container changeLastStatement it
}
public inline infix fun JoinSelectStatement.ORDER_BY(column2Way: Pair): OrderBySelectStatement =
ORDER_BY(mapOf(column2Way))
public infix fun JoinSelectStatement.ORDER_BY(column2WayMap: Map): OrderBySelectStatement =
appendToOrderBy(CompleteOrderByClause(column2WayMap)).also {
container changeLastStatement it
}
internal class SimpleOrderByClause(private val columns: Iterable) : OrderByClause {
override val clauseStr: String
get() {
val iterator = columns.iterator()
require(iterator.hasNext()) { "Please provider at least one 'BaseClauseElement' for 'ORDER BY' clause!!!" }
return buildString {
append(" ORDER BY ")
append(iterator.next().valueName)
while (iterator.hasNext()) {
append(',')
append(iterator.next().valueName)
}
}
}
}
public fun ORDER_BY(vararg elements: ClauseElement): OrderByClause =
SimpleOrderByClause(elements.toList())
public inline infix fun WhereSelectStatement.ORDER_BY(column: ClauseElement): OrderBySelectStatement =
ORDER_BY(listOf(column))
public infix fun WhereSelectStatement.ORDER_BY(columns: Iterable): OrderBySelectStatement =
appendToOrderBy(SimpleOrderByClause(columns)).also {
container changeLastStatement it
}
public inline infix fun HavingSelectStatement.ORDER_BY(column: ClauseElement): OrderBySelectStatement =
ORDER_BY(listOf(column))
public infix fun HavingSelectStatement.ORDER_BY(columns: Iterable): OrderBySelectStatement =
appendToOrderBy(SimpleOrderByClause(columns)).also {
container changeLastStatement it
}
public inline infix fun GroupBySelectStatement.ORDER_BY(column: ClauseElement): OrderBySelectStatement =
ORDER_BY(listOf(column))
public infix fun GroupBySelectStatement.ORDER_BY(columns: Iterable): OrderBySelectStatement =
appendToOrderBy(SimpleOrderByClause(columns)).also {
container changeLastStatement it
}
public inline infix fun JoinSelectStatement.ORDER_BY(column: ClauseElement): OrderBySelectStatement =
ORDER_BY(listOf(column))
public infix fun JoinSelectStatement.ORDER_BY(columns: Iterable): OrderBySelectStatement =
appendToOrderBy(SimpleOrderByClause(columns)).also {
container changeLastStatement it
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy