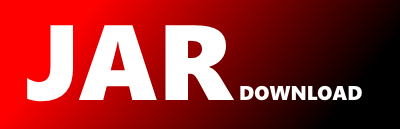
Model.Ptsv2paymentsTravelInformationAutoRental Maven / Gradle / Ivy
/*
* CyberSource Merged Spec
* All CyberSource API specs merged together. These are available at https://developer.cybersource.com/api/reference/api-reference.html
*
* OpenAPI spec version: 0.0.1
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package Model;
import java.util.Objects;
import java.util.Arrays;
import Model.Ptsv2paymentsTravelInformationAutoRentalRentalAddress;
import Model.Ptsv2paymentsTravelInformationAutoRentalReturnAddress;
import Model.Ptsv2paymentsTravelInformationAutoRentalTaxDetails;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
/**
* Ptsv2paymentsTravelInformationAutoRental
*/
public class Ptsv2paymentsTravelInformationAutoRental {
@SerializedName("noShowIndicator")
private Boolean noShowIndicator = null;
@SerializedName("customerName")
private String customerName = null;
@SerializedName("vehicleClass")
private String vehicleClass = null;
@SerializedName("distanceTravelled")
private String distanceTravelled = null;
@SerializedName("distanceUnit")
private String distanceUnit = null;
@SerializedName("returnDateTime")
private String returnDateTime = null;
@SerializedName("rentalDateTime")
private String rentalDateTime = null;
@SerializedName("maxFreeDistance")
private String maxFreeDistance = null;
@SerializedName("insuranceIndicator")
private Boolean insuranceIndicator = null;
@SerializedName("programCode")
private String programCode = null;
@SerializedName("returnAddress")
private Ptsv2paymentsTravelInformationAutoRentalReturnAddress returnAddress = null;
@SerializedName("rentalAddress")
private Ptsv2paymentsTravelInformationAutoRentalRentalAddress rentalAddress = null;
@SerializedName("agreementNumber")
private String agreementNumber = null;
@SerializedName("odometerReading")
private String odometerReading = null;
@SerializedName("vehicleIdentificationNumber")
private String vehicleIdentificationNumber = null;
@SerializedName("companyId")
private String companyId = null;
@SerializedName("numberOfAdditionalDrivers")
private String numberOfAdditionalDrivers = null;
@SerializedName("driverAge")
private String driverAge = null;
@SerializedName("specialProgramCode")
private String specialProgramCode = null;
@SerializedName("vehicleMake")
private String vehicleMake = null;
@SerializedName("vehicleModel")
private String vehicleModel = null;
@SerializedName("timePeriod")
private String timePeriod = null;
@SerializedName("commodityCode")
private String commodityCode = null;
@SerializedName("customerServicePhoneNumber")
private String customerServicePhoneNumber = null;
@SerializedName("taxDetails")
private Ptsv2paymentsTravelInformationAutoRentalTaxDetails taxDetails = null;
@SerializedName("insuranceAmount")
private String insuranceAmount = null;
@SerializedName("oneWayDropOffAmount")
private String oneWayDropOffAmount = null;
@SerializedName("adjustedAmountIndicator")
private String adjustedAmountIndicator = null;
@SerializedName("adjustedAmount")
private String adjustedAmount = null;
@SerializedName("fuelCharges")
private String fuelCharges = null;
@SerializedName("weeklyRentalRate")
private String weeklyRentalRate = null;
@SerializedName("dailyRentalRate")
private String dailyRentalRate = null;
@SerializedName("ratePerMile")
private String ratePerMile = null;
@SerializedName("mileageCharge")
private String mileageCharge = null;
@SerializedName("extraMileageCharge")
private String extraMileageCharge = null;
@SerializedName("lateFeeAmount")
private String lateFeeAmount = null;
@SerializedName("towingCharge")
private String towingCharge = null;
@SerializedName("extraCharge")
private String extraCharge = null;
@SerializedName("gpsCharge")
private String gpsCharge = null;
@SerializedName("phoneCharge")
private String phoneCharge = null;
@SerializedName("parkingViolationCharge")
private String parkingViolationCharge = null;
@SerializedName("otherCharges")
private String otherCharges = null;
@SerializedName("companyName")
private String companyName = null;
@SerializedName("affiliateName")
private String affiliateName = null;
public Ptsv2paymentsTravelInformationAutoRental noShowIndicator(Boolean noShowIndicator) {
this.noShowIndicator = noShowIndicator;
return this;
}
/**
* No Show Indicator provides an indicator noting that the individual did not show up after making a reservation for a vehicle. Possible values: - true - false
* @return noShowIndicator
**/
@ApiModelProperty(value = "No Show Indicator provides an indicator noting that the individual did not show up after making a reservation for a vehicle. Possible values: - true - false ")
public Boolean isNoShowIndicator() {
return noShowIndicator;
}
public void setNoShowIndicator(Boolean noShowIndicator) {
this.noShowIndicator = noShowIndicator;
}
public Ptsv2paymentsTravelInformationAutoRental customerName(String customerName) {
this.customerName = customerName;
return this;
}
/**
* Name of the individual making the rental agreement. Valid data lengths by card: |Card Specific Validation|VISA|MasterCard|Discover|AMEX| |--- |--- |--- |--- | | Filed Length| 40| 40| 29| 26| | Field Type| AN| ANS| AN| AN| | M/O/C| O| M| M| M|
* @return customerName
**/
@ApiModelProperty(value = "Name of the individual making the rental agreement. Valid data lengths by card: |Card Specific Validation|VISA|MasterCard|Discover|AMEX| |--- |--- |--- |--- | | Filed Length| 40| 40| 29| 26| | Field Type| AN| ANS| AN| AN| | M/O/C| O| M| M| M| ")
public String getCustomerName() {
return customerName;
}
public void setCustomerName(String customerName) {
this.customerName = customerName;
}
public Ptsv2paymentsTravelInformationAutoRental vehicleClass(String vehicleClass) {
this.vehicleClass = vehicleClass;
return this;
}
/**
* Classification of the rented auto. **NOTE** For VISA, this is a 2-byte optional code. Valid values for American Express & MasterCard: |American Express |MasterCard |Description| |--- |--- |--- | | 0001| 0001| Mini| | 0002| 0002| Subcompact| | 0003| 0003| Economy| | 0004| 0004| Compact| | 0005| 0005| Midsize| | 0006| 0006| Intermediate| | 0007| 0007| Standard| | 0008| 0008| Fulll size| | 0009| 0009| Luxury| | 0010| 0010| Premium| | 0011| 0011| Minivan| | 0012| 0012| 12-passenger van| | 0013| 0013| Moving van| | 0014| 0014| 15-passenger van| | 0015| 0015| Cargo van| | 0016| 0016| 12-foot truck| | 0017| 0017| 20-foot truck| | 0018| 0018| 24-foot truck| | 0019| 0019| 26-foot truck| | 0020| 0020| Moped| | 0021| 0021| Stretch| | 0022| 0022| Regular| | 0023| 0023| Unique| | 0024| 0024| Exotic| | 0025| 0025| Small/medium truck| | 0026| 0026| Large truck| | 0027| 0027| Small SUV| | 0028| 0028| Medium SUV| | 0029| 0029| Large SUV| | 0030| 0030| Exotic SUV| | 9999| 9999| Miscellaneous| Additional Values allowed **only** for `American Express`: |American Express|MasterCard|Description| |--- |--- |--- | | 0031| NA| Four Wheel Drive| | 0032| NA| Special| | 0099| NA| Taxi|
* @return vehicleClass
**/
@ApiModelProperty(value = "Classification of the rented auto. **NOTE** For VISA, this is a 2-byte optional code. Valid values for American Express & MasterCard: |American Express |MasterCard |Description| |--- |--- |--- | | 0001| 0001| Mini| | 0002| 0002| Subcompact| | 0003| 0003| Economy| | 0004| 0004| Compact| | 0005| 0005| Midsize| | 0006| 0006| Intermediate| | 0007| 0007| Standard| | 0008| 0008| Fulll size| | 0009| 0009| Luxury| | 0010| 0010| Premium| | 0011| 0011| Minivan| | 0012| 0012| 12-passenger van| | 0013| 0013| Moving van| | 0014| 0014| 15-passenger van| | 0015| 0015| Cargo van| | 0016| 0016| 12-foot truck| | 0017| 0017| 20-foot truck| | 0018| 0018| 24-foot truck| | 0019| 0019| 26-foot truck| | 0020| 0020| Moped| | 0021| 0021| Stretch| | 0022| 0022| Regular| | 0023| 0023| Unique| | 0024| 0024| Exotic| | 0025| 0025| Small/medium truck| | 0026| 0026| Large truck| | 0027| 0027| Small SUV| | 0028| 0028| Medium SUV| | 0029| 0029| Large SUV| | 0030| 0030| Exotic SUV| | 9999| 9999| Miscellaneous| Additional Values allowed **only** for `American Express`: |American Express|MasterCard|Description| |--- |--- |--- | | 0031| NA| Four Wheel Drive| | 0032| NA| Special| | 0099| NA| Taxi| ")
public String getVehicleClass() {
return vehicleClass;
}
public void setVehicleClass(String vehicleClass) {
this.vehicleClass = vehicleClass;
}
public Ptsv2paymentsTravelInformationAutoRental distanceTravelled(String distanceTravelled) {
this.distanceTravelled = distanceTravelled;
return this;
}
/**
* Total number of miles driven by the customer. This field is supported only for MasterCard and American Express.
* @return distanceTravelled
**/
@ApiModelProperty(value = "Total number of miles driven by the customer. This field is supported only for MasterCard and American Express. ")
public String getDistanceTravelled() {
return distanceTravelled;
}
public void setDistanceTravelled(String distanceTravelled) {
this.distanceTravelled = distanceTravelled;
}
public Ptsv2paymentsTravelInformationAutoRental distanceUnit(String distanceUnit) {
this.distanceUnit = distanceUnit;
return this;
}
/**
* Miles/Kilometers Indicator shows whether the \"miles\" fields are expressed in miles or kilometers. Allowed values: - `K` - Kilometers - `M` - Miles
* @return distanceUnit
**/
@ApiModelProperty(value = "Miles/Kilometers Indicator shows whether the \"miles\" fields are expressed in miles or kilometers. Allowed values: - `K` - Kilometers - `M` - Miles ")
public String getDistanceUnit() {
return distanceUnit;
}
public void setDistanceUnit(String distanceUnit) {
this.distanceUnit = distanceUnit;
}
public Ptsv2paymentsTravelInformationAutoRental returnDateTime(String returnDateTime) {
this.returnDateTime = returnDateTime;
return this;
}
/**
* Date/time the auto was returned to the rental agency. Format: ``yyyy-MM-dd HH-mm-ss z`` This field is supported for Visa, MasterCard, and American Express.
* @return returnDateTime
**/
@ApiModelProperty(value = "Date/time the auto was returned to the rental agency. Format: ``yyyy-MM-dd HH-mm-ss z`` This field is supported for Visa, MasterCard, and American Express. ")
public String getReturnDateTime() {
return returnDateTime;
}
public void setReturnDateTime(String returnDateTime) {
this.returnDateTime = returnDateTime;
}
public Ptsv2paymentsTravelInformationAutoRental rentalDateTime(String rentalDateTime) {
this.rentalDateTime = rentalDateTime;
return this;
}
/**
* Date/time the auto was picked up from the rental agency. Format: `yyyy-MM-dd HH-mm-ss z` This field is supported for Visa, MasterCard, and American Express.
* @return rentalDateTime
**/
@ApiModelProperty(value = "Date/time the auto was picked up from the rental agency. Format: `yyyy-MM-dd HH-mm-ss z` This field is supported for Visa, MasterCard, and American Express. ")
public String getRentalDateTime() {
return rentalDateTime;
}
public void setRentalDateTime(String rentalDateTime) {
this.rentalDateTime = rentalDateTime;
}
public Ptsv2paymentsTravelInformationAutoRental maxFreeDistance(String maxFreeDistance) {
this.maxFreeDistance = maxFreeDistance;
return this;
}
/**
* Maximum number of free miles or kilometers allowed to a customer for the duration of the auto rental agreement. This field is supported only for MasterCard and American Express.
* @return maxFreeDistance
**/
@ApiModelProperty(value = "Maximum number of free miles or kilometers allowed to a customer for the duration of the auto rental agreement. This field is supported only for MasterCard and American Express. ")
public String getMaxFreeDistance() {
return maxFreeDistance;
}
public void setMaxFreeDistance(String maxFreeDistance) {
this.maxFreeDistance = maxFreeDistance;
}
public Ptsv2paymentsTravelInformationAutoRental insuranceIndicator(Boolean insuranceIndicator) {
this.insuranceIndicator = insuranceIndicator;
return this;
}
/**
* Used for MC and Discover Valid values: - `true` - Yes (insurance was purchased) - `false` - No (insurance was not purchased)
* @return insuranceIndicator
**/
@ApiModelProperty(value = "Used for MC and Discover Valid values: - `true` - Yes (insurance was purchased) - `false` - No (insurance was not purchased) ")
public Boolean isInsuranceIndicator() {
return insuranceIndicator;
}
public void setInsuranceIndicator(Boolean insuranceIndicator) {
this.insuranceIndicator = insuranceIndicator;
}
public Ptsv2paymentsTravelInformationAutoRental programCode(String programCode) {
this.programCode = programCode;
return this;
}
/**
* Used to identify special circumstances applicable to the Card Transaction or Cardholder, such as \"renter\" or \"show\". This code is `2 digit` value agreed by Merchant and processor.
* @return programCode
**/
@ApiModelProperty(value = "Used to identify special circumstances applicable to the Card Transaction or Cardholder, such as \"renter\" or \"show\". This code is `2 digit` value agreed by Merchant and processor. ")
public String getProgramCode() {
return programCode;
}
public void setProgramCode(String programCode) {
this.programCode = programCode;
}
public Ptsv2paymentsTravelInformationAutoRental returnAddress(Ptsv2paymentsTravelInformationAutoRentalReturnAddress returnAddress) {
this.returnAddress = returnAddress;
return this;
}
/**
* Get returnAddress
* @return returnAddress
**/
@ApiModelProperty(value = "")
public Ptsv2paymentsTravelInformationAutoRentalReturnAddress getReturnAddress() {
return returnAddress;
}
public void setReturnAddress(Ptsv2paymentsTravelInformationAutoRentalReturnAddress returnAddress) {
this.returnAddress = returnAddress;
}
public Ptsv2paymentsTravelInformationAutoRental rentalAddress(Ptsv2paymentsTravelInformationAutoRentalRentalAddress rentalAddress) {
this.rentalAddress = rentalAddress;
return this;
}
/**
* Get rentalAddress
* @return rentalAddress
**/
@ApiModelProperty(value = "")
public Ptsv2paymentsTravelInformationAutoRentalRentalAddress getRentalAddress() {
return rentalAddress;
}
public void setRentalAddress(Ptsv2paymentsTravelInformationAutoRentalRentalAddress rentalAddress) {
this.rentalAddress = rentalAddress;
}
public Ptsv2paymentsTravelInformationAutoRental agreementNumber(String agreementNumber) {
this.agreementNumber = agreementNumber;
return this;
}
/**
* Auto rental agency's agreement (invoice) number provided to the customer. It is used to trace any inquiries about transactions. This field is supported for Visa, MasterCard, and American Express. This Merchant-defined value, which may be composed of any combination of characters and/or numerals, may become part of the descriptive bill on the Cardmember's statement.
* @return agreementNumber
**/
@ApiModelProperty(value = "Auto rental agency's agreement (invoice) number provided to the customer. It is used to trace any inquiries about transactions. This field is supported for Visa, MasterCard, and American Express. This Merchant-defined value, which may be composed of any combination of characters and/or numerals, may become part of the descriptive bill on the Cardmember's statement. ")
public String getAgreementNumber() {
return agreementNumber;
}
public void setAgreementNumber(String agreementNumber) {
this.agreementNumber = agreementNumber;
}
public Ptsv2paymentsTravelInformationAutoRental odometerReading(String odometerReading) {
this.odometerReading = odometerReading;
return this;
}
/**
* Odometer reading at time of vehicle rental.
* @return odometerReading
**/
@ApiModelProperty(value = "Odometer reading at time of vehicle rental. ")
public String getOdometerReading() {
return odometerReading;
}
public void setOdometerReading(String odometerReading) {
this.odometerReading = odometerReading;
}
public Ptsv2paymentsTravelInformationAutoRental vehicleIdentificationNumber(String vehicleIdentificationNumber) {
this.vehicleIdentificationNumber = vehicleIdentificationNumber;
return this;
}
/**
* This field contains a unique identifier assigned by the company to the vehicle.
* @return vehicleIdentificationNumber
**/
@ApiModelProperty(value = "This field contains a unique identifier assigned by the company to the vehicle. ")
public String getVehicleIdentificationNumber() {
return vehicleIdentificationNumber;
}
public void setVehicleIdentificationNumber(String vehicleIdentificationNumber) {
this.vehicleIdentificationNumber = vehicleIdentificationNumber;
}
public Ptsv2paymentsTravelInformationAutoRental companyId(String companyId) {
this.companyId = companyId;
return this;
}
/**
* Corporate Identifier provides the unique identifier of the corporation or entity renting the vehicle: |Card Specific Validation|VISA|MasterCard|Discover|AMEX| |--- |--- |--- |--- | | Filed Length| NA| 12| NA| NA| | Field Type| NA| AN| NA| NA| | M/O/C| NA| O| NA| NA|
* @return companyId
**/
@ApiModelProperty(value = "Corporate Identifier provides the unique identifier of the corporation or entity renting the vehicle: |Card Specific Validation|VISA|MasterCard|Discover|AMEX| |--- |--- |--- |--- | | Filed Length| NA| 12| NA| NA| | Field Type| NA| AN| NA| NA| | M/O/C| NA| O| NA| NA| ")
public String getCompanyId() {
return companyId;
}
public void setCompanyId(String companyId) {
this.companyId = companyId;
}
public Ptsv2paymentsTravelInformationAutoRental numberOfAdditionalDrivers(String numberOfAdditionalDrivers) {
this.numberOfAdditionalDrivers = numberOfAdditionalDrivers;
return this;
}
/**
* The number of additional drivers included on the rental agreement not including the individual who signed the rental agreement.
* @return numberOfAdditionalDrivers
**/
@ApiModelProperty(value = "The number of additional drivers included on the rental agreement not including the individual who signed the rental agreement. ")
public String getNumberOfAdditionalDrivers() {
return numberOfAdditionalDrivers;
}
public void setNumberOfAdditionalDrivers(String numberOfAdditionalDrivers) {
this.numberOfAdditionalDrivers = numberOfAdditionalDrivers;
}
public Ptsv2paymentsTravelInformationAutoRental driverAge(String driverAge) {
this.driverAge = driverAge;
return this;
}
/**
* Age of the driver renting the vehicle.
* @return driverAge
**/
@ApiModelProperty(value = "Age of the driver renting the vehicle. ")
public String getDriverAge() {
return driverAge;
}
public void setDriverAge(String driverAge) {
this.driverAge = driverAge;
}
public Ptsv2paymentsTravelInformationAutoRental specialProgramCode(String specialProgramCode) {
this.specialProgramCode = specialProgramCode;
return this;
}
/**
* Program code used to identify special circumstances, such as \"frequent renter\" or \"no show\" status for the renter. Possible values: - `0`: not applicable (default) - `1`: frequent renter - `2`: no show For authorizations, this field is supported only for Visa. For captures, this field is supported for Visa, MasterCard, and American Express. Code for special programs applicable to the Card Transaction or the Cardholder.
* @return specialProgramCode
**/
@ApiModelProperty(value = "Program code used to identify special circumstances, such as \"frequent renter\" or \"no show\" status for the renter. Possible values: - `0`: not applicable (default) - `1`: frequent renter - `2`: no show For authorizations, this field is supported only for Visa. For captures, this field is supported for Visa, MasterCard, and American Express. Code for special programs applicable to the Card Transaction or the Cardholder. ")
public String getSpecialProgramCode() {
return specialProgramCode;
}
public void setSpecialProgramCode(String specialProgramCode) {
this.specialProgramCode = specialProgramCode;
}
public Ptsv2paymentsTravelInformationAutoRental vehicleMake(String vehicleMake) {
this.vehicleMake = vehicleMake;
return this;
}
/**
* Make of the vehicle being rented (e.g., Chevrolet or Ford).
* @return vehicleMake
**/
@ApiModelProperty(value = "Make of the vehicle being rented (e.g., Chevrolet or Ford). ")
public String getVehicleMake() {
return vehicleMake;
}
public void setVehicleMake(String vehicleMake) {
this.vehicleMake = vehicleMake;
}
public Ptsv2paymentsTravelInformationAutoRental vehicleModel(String vehicleModel) {
this.vehicleModel = vehicleModel;
return this;
}
/**
* Model of the vehicle being rented (e.g., Cavalier or Focus).
* @return vehicleModel
**/
@ApiModelProperty(value = "Model of the vehicle being rented (e.g., Cavalier or Focus). ")
public String getVehicleModel() {
return vehicleModel;
}
public void setVehicleModel(String vehicleModel) {
this.vehicleModel = vehicleModel;
}
public Ptsv2paymentsTravelInformationAutoRental timePeriod(String timePeriod) {
this.timePeriod = timePeriod;
return this;
}
/**
* Indicates the time period for which the vehicle rental rate applies (e.g., daily, weekly or monthly). Daily, Weekly and Monthly are valid values.
* @return timePeriod
**/
@ApiModelProperty(value = "Indicates the time period for which the vehicle rental rate applies (e.g., daily, weekly or monthly). Daily, Weekly and Monthly are valid values. ")
public String getTimePeriod() {
return timePeriod;
}
public void setTimePeriod(String timePeriod) {
this.timePeriod = timePeriod;
}
public Ptsv2paymentsTravelInformationAutoRental commodityCode(String commodityCode) {
this.commodityCode = commodityCode;
return this;
}
/**
* Commodity code or International description code used to classify the item. Contact your acquirer for a list of codes.
* @return commodityCode
**/
@ApiModelProperty(value = "Commodity code or International description code used to classify the item. Contact your acquirer for a list of codes. ")
public String getCommodityCode() {
return commodityCode;
}
public void setCommodityCode(String commodityCode) {
this.commodityCode = commodityCode;
}
public Ptsv2paymentsTravelInformationAutoRental customerServicePhoneNumber(String customerServicePhoneNumber) {
this.customerServicePhoneNumber = customerServicePhoneNumber;
return this;
}
/**
* Customer service telephone number that is used to resolve questions or disputes. Include the area code, exchange, and number. This field is supported only for MasterCard and American Express.
* @return customerServicePhoneNumber
**/
@ApiModelProperty(value = "Customer service telephone number that is used to resolve questions or disputes. Include the area code, exchange, and number. This field is supported only for MasterCard and American Express. ")
public String getCustomerServicePhoneNumber() {
return customerServicePhoneNumber;
}
public void setCustomerServicePhoneNumber(String customerServicePhoneNumber) {
this.customerServicePhoneNumber = customerServicePhoneNumber;
}
public Ptsv2paymentsTravelInformationAutoRental taxDetails(Ptsv2paymentsTravelInformationAutoRentalTaxDetails taxDetails) {
this.taxDetails = taxDetails;
return this;
}
/**
* Get taxDetails
* @return taxDetails
**/
@ApiModelProperty(value = "")
public Ptsv2paymentsTravelInformationAutoRentalTaxDetails getTaxDetails() {
return taxDetails;
}
public void setTaxDetails(Ptsv2paymentsTravelInformationAutoRentalTaxDetails taxDetails) {
this.taxDetails = taxDetails;
}
public Ptsv2paymentsTravelInformationAutoRental insuranceAmount(String insuranceAmount) {
this.insuranceAmount = insuranceAmount;
return this;
}
/**
* Insurance charges. Field is conditional and can include decimal point.
* @return insuranceAmount
**/
@ApiModelProperty(value = "Insurance charges. Field is conditional and can include decimal point. ")
public String getInsuranceAmount() {
return insuranceAmount;
}
public void setInsuranceAmount(String insuranceAmount) {
this.insuranceAmount = insuranceAmount;
}
public Ptsv2paymentsTravelInformationAutoRental oneWayDropOffAmount(String oneWayDropOffAmount) {
this.oneWayDropOffAmount = oneWayDropOffAmount;
return this;
}
/**
* Extra charges incurred for a one-way rental agreement for the auto. This field is supported only for Visa.
* @return oneWayDropOffAmount
**/
@ApiModelProperty(value = "Extra charges incurred for a one-way rental agreement for the auto. This field is supported only for Visa. ")
public String getOneWayDropOffAmount() {
return oneWayDropOffAmount;
}
public void setOneWayDropOffAmount(String oneWayDropOffAmount) {
this.oneWayDropOffAmount = oneWayDropOffAmount;
}
public Ptsv2paymentsTravelInformationAutoRental adjustedAmountIndicator(String adjustedAmountIndicator) {
this.adjustedAmountIndicator = adjustedAmountIndicator;
return this;
}
/**
* For **MasterCard** and **Discover**: Adjusted amount indicator code that indicates any miscellaneous charges incurred after the auto was returned. Possible values: - `A` - Drop-off charges - `B` - Delivery charges - `C` - Parking expenses - `D` - Extra hours - `E` - Violations - `X` - More than one of the above charges For **American Express**: Audit indicator code that indicates any adjustment for mileage, fuel, auto damage, etc. made to a rental agreement and whether the cardholder was notified. Possible value for the authorization service: - `A` (default): adjustment amount greater than 0 (zero) Possible values for the capture service: - `X` - Multiple adjustments - `Y` - One adjustment only; Cardmember notified - `Z` - One adjustment only; Cardmember not notified. This value is used as the default if the request does not include this field and includes an adjustment amount greater than 0 (zero). This is an optional field.
* @return adjustedAmountIndicator
**/
@ApiModelProperty(value = "For **MasterCard** and **Discover**: Adjusted amount indicator code that indicates any miscellaneous charges incurred after the auto was returned. Possible values: - `A` - Drop-off charges - `B` - Delivery charges - `C` - Parking expenses - `D` - Extra hours - `E` - Violations - `X` - More than one of the above charges For **American Express**: Audit indicator code that indicates any adjustment for mileage, fuel, auto damage, etc. made to a rental agreement and whether the cardholder was notified. Possible value for the authorization service: - `A` (default): adjustment amount greater than 0 (zero) Possible values for the capture service: - `X` - Multiple adjustments - `Y` - One adjustment only; Cardmember notified - `Z` - One adjustment only; Cardmember not notified. This value is used as the default if the request does not include this field and includes an adjustment amount greater than 0 (zero). This is an optional field. ")
public String getAdjustedAmountIndicator() {
return adjustedAmountIndicator;
}
public void setAdjustedAmountIndicator(String adjustedAmountIndicator) {
this.adjustedAmountIndicator = adjustedAmountIndicator;
}
public Ptsv2paymentsTravelInformationAutoRental adjustedAmount(String adjustedAmount) {
this.adjustedAmount = adjustedAmount;
return this;
}
/**
* Adjusted Amount indicates whether any miscellaneous charges were incurred after the vehicle was returned. For authorizations, this field is supported only for American Express. For captures, this field is supported only for MasterCard and American Express. **NOTE** For American Express, this field is required if the `travelInformation.autoRental.adjustedAmountIndicator` field is included in the request and has a value; otherwise, this field is optional. For all other card types, this field is ignored.
* @return adjustedAmount
**/
@ApiModelProperty(value = "Adjusted Amount indicates whether any miscellaneous charges were incurred after the vehicle was returned. For authorizations, this field is supported only for American Express. For captures, this field is supported only for MasterCard and American Express. **NOTE** For American Express, this field is required if the `travelInformation.autoRental.adjustedAmountIndicator` field is included in the request and has a value; otherwise, this field is optional. For all other card types, this field is ignored. ")
public String getAdjustedAmount() {
return adjustedAmount;
}
public void setAdjustedAmount(String adjustedAmount) {
this.adjustedAmount = adjustedAmount;
}
public Ptsv2paymentsTravelInformationAutoRental fuelCharges(String fuelCharges) {
this.fuelCharges = fuelCharges;
return this;
}
/**
* Extra gasoline charges that extend beyond the basic rental agreement. This field is supported only for Visa.
* @return fuelCharges
**/
@ApiModelProperty(value = "Extra gasoline charges that extend beyond the basic rental agreement. This field is supported only for Visa. ")
public String getFuelCharges() {
return fuelCharges;
}
public void setFuelCharges(String fuelCharges) {
this.fuelCharges = fuelCharges;
}
public Ptsv2paymentsTravelInformationAutoRental weeklyRentalRate(String weeklyRentalRate) {
this.weeklyRentalRate = weeklyRentalRate;
return this;
}
/**
* Weekly Rental Amount provides the amount charged for a seven-day rental period. Field - Time Period needs to be populated with Weekly if this field is present
* @return weeklyRentalRate
**/
@ApiModelProperty(value = "Weekly Rental Amount provides the amount charged for a seven-day rental period. Field - Time Period needs to be populated with Weekly if this field is present ")
public String getWeeklyRentalRate() {
return weeklyRentalRate;
}
public void setWeeklyRentalRate(String weeklyRentalRate) {
this.weeklyRentalRate = weeklyRentalRate;
}
public Ptsv2paymentsTravelInformationAutoRental dailyRentalRate(String dailyRentalRate) {
this.dailyRentalRate = dailyRentalRate;
return this;
}
/**
* Daily auto rental rate charged. This field is supported only for MasterCard and American Express. Field - Time Period needs to be populated with Daily if this field is present
* @return dailyRentalRate
**/
@ApiModelProperty(value = "Daily auto rental rate charged. This field is supported only for MasterCard and American Express. Field - Time Period needs to be populated with Daily if this field is present ")
public String getDailyRentalRate() {
return dailyRentalRate;
}
public void setDailyRentalRate(String dailyRentalRate) {
this.dailyRentalRate = dailyRentalRate;
}
public Ptsv2paymentsTravelInformationAutoRental ratePerMile(String ratePerMile) {
this.ratePerMile = ratePerMile;
return this;
}
/**
* Rate charged for each mile. This field is supported only for MasterCard and American Express.
* @return ratePerMile
**/
@ApiModelProperty(value = "Rate charged for each mile. This field is supported only for MasterCard and American Express. ")
public String getRatePerMile() {
return ratePerMile;
}
public void setRatePerMile(String ratePerMile) {
this.ratePerMile = ratePerMile;
}
public Ptsv2paymentsTravelInformationAutoRental mileageCharge(String mileageCharge) {
this.mileageCharge = mileageCharge;
return this;
}
/**
* Regular Mileage Charge provides the amount charged for regular miles traveled during vehicle rental. Two decimal places
* @return mileageCharge
**/
@ApiModelProperty(value = "Regular Mileage Charge provides the amount charged for regular miles traveled during vehicle rental. Two decimal places ")
public String getMileageCharge() {
return mileageCharge;
}
public void setMileageCharge(String mileageCharge) {
this.mileageCharge = mileageCharge;
}
public Ptsv2paymentsTravelInformationAutoRental extraMileageCharge(String extraMileageCharge) {
this.extraMileageCharge = extraMileageCharge;
return this;
}
/**
* Extra mileage charges that extend beyond the basic rental agreement. This field is supported only for Visa.
* @return extraMileageCharge
**/
@ApiModelProperty(value = "Extra mileage charges that extend beyond the basic rental agreement. This field is supported only for Visa. ")
public String getExtraMileageCharge() {
return extraMileageCharge;
}
public void setExtraMileageCharge(String extraMileageCharge) {
this.extraMileageCharge = extraMileageCharge;
}
public Ptsv2paymentsTravelInformationAutoRental lateFeeAmount(String lateFeeAmount) {
this.lateFeeAmount = lateFeeAmount;
return this;
}
/**
* Extra charges related to a late return of the rented auto. This field is supported only for Visa.
* @return lateFeeAmount
**/
@ApiModelProperty(value = "Extra charges related to a late return of the rented auto. This field is supported only for Visa. ")
public String getLateFeeAmount() {
return lateFeeAmount;
}
public void setLateFeeAmount(String lateFeeAmount) {
this.lateFeeAmount = lateFeeAmount;
}
public Ptsv2paymentsTravelInformationAutoRental towingCharge(String towingCharge) {
this.towingCharge = towingCharge;
return this;
}
/**
* (Towing Charges) provides the amount charged to tow the rental vehicle.
* @return towingCharge
**/
@ApiModelProperty(value = "(Towing Charges) provides the amount charged to tow the rental vehicle. ")
public String getTowingCharge() {
return towingCharge;
}
public void setTowingCharge(String towingCharge) {
this.towingCharge = towingCharge;
}
public Ptsv2paymentsTravelInformationAutoRental extraCharge(String extraCharge) {
this.extraCharge = extraCharge;
return this;
}
/**
* (Extra Charges) provides the extra charges associated with the vehicle rental.
* @return extraCharge
**/
@ApiModelProperty(value = "(Extra Charges) provides the extra charges associated with the vehicle rental. ")
public String getExtraCharge() {
return extraCharge;
}
public void setExtraCharge(String extraCharge) {
this.extraCharge = extraCharge;
}
public Ptsv2paymentsTravelInformationAutoRental gpsCharge(String gpsCharge) {
this.gpsCharge = gpsCharge;
return this;
}
/**
* Amount charged for renting a Global Positioning Service (GPS).
* @return gpsCharge
**/
@ApiModelProperty(value = "Amount charged for renting a Global Positioning Service (GPS). ")
public String getGpsCharge() {
return gpsCharge;
}
public void setGpsCharge(String gpsCharge) {
this.gpsCharge = gpsCharge;
}
public Ptsv2paymentsTravelInformationAutoRental phoneCharge(String phoneCharge) {
this.phoneCharge = phoneCharge;
return this;
}
/**
* Additional charges incurred for phone usage included on the total bill.
* @return phoneCharge
**/
@ApiModelProperty(value = "Additional charges incurred for phone usage included on the total bill. ")
public String getPhoneCharge() {
return phoneCharge;
}
public void setPhoneCharge(String phoneCharge) {
this.phoneCharge = phoneCharge;
}
public Ptsv2paymentsTravelInformationAutoRental parkingViolationCharge(String parkingViolationCharge) {
this.parkingViolationCharge = parkingViolationCharge;
return this;
}
/**
* Extra charges incurred due to a parking violation for the auto. This field is supported only for Visa.
* @return parkingViolationCharge
**/
@ApiModelProperty(value = "Extra charges incurred due to a parking violation for the auto. This field is supported only for Visa. ")
public String getParkingViolationCharge() {
return parkingViolationCharge;
}
public void setParkingViolationCharge(String parkingViolationCharge) {
this.parkingViolationCharge = parkingViolationCharge;
}
public Ptsv2paymentsTravelInformationAutoRental otherCharges(String otherCharges) {
this.otherCharges = otherCharges;
return this;
}
/**
* Total amount charged for all other miscellaneous charges not previously defined.
* @return otherCharges
**/
@ApiModelProperty(value = "Total amount charged for all other miscellaneous charges not previously defined. ")
public String getOtherCharges() {
return otherCharges;
}
public void setOtherCharges(String otherCharges) {
this.otherCharges = otherCharges;
}
public Ptsv2paymentsTravelInformationAutoRental companyName(String companyName) {
this.companyName = companyName;
return this;
}
/**
* Merchant to send their auto rental company name
* @return companyName
**/
@ApiModelProperty(value = "Merchant to send their auto rental company name ")
public String getCompanyName() {
return companyName;
}
public void setCompanyName(String companyName) {
this.companyName = companyName;
}
public Ptsv2paymentsTravelInformationAutoRental affiliateName(String affiliateName) {
this.affiliateName = affiliateName;
return this;
}
/**
* When merchant wants to send the affiliate name.
* @return affiliateName
**/
@ApiModelProperty(value = "When merchant wants to send the affiliate name. ")
public String getAffiliateName() {
return affiliateName;
}
public void setAffiliateName(String affiliateName) {
this.affiliateName = affiliateName;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Ptsv2paymentsTravelInformationAutoRental ptsv2paymentsTravelInformationAutoRental = (Ptsv2paymentsTravelInformationAutoRental) o;
return Objects.equals(this.noShowIndicator, ptsv2paymentsTravelInformationAutoRental.noShowIndicator) &&
Objects.equals(this.customerName, ptsv2paymentsTravelInformationAutoRental.customerName) &&
Objects.equals(this.vehicleClass, ptsv2paymentsTravelInformationAutoRental.vehicleClass) &&
Objects.equals(this.distanceTravelled, ptsv2paymentsTravelInformationAutoRental.distanceTravelled) &&
Objects.equals(this.distanceUnit, ptsv2paymentsTravelInformationAutoRental.distanceUnit) &&
Objects.equals(this.returnDateTime, ptsv2paymentsTravelInformationAutoRental.returnDateTime) &&
Objects.equals(this.rentalDateTime, ptsv2paymentsTravelInformationAutoRental.rentalDateTime) &&
Objects.equals(this.maxFreeDistance, ptsv2paymentsTravelInformationAutoRental.maxFreeDistance) &&
Objects.equals(this.insuranceIndicator, ptsv2paymentsTravelInformationAutoRental.insuranceIndicator) &&
Objects.equals(this.programCode, ptsv2paymentsTravelInformationAutoRental.programCode) &&
Objects.equals(this.returnAddress, ptsv2paymentsTravelInformationAutoRental.returnAddress) &&
Objects.equals(this.rentalAddress, ptsv2paymentsTravelInformationAutoRental.rentalAddress) &&
Objects.equals(this.agreementNumber, ptsv2paymentsTravelInformationAutoRental.agreementNumber) &&
Objects.equals(this.odometerReading, ptsv2paymentsTravelInformationAutoRental.odometerReading) &&
Objects.equals(this.vehicleIdentificationNumber, ptsv2paymentsTravelInformationAutoRental.vehicleIdentificationNumber) &&
Objects.equals(this.companyId, ptsv2paymentsTravelInformationAutoRental.companyId) &&
Objects.equals(this.numberOfAdditionalDrivers, ptsv2paymentsTravelInformationAutoRental.numberOfAdditionalDrivers) &&
Objects.equals(this.driverAge, ptsv2paymentsTravelInformationAutoRental.driverAge) &&
Objects.equals(this.specialProgramCode, ptsv2paymentsTravelInformationAutoRental.specialProgramCode) &&
Objects.equals(this.vehicleMake, ptsv2paymentsTravelInformationAutoRental.vehicleMake) &&
Objects.equals(this.vehicleModel, ptsv2paymentsTravelInformationAutoRental.vehicleModel) &&
Objects.equals(this.timePeriod, ptsv2paymentsTravelInformationAutoRental.timePeriod) &&
Objects.equals(this.commodityCode, ptsv2paymentsTravelInformationAutoRental.commodityCode) &&
Objects.equals(this.customerServicePhoneNumber, ptsv2paymentsTravelInformationAutoRental.customerServicePhoneNumber) &&
Objects.equals(this.taxDetails, ptsv2paymentsTravelInformationAutoRental.taxDetails) &&
Objects.equals(this.insuranceAmount, ptsv2paymentsTravelInformationAutoRental.insuranceAmount) &&
Objects.equals(this.oneWayDropOffAmount, ptsv2paymentsTravelInformationAutoRental.oneWayDropOffAmount) &&
Objects.equals(this.adjustedAmountIndicator, ptsv2paymentsTravelInformationAutoRental.adjustedAmountIndicator) &&
Objects.equals(this.adjustedAmount, ptsv2paymentsTravelInformationAutoRental.adjustedAmount) &&
Objects.equals(this.fuelCharges, ptsv2paymentsTravelInformationAutoRental.fuelCharges) &&
Objects.equals(this.weeklyRentalRate, ptsv2paymentsTravelInformationAutoRental.weeklyRentalRate) &&
Objects.equals(this.dailyRentalRate, ptsv2paymentsTravelInformationAutoRental.dailyRentalRate) &&
Objects.equals(this.ratePerMile, ptsv2paymentsTravelInformationAutoRental.ratePerMile) &&
Objects.equals(this.mileageCharge, ptsv2paymentsTravelInformationAutoRental.mileageCharge) &&
Objects.equals(this.extraMileageCharge, ptsv2paymentsTravelInformationAutoRental.extraMileageCharge) &&
Objects.equals(this.lateFeeAmount, ptsv2paymentsTravelInformationAutoRental.lateFeeAmount) &&
Objects.equals(this.towingCharge, ptsv2paymentsTravelInformationAutoRental.towingCharge) &&
Objects.equals(this.extraCharge, ptsv2paymentsTravelInformationAutoRental.extraCharge) &&
Objects.equals(this.gpsCharge, ptsv2paymentsTravelInformationAutoRental.gpsCharge) &&
Objects.equals(this.phoneCharge, ptsv2paymentsTravelInformationAutoRental.phoneCharge) &&
Objects.equals(this.parkingViolationCharge, ptsv2paymentsTravelInformationAutoRental.parkingViolationCharge) &&
Objects.equals(this.otherCharges, ptsv2paymentsTravelInformationAutoRental.otherCharges) &&
Objects.equals(this.companyName, ptsv2paymentsTravelInformationAutoRental.companyName) &&
Objects.equals(this.affiliateName, ptsv2paymentsTravelInformationAutoRental.affiliateName);
}
@Override
public int hashCode() {
return Objects.hash(noShowIndicator, customerName, vehicleClass, distanceTravelled, distanceUnit, returnDateTime, rentalDateTime, maxFreeDistance, insuranceIndicator, programCode, returnAddress, rentalAddress, agreementNumber, odometerReading, vehicleIdentificationNumber, companyId, numberOfAdditionalDrivers, driverAge, specialProgramCode, vehicleMake, vehicleModel, timePeriod, commodityCode, customerServicePhoneNumber, taxDetails, insuranceAmount, oneWayDropOffAmount, adjustedAmountIndicator, adjustedAmount, fuelCharges, weeklyRentalRate, dailyRentalRate, ratePerMile, mileageCharge, extraMileageCharge, lateFeeAmount, towingCharge, extraCharge, gpsCharge, phoneCharge, parkingViolationCharge, otherCharges, companyName, affiliateName);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Ptsv2paymentsTravelInformationAutoRental {\n");
sb.append(" noShowIndicator: ").append(toIndentedString(noShowIndicator)).append("\n");
sb.append(" customerName: ").append(toIndentedString(customerName)).append("\n");
sb.append(" vehicleClass: ").append(toIndentedString(vehicleClass)).append("\n");
sb.append(" distanceTravelled: ").append(toIndentedString(distanceTravelled)).append("\n");
sb.append(" distanceUnit: ").append(toIndentedString(distanceUnit)).append("\n");
sb.append(" returnDateTime: ").append(toIndentedString(returnDateTime)).append("\n");
sb.append(" rentalDateTime: ").append(toIndentedString(rentalDateTime)).append("\n");
sb.append(" maxFreeDistance: ").append(toIndentedString(maxFreeDistance)).append("\n");
sb.append(" insuranceIndicator: ").append(toIndentedString(insuranceIndicator)).append("\n");
sb.append(" programCode: ").append(toIndentedString(programCode)).append("\n");
sb.append(" returnAddress: ").append(toIndentedString(returnAddress)).append("\n");
sb.append(" rentalAddress: ").append(toIndentedString(rentalAddress)).append("\n");
sb.append(" agreementNumber: ").append(toIndentedString(agreementNumber)).append("\n");
sb.append(" odometerReading: ").append(toIndentedString(odometerReading)).append("\n");
sb.append(" vehicleIdentificationNumber: ").append(toIndentedString(vehicleIdentificationNumber)).append("\n");
sb.append(" companyId: ").append(toIndentedString(companyId)).append("\n");
sb.append(" numberOfAdditionalDrivers: ").append(toIndentedString(numberOfAdditionalDrivers)).append("\n");
sb.append(" driverAge: ").append(toIndentedString(driverAge)).append("\n");
sb.append(" specialProgramCode: ").append(toIndentedString(specialProgramCode)).append("\n");
sb.append(" vehicleMake: ").append(toIndentedString(vehicleMake)).append("\n");
sb.append(" vehicleModel: ").append(toIndentedString(vehicleModel)).append("\n");
sb.append(" timePeriod: ").append(toIndentedString(timePeriod)).append("\n");
sb.append(" commodityCode: ").append(toIndentedString(commodityCode)).append("\n");
sb.append(" customerServicePhoneNumber: ").append(toIndentedString(customerServicePhoneNumber)).append("\n");
sb.append(" taxDetails: ").append(toIndentedString(taxDetails)).append("\n");
sb.append(" insuranceAmount: ").append(toIndentedString(insuranceAmount)).append("\n");
sb.append(" oneWayDropOffAmount: ").append(toIndentedString(oneWayDropOffAmount)).append("\n");
sb.append(" adjustedAmountIndicator: ").append(toIndentedString(adjustedAmountIndicator)).append("\n");
sb.append(" adjustedAmount: ").append(toIndentedString(adjustedAmount)).append("\n");
sb.append(" fuelCharges: ").append(toIndentedString(fuelCharges)).append("\n");
sb.append(" weeklyRentalRate: ").append(toIndentedString(weeklyRentalRate)).append("\n");
sb.append(" dailyRentalRate: ").append(toIndentedString(dailyRentalRate)).append("\n");
sb.append(" ratePerMile: ").append(toIndentedString(ratePerMile)).append("\n");
sb.append(" mileageCharge: ").append(toIndentedString(mileageCharge)).append("\n");
sb.append(" extraMileageCharge: ").append(toIndentedString(extraMileageCharge)).append("\n");
sb.append(" lateFeeAmount: ").append(toIndentedString(lateFeeAmount)).append("\n");
sb.append(" towingCharge: ").append(toIndentedString(towingCharge)).append("\n");
sb.append(" extraCharge: ").append(toIndentedString(extraCharge)).append("\n");
sb.append(" gpsCharge: ").append(toIndentedString(gpsCharge)).append("\n");
sb.append(" phoneCharge: ").append(toIndentedString(phoneCharge)).append("\n");
sb.append(" parkingViolationCharge: ").append(toIndentedString(parkingViolationCharge)).append("\n");
sb.append(" otherCharges: ").append(toIndentedString(otherCharges)).append("\n");
sb.append(" companyName: ").append(toIndentedString(companyName)).append("\n");
sb.append(" affiliateName: ").append(toIndentedString(affiliateName)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy