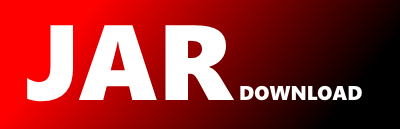
commonMain.com.ctrip.sqllin.dsl.sql.operation.Select.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sqllin-dsl Show documentation
Show all versions of sqllin-dsl Show documentation
SQL DSL APIs for SQLite on Kotlin Multiplatform
/*
* Copyright (C) 2022 Ctrip.com.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.ctrip.sqllin.dsl.sql.operation
import com.ctrip.sqllin.driver.DatabaseConnection
import com.ctrip.sqllin.dsl.sql.Table
import com.ctrip.sqllin.dsl.sql.clause.*
import com.ctrip.sqllin.dsl.sql.compiler.appendDBColumnName
import com.ctrip.sqllin.dsl.sql.statement.*
import kotlinx.serialization.DeserializationStrategy
/**
* SQL query
* @author yaqiao
*/
internal object Select : Operation {
override val sqlStr: String
get() = "SELECT "
fun select(
table: Table,
clause: WhereClause,
isDistinct: Boolean,
deserializer: DeserializationStrategy,
connection: DatabaseConnection,
container: StatementContainer,
): WhereSelectStatement =
WhereSelectStatement(buildSQL(table, clause, isDistinct, deserializer), deserializer, connection, container, clause.selectCondition.parameters)
fun select(
table: Table,
clause: OrderByClause,
isDistinct: Boolean,
deserializer: DeserializationStrategy,
connection: DatabaseConnection,
container: StatementContainer,
): OrderBySelectStatement =
OrderBySelectStatement(buildSQL(table, clause, isDistinct, deserializer), deserializer, connection, container, null)
fun select(
table: Table,
clause: LimitClause,
isDistinct: Boolean,
deserializer: DeserializationStrategy,
connection: DatabaseConnection,
container: StatementContainer,
): LimitSelectStatement =
LimitSelectStatement(buildSQL(table, clause, isDistinct, deserializer), deserializer, connection, container, null)
fun select(
table: Table,
clause: GroupByClause,
isDistinct: Boolean,
deserializer: DeserializationStrategy,
connection: DatabaseConnection,
container: StatementContainer,
): GroupBySelectStatement =
GroupBySelectStatement(buildSQL(table, clause, isDistinct, deserializer), deserializer, connection, container, null)
fun select(
table: Table<*>,
clause: NaturalJoinClause,
isDistinct: Boolean,
deserializer: DeserializationStrategy,
connection: DatabaseConnection,
container: StatementContainer,
) : JoinSelectStatement =
JoinSelectStatement(buildSQL(table, clause, isDistinct, deserializer), deserializer, connection, container, null)
fun select(
table: Table<*>,
clause: JoinClause,
isDistinct: Boolean,
deserializer: DeserializationStrategy,
connection: DatabaseConnection,
container: StatementContainer,
addSelectStatement: (SelectStatement) -> Unit
) : JoinStatementWithoutCondition =
JoinStatementWithoutCondition(
buildSQL(table, clause, isDistinct, deserializer),
deserializer,
connection,
container,
addSelectStatement,
)
private fun buildSQL(
table: Table<*>,
clause: SelectClause,
isDistinct: Boolean,
deserializer: DeserializationStrategy,
): String = buildString {
append(sqlStr)
if (isDistinct)
append("DISTINCT ")
appendDBColumnName(deserializer.descriptor)
append(" FROM ")
append(table.tableName)
append(clause.clauseStr)
}
fun select(
table: Table,
isDistinct: Boolean,
deserializer: DeserializationStrategy,
connection: DatabaseConnection,
container: StatementContainer,
): FinalSelectStatement {
val sql = buildString {
append(sqlStr)
if (isDistinct)
append("DISTINCT ")
appendDBColumnName(deserializer.descriptor)
append(" FROM ")
append(table.tableName)
}
return FinalSelectStatement(sql, deserializer, connection, container, null)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy