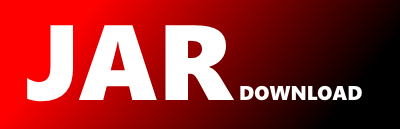
Model.Ptsv2paymentsidreversalsProcessingInformation Maven / Gradle / Ivy
/*
* CyberSource Merged Spec
* All CyberSource API specs merged together. These are available at https://developer.cybersource.com/api/reference/api-reference.html
*
* OpenAPI spec version: 0.0.1
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package Model;
import java.util.Objects;
import java.util.Arrays;
import Model.Ptsv2paymentsIssuerInformation;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
/**
* Ptsv2paymentsidreversalsProcessingInformation
*/
public class Ptsv2paymentsidreversalsProcessingInformation {
@SerializedName("paymentSolution")
private String paymentSolution = null;
@SerializedName("reconciliationId")
private String reconciliationId = null;
@SerializedName("linkId")
private String linkId = null;
@SerializedName("reportGroup")
private String reportGroup = null;
@SerializedName("visaCheckoutId")
private String visaCheckoutId = null;
@SerializedName("issuer")
private Ptsv2paymentsIssuerInformation issuer = null;
@SerializedName("actionList")
private List actionList = null;
public Ptsv2paymentsidreversalsProcessingInformation paymentSolution(String paymentSolution) {
this.paymentSolution = paymentSolution;
return this;
}
/**
* Type of digital payment solution for the transaction. Possible Values: - `visacheckout`: Visa Checkout. This value is required for Visa Checkout transactions. For details, see `payment_solution` field description in [Visa Checkout Using the REST API.](https://developer.cybersource.com/content/dam/docs/cybs/en-us/apifields/reference/all/rest/api-fields.pdf) - `001`: Apple Pay. - `004`: Cybersource In-App Solution. - `005`: Masterpass. This value is required for Masterpass transactions on OmniPay Direct. - `006`: Android Pay. - `007`: Chase Pay. - `008`: Samsung Pay. - `012`: Google Pay. - `013`: Cybersource P2PE Decryption - `014`: Mastercard credential on file (COF) payment network token. Returned in authorizations that use a payment network token associated with a TMS token. - `015`: Visa credential on file (COF) payment network token. Returned in authorizations that use a payment network token associated with a TMS token. - `027`: Click to Pay.
* @return paymentSolution
**/
@ApiModelProperty(value = "Type of digital payment solution for the transaction. Possible Values: - `visacheckout`: Visa Checkout. This value is required for Visa Checkout transactions. For details, see `payment_solution` field description in [Visa Checkout Using the REST API.](https://developer.cybersource.com/content/dam/docs/cybs/en-us/apifields/reference/all/rest/api-fields.pdf) - `001`: Apple Pay. - `004`: Cybersource In-App Solution. - `005`: Masterpass. This value is required for Masterpass transactions on OmniPay Direct. - `006`: Android Pay. - `007`: Chase Pay. - `008`: Samsung Pay. - `012`: Google Pay. - `013`: Cybersource P2PE Decryption - `014`: Mastercard credential on file (COF) payment network token. Returned in authorizations that use a payment network token associated with a TMS token. - `015`: Visa credential on file (COF) payment network token. Returned in authorizations that use a payment network token associated with a TMS token. - `027`: Click to Pay. ")
public String getPaymentSolution() {
return paymentSolution;
}
public void setPaymentSolution(String paymentSolution) {
this.paymentSolution = paymentSolution;
}
public Ptsv2paymentsidreversalsProcessingInformation reconciliationId(String reconciliationId) {
this.reconciliationId = reconciliationId;
return this;
}
/**
* Please check with Cybersource customer support to see if your merchant account is configured correctly so you can include this field in your request. * For Payouts: max length for FDCCompass is String (22).
* @return reconciliationId
**/
@ApiModelProperty(value = "Please check with Cybersource customer support to see if your merchant account is configured correctly so you can include this field in your request. * For Payouts: max length for FDCCompass is String (22). ")
public String getReconciliationId() {
return reconciliationId;
}
public void setReconciliationId(String reconciliationId) {
this.reconciliationId = reconciliationId;
}
public Ptsv2paymentsidreversalsProcessingInformation linkId(String linkId) {
this.linkId = linkId;
return this;
}
/**
* Value that links the current authorization request to the original authorization request. Set this value to the ID that was returned in the reply message from the original authorization request. This value is used for: - Partial authorizations - Split shipments
* @return linkId
**/
@ApiModelProperty(value = "Value that links the current authorization request to the original authorization request. Set this value to the ID that was returned in the reply message from the original authorization request. This value is used for: - Partial authorizations - Split shipments ")
public String getLinkId() {
return linkId;
}
public void setLinkId(String linkId) {
this.linkId = linkId;
}
public Ptsv2paymentsidreversalsProcessingInformation reportGroup(String reportGroup) {
this.reportGroup = reportGroup;
return this;
}
/**
* Attribute that lets you define custom grouping for your processor reports. This field is supported only for **Worldpay VAP**.
* @return reportGroup
**/
@ApiModelProperty(value = "Attribute that lets you define custom grouping for your processor reports. This field is supported only for **Worldpay VAP**. ")
public String getReportGroup() {
return reportGroup;
}
public void setReportGroup(String reportGroup) {
this.reportGroup = reportGroup;
}
public Ptsv2paymentsidreversalsProcessingInformation visaCheckoutId(String visaCheckoutId) {
this.visaCheckoutId = visaCheckoutId;
return this;
}
/**
* Identifier for the **Visa Checkout** order. Visa Checkout provides a unique order ID for every transaction in the Visa Checkout **callID** field.
* @return visaCheckoutId
**/
@ApiModelProperty(value = "Identifier for the **Visa Checkout** order. Visa Checkout provides a unique order ID for every transaction in the Visa Checkout **callID** field. ")
public String getVisaCheckoutId() {
return visaCheckoutId;
}
public void setVisaCheckoutId(String visaCheckoutId) {
this.visaCheckoutId = visaCheckoutId;
}
public Ptsv2paymentsidreversalsProcessingInformation issuer(Ptsv2paymentsIssuerInformation issuer) {
this.issuer = issuer;
return this;
}
/**
* Get issuer
* @return issuer
**/
@ApiModelProperty(value = "")
public Ptsv2paymentsIssuerInformation getIssuer() {
return issuer;
}
public void setIssuer(Ptsv2paymentsIssuerInformation issuer) {
this.issuer = issuer;
}
public Ptsv2paymentsidreversalsProcessingInformation actionList(List actionList) {
this.actionList = actionList;
return this;
}
public Ptsv2paymentsidreversalsProcessingInformation addActionListItem(String actionListItem) {
if (this.actionList == null) {
this.actionList = new ArrayList();
}
this.actionList.add(actionListItem);
return this;
}
/**
* Array of actions (one or more) to be included in the reversal Possible value: - `AP_AUTH_REVERSAL`: Use this when you want to reverse an Alternative Payment Authorization.
* @return actionList
**/
@ApiModelProperty(value = "Array of actions (one or more) to be included in the reversal Possible value: - `AP_AUTH_REVERSAL`: Use this when you want to reverse an Alternative Payment Authorization. ")
public List getActionList() {
return actionList;
}
public void setActionList(List actionList) {
this.actionList = actionList;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Ptsv2paymentsidreversalsProcessingInformation ptsv2paymentsidreversalsProcessingInformation = (Ptsv2paymentsidreversalsProcessingInformation) o;
return Objects.equals(this.paymentSolution, ptsv2paymentsidreversalsProcessingInformation.paymentSolution) &&
Objects.equals(this.reconciliationId, ptsv2paymentsidreversalsProcessingInformation.reconciliationId) &&
Objects.equals(this.linkId, ptsv2paymentsidreversalsProcessingInformation.linkId) &&
Objects.equals(this.reportGroup, ptsv2paymentsidreversalsProcessingInformation.reportGroup) &&
Objects.equals(this.visaCheckoutId, ptsv2paymentsidreversalsProcessingInformation.visaCheckoutId) &&
Objects.equals(this.issuer, ptsv2paymentsidreversalsProcessingInformation.issuer) &&
Objects.equals(this.actionList, ptsv2paymentsidreversalsProcessingInformation.actionList);
}
@Override
public int hashCode() {
return Objects.hash(paymentSolution, reconciliationId, linkId, reportGroup, visaCheckoutId, issuer, actionList);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Ptsv2paymentsidreversalsProcessingInformation {\n");
sb.append(" paymentSolution: ").append(toIndentedString(paymentSolution)).append("\n");
sb.append(" reconciliationId: ").append(toIndentedString(reconciliationId)).append("\n");
sb.append(" linkId: ").append(toIndentedString(linkId)).append("\n");
sb.append(" reportGroup: ").append(toIndentedString(reportGroup)).append("\n");
sb.append(" visaCheckoutId: ").append(toIndentedString(visaCheckoutId)).append("\n");
sb.append(" issuer: ").append(toIndentedString(issuer)).append("\n");
sb.append(" actionList: ").append(toIndentedString(actionList)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy