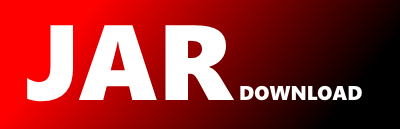
Model.Ptsv2paymentsSenderInformation Maven / Gradle / Ivy
/*
* CyberSource Merged Spec
* All CyberSource API specs merged together. These are available at https://developer.cybersource.com/api/reference/api-reference.html
*
* OpenAPI spec version: 0.0.1
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package Model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
/**
* Ptsv2paymentsSenderInformation
*/
public class Ptsv2paymentsSenderInformation {
@SerializedName("firstName")
private String firstName = null;
@SerializedName("lastName")
private String lastName = null;
@SerializedName("middleName")
private String middleName = null;
@SerializedName("address1")
private String address1 = null;
@SerializedName("locality")
private String locality = null;
@SerializedName("administrativeArea")
private String administrativeArea = null;
@SerializedName("countryCode")
private String countryCode = null;
public Ptsv2paymentsSenderInformation firstName(String firstName) {
this.firstName = firstName;
return this;
}
/**
* First name of the sender. **Applicable for Barclays AFT transactions only.** The field is mandatory for Visa and not applicable for Mastercard AFT. Only alpha numeric values are supported. Special characters not in the standard ASCII character set, are not supported and will be stripped before being sent to Barclays. For Visa, the maximum length of First Name, Middle Name and Last Name is 30 characters. Values exceeding the above limits will be truncated.
* @return firstName
**/
@ApiModelProperty(value = "First name of the sender. **Applicable for Barclays AFT transactions only.** The field is mandatory for Visa and not applicable for Mastercard AFT. Only alpha numeric values are supported. Special characters not in the standard ASCII character set, are not supported and will be stripped before being sent to Barclays. For Visa, the maximum length of First Name, Middle Name and Last Name is 30 characters. Values exceeding the above limits will be truncated. ")
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public Ptsv2paymentsSenderInformation lastName(String lastName) {
this.lastName = lastName;
return this;
}
/**
* Last name of the sender. **Applicable for Barclays AFT transactions only.** This field is optional for Visa and not applicable for Mastercard AFT. Only alpha numeric values are supported. Special characters not in the standard ASCII character set, are not supported and will be stripped before being sent to Barclays. For Visa, the maximum length of First Name, Middle Name and Last Name is 30 characters. Values exceeding these limits will be truncated.
* @return lastName
**/
@ApiModelProperty(value = "Last name of the sender. **Applicable for Barclays AFT transactions only.** This field is optional for Visa and not applicable for Mastercard AFT. Only alpha numeric values are supported. Special characters not in the standard ASCII character set, are not supported and will be stripped before being sent to Barclays. For Visa, the maximum length of First Name, Middle Name and Last Name is 30 characters. Values exceeding these limits will be truncated. ")
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
public Ptsv2paymentsSenderInformation middleName(String middleName) {
this.middleName = middleName;
return this;
}
/**
* Middle name of the sender. **Applicable for Barclays AFT transactions only.** The field is mandatory for Visa and not applicable for Mastercard AFT. Only alpha numeric values are supported. Special characters not in the standard ASCII character set, are not supported and will be stripped before being sent to Barclays. For Visa, the maximum length of First Name, Middle Name and Last Name is 30 characters. Values exceeding these limits will be truncated.
* @return middleName
**/
@ApiModelProperty(value = "Middle name of the sender. **Applicable for Barclays AFT transactions only.** The field is mandatory for Visa and not applicable for Mastercard AFT. Only alpha numeric values are supported. Special characters not in the standard ASCII character set, are not supported and will be stripped before being sent to Barclays. For Visa, the maximum length of First Name, Middle Name and Last Name is 30 characters. Values exceeding these limits will be truncated. ")
public String getMiddleName() {
return middleName;
}
public void setMiddleName(String middleName) {
this.middleName = middleName;
}
public Ptsv2paymentsSenderInformation address1(String address1) {
this.address1 = address1;
return this;
}
/**
* The street address of the sender. **Applicable for Barclays AFT transactions only.** The field is mandatory for Visa and not applicable for Mastercard AFT. Only alpha numeric values are supported. Special characters not in the standard ASCII character set are not supported and will be stripped before being sent to Barclays. The field has a maximum length of 35 characters. Values exceeding these limits will be truncated.
* @return address1
**/
@ApiModelProperty(value = "The street address of the sender. **Applicable for Barclays AFT transactions only.** The field is mandatory for Visa and not applicable for Mastercard AFT. Only alpha numeric values are supported. Special characters not in the standard ASCII character set are not supported and will be stripped before being sent to Barclays. The field has a maximum length of 35 characters. Values exceeding these limits will be truncated. ")
public String getAddress1() {
return address1;
}
public void setAddress1(String address1) {
this.address1 = address1;
}
public Ptsv2paymentsSenderInformation locality(String locality) {
this.locality = locality;
return this;
}
/**
* The city or locality of the sender.in **Applicable for Barclays AFT transactions only.** The field is optional for Visa and not applicable for Mastercard AFT. Only alpha numeric values are supported. Special characters not in the standard ASCII character set are not supported and will be stripped before being sent to Barclays. The field has a maximum length of 25 characters. Values exceeding these limits will be truncated.
* @return locality
**/
@ApiModelProperty(value = "The city or locality of the sender.in **Applicable for Barclays AFT transactions only.** The field is optional for Visa and not applicable for Mastercard AFT. Only alpha numeric values are supported. Special characters not in the standard ASCII character set are not supported and will be stripped before being sent to Barclays. The field has a maximum length of 25 characters. Values exceeding these limits will be truncated. ")
public String getLocality() {
return locality;
}
public void setLocality(String locality) {
this.locality = locality;
}
public Ptsv2paymentsSenderInformation administrativeArea(String administrativeArea) {
this.administrativeArea = administrativeArea;
return this;
}
/**
* The state or province of the sender. **Applicable for Barclays AFT transactions only.** The field is mandatory for Visa AFT when the sender country is US or CA else it is optional for Visa AFT. This field is not applicable for Mastercard AFT. Must be a two character value
* @return administrativeArea
**/
@ApiModelProperty(value = "The state or province of the sender. **Applicable for Barclays AFT transactions only.** The field is mandatory for Visa AFT when the sender country is US or CA else it is optional for Visa AFT. This field is not applicable for Mastercard AFT. Must be a two character value ")
public String getAdministrativeArea() {
return administrativeArea;
}
public void setAdministrativeArea(String administrativeArea) {
this.administrativeArea = administrativeArea;
}
public Ptsv2paymentsSenderInformation countryCode(String countryCode) {
this.countryCode = countryCode;
return this;
}
/**
* The country associated with the address of the sender. **Applicable for Barclays AFT transactions only.** The field is mandatory for Visa and not applicable for Mastercard AFT. Must be a two character ISO country code. For example, see [ISO Country Code](https://developer.cybersource.com/docs/cybs/en-us/country-codes/reference/all/na/country-codes/country-codes.html)
* @return countryCode
**/
@ApiModelProperty(value = "The country associated with the address of the sender. **Applicable for Barclays AFT transactions only.** The field is mandatory for Visa and not applicable for Mastercard AFT. Must be a two character ISO country code. For example, see [ISO Country Code](https://developer.cybersource.com/docs/cybs/en-us/country-codes/reference/all/na/country-codes/country-codes.html) ")
public String getCountryCode() {
return countryCode;
}
public void setCountryCode(String countryCode) {
this.countryCode = countryCode;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Ptsv2paymentsSenderInformation ptsv2paymentsSenderInformation = (Ptsv2paymentsSenderInformation) o;
return Objects.equals(this.firstName, ptsv2paymentsSenderInformation.firstName) &&
Objects.equals(this.lastName, ptsv2paymentsSenderInformation.lastName) &&
Objects.equals(this.middleName, ptsv2paymentsSenderInformation.middleName) &&
Objects.equals(this.address1, ptsv2paymentsSenderInformation.address1) &&
Objects.equals(this.locality, ptsv2paymentsSenderInformation.locality) &&
Objects.equals(this.administrativeArea, ptsv2paymentsSenderInformation.administrativeArea) &&
Objects.equals(this.countryCode, ptsv2paymentsSenderInformation.countryCode);
}
@Override
public int hashCode() {
return Objects.hash(firstName, lastName, middleName, address1, locality, administrativeArea, countryCode);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Ptsv2paymentsSenderInformation {\n");
sb.append(" firstName: ").append(toIndentedString(firstName)).append("\n");
sb.append(" lastName: ").append(toIndentedString(lastName)).append("\n");
sb.append(" middleName: ").append(toIndentedString(middleName)).append("\n");
sb.append(" address1: ").append(toIndentedString(address1)).append("\n");
sb.append(" locality: ").append(toIndentedString(locality)).append("\n");
sb.append(" administrativeArea: ").append(toIndentedString(administrativeArea)).append("\n");
sb.append(" countryCode: ").append(toIndentedString(countryCode)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy