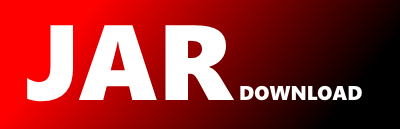
Model.InlineResponse401 Maven / Gradle / Ivy
/*
* CyberSource Merged Spec
* All CyberSource API specs merged together. These are available at https://developer.cybersource.com/api/reference/api-reference.html
*
* OpenAPI spec version: 0.0.1
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package Model;
import java.util.Objects;
import java.util.Arrays;
import Model.InlineResponse401Fields;
import Model.InlineResponse401Links;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
/**
* InlineResponse401
*/
public class InlineResponse401 {
@SerializedName("_links")
private InlineResponse401Links links = null;
@SerializedName("code")
private String code = null;
@SerializedName("correlationId")
private String correlationId = null;
@SerializedName("detail")
private String detail = null;
@SerializedName("fields")
private List fields = null;
@SerializedName("localizationKey")
private String localizationKey = null;
@SerializedName("message")
private String message = null;
public InlineResponse401 links(InlineResponse401Links links) {
this.links = links;
return this;
}
/**
* Get links
* @return links
**/
@ApiModelProperty(value = "")
public InlineResponse401Links getLinks() {
return links;
}
public void setLinks(InlineResponse401Links links) {
this.links = links;
}
public InlineResponse401 code(String code) {
this.code = code;
return this;
}
/**
* Valid Values: * FORBIDDEN_RESPONSE * VALIDATION_ERROR * UNSUPPORTED_MEDIA_TYPE * MALFORMED_PAYLOAD_ERROR * SERVER_ERROR
* @return code
**/
@ApiModelProperty(value = "Valid Values: * FORBIDDEN_RESPONSE * VALIDATION_ERROR * UNSUPPORTED_MEDIA_TYPE * MALFORMED_PAYLOAD_ERROR * SERVER_ERROR ")
public String getCode() {
return code;
}
public void setCode(String code) {
this.code = code;
}
public InlineResponse401 correlationId(String correlationId) {
this.correlationId = correlationId;
return this;
}
/**
* Get correlationId
* @return correlationId
**/
@ApiModelProperty(example = "c7b74452a7314f9ca28197d1084447a5", value = "")
public String getCorrelationId() {
return correlationId;
}
public void setCorrelationId(String correlationId) {
this.correlationId = correlationId;
}
public InlineResponse401 detail(String detail) {
this.detail = detail;
return this;
}
/**
* Get detail
* @return detail
**/
@ApiModelProperty(example = "One or more fields failed validation", value = "")
public String getDetail() {
return detail;
}
public void setDetail(String detail) {
this.detail = detail;
}
public InlineResponse401 fields(List fields) {
this.fields = fields;
return this;
}
public InlineResponse401 addFieldsItem(InlineResponse401Fields fieldsItem) {
if (this.fields == null) {
this.fields = new ArrayList();
}
this.fields.add(fieldsItem);
return this;
}
/**
* Get fields
* @return fields
**/
@ApiModelProperty(value = "")
public List getFields() {
return fields;
}
public void setFields(List fields) {
this.fields = fields;
}
public InlineResponse401 localizationKey(String localizationKey) {
this.localizationKey = localizationKey;
return this;
}
/**
* Valid Values: * cybsapi.forbidden.response * cybsapi.validation.error * cybsapi.media.notsupported
* @return localizationKey
**/
@ApiModelProperty(value = "Valid Values: * cybsapi.forbidden.response * cybsapi.validation.error * cybsapi.media.notsupported ")
public String getLocalizationKey() {
return localizationKey;
}
public void setLocalizationKey(String localizationKey) {
this.localizationKey = localizationKey;
}
public InlineResponse401 message(String message) {
this.message = message;
return this;
}
/**
* Get message
* @return message
**/
@ApiModelProperty(example = "Field validation error", value = "")
public String getMessage() {
return message;
}
public void setMessage(String message) {
this.message = message;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
InlineResponse401 inlineResponse401 = (InlineResponse401) o;
return Objects.equals(this.links, inlineResponse401.links) &&
Objects.equals(this.code, inlineResponse401.code) &&
Objects.equals(this.correlationId, inlineResponse401.correlationId) &&
Objects.equals(this.detail, inlineResponse401.detail) &&
Objects.equals(this.fields, inlineResponse401.fields) &&
Objects.equals(this.localizationKey, inlineResponse401.localizationKey) &&
Objects.equals(this.message, inlineResponse401.message);
}
@Override
public int hashCode() {
return Objects.hash(links, code, correlationId, detail, fields, localizationKey, message);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class InlineResponse401 {\n");
sb.append(" links: ").append(toIndentedString(links)).append("\n");
sb.append(" code: ").append(toIndentedString(code)).append("\n");
sb.append(" correlationId: ").append(toIndentedString(correlationId)).append("\n");
sb.append(" detail: ").append(toIndentedString(detail)).append("\n");
sb.append(" fields: ").append(toIndentedString(fields)).append("\n");
sb.append(" localizationKey: ").append(toIndentedString(localizationKey)).append("\n");
sb.append(" message: ").append(toIndentedString(message)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy