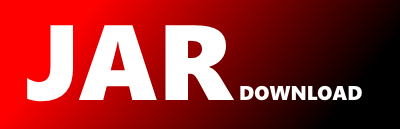
Model.Riskv1decisionsOrderInformation Maven / Gradle / Ivy
/*
* CyberSource Merged Spec
* All CyberSource API specs merged together. These are available at https://developer.cybersource.com/api/reference/api-reference.html
*
* OpenAPI spec version: 0.0.1
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package Model;
import java.util.Objects;
import java.util.Arrays;
import Model.Riskv1decisionsOrderInformationAmountDetails;
import Model.Riskv1decisionsOrderInformationBillTo;
import Model.Riskv1decisionsOrderInformationLineItems;
import Model.Riskv1decisionsOrderInformationShipTo;
import Model.Riskv1decisionsOrderInformationShippingDetails;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
/**
* Contains detailed order-level information.
*/
@ApiModel(description = "Contains detailed order-level information.")
public class Riskv1decisionsOrderInformation {
@SerializedName("amountDetails")
private Riskv1decisionsOrderInformationAmountDetails amountDetails = null;
@SerializedName("preOrder")
private String preOrder = null;
@SerializedName("preOrderDate")
private String preOrderDate = null;
@SerializedName("cutoffDateTime")
private String cutoffDateTime = null;
@SerializedName("reordered")
private Boolean reordered = null;
@SerializedName("shippingDetails")
private Riskv1decisionsOrderInformationShippingDetails shippingDetails = null;
@SerializedName("shipTo")
private Riskv1decisionsOrderInformationShipTo shipTo = null;
@SerializedName("returnsAccepted")
private Boolean returnsAccepted = null;
@SerializedName("lineItems")
private List lineItems = null;
@SerializedName("billTo")
private Riskv1decisionsOrderInformationBillTo billTo = null;
@SerializedName("totalOffersCount")
private String totalOffersCount = null;
public Riskv1decisionsOrderInformation amountDetails(Riskv1decisionsOrderInformationAmountDetails amountDetails) {
this.amountDetails = amountDetails;
return this;
}
/**
* Get amountDetails
* @return amountDetails
**/
@ApiModelProperty(value = "")
public Riskv1decisionsOrderInformationAmountDetails getAmountDetails() {
return amountDetails;
}
public void setAmountDetails(Riskv1decisionsOrderInformationAmountDetails amountDetails) {
this.amountDetails = amountDetails;
}
public Riskv1decisionsOrderInformation preOrder(String preOrder) {
this.preOrder = preOrder;
return this;
}
/**
* Indicates whether cardholder is placing an order with a future availability or release date. This field can contain one of these values: - MERCHANDISE_AVAILABLE: Merchandise available - FUTURE_AVAILABILITY: Future availability
* @return preOrder
**/
@ApiModelProperty(value = "Indicates whether cardholder is placing an order with a future availability or release date. This field can contain one of these values: - MERCHANDISE_AVAILABLE: Merchandise available - FUTURE_AVAILABILITY: Future availability ")
public String getPreOrder() {
return preOrder;
}
public void setPreOrder(String preOrder) {
this.preOrder = preOrder;
}
public Riskv1decisionsOrderInformation preOrderDate(String preOrderDate) {
this.preOrderDate = preOrderDate;
return this;
}
/**
* Expected date that a pre-ordered purchase will be available. Format: YYYYMMDD
* @return preOrderDate
**/
@ApiModelProperty(value = "Expected date that a pre-ordered purchase will be available. Format: YYYYMMDD ")
public String getPreOrderDate() {
return preOrderDate;
}
public void setPreOrderDate(String preOrderDate) {
this.preOrderDate = preOrderDate;
}
public Riskv1decisionsOrderInformation cutoffDateTime(String cutoffDateTime) {
this.cutoffDateTime = cutoffDateTime;
return this;
}
/**
* Starting date and time for an event or a journey that is independent of which transportation mechanism, in UTC. The cutoffDateTime will supersede travelInformation.departureTime if both are supplied in the request. Format: YYYY-MM-DDThh:mm:ssZ. Example 2016-08-11T22:47:57Z equals August 11, 2016, at 22:47:57 (10:47:57 p.m.). The T separates the date and the time. The Z indicates UTC.
* @return cutoffDateTime
**/
@ApiModelProperty(value = "Starting date and time for an event or a journey that is independent of which transportation mechanism, in UTC. The cutoffDateTime will supersede travelInformation.departureTime if both are supplied in the request. Format: YYYY-MM-DDThh:mm:ssZ. Example 2016-08-11T22:47:57Z equals August 11, 2016, at 22:47:57 (10:47:57 p.m.). The T separates the date and the time. The Z indicates UTC. ")
public String getCutoffDateTime() {
return cutoffDateTime;
}
public void setCutoffDateTime(String cutoffDateTime) {
this.cutoffDateTime = cutoffDateTime;
}
public Riskv1decisionsOrderInformation reordered(Boolean reordered) {
this.reordered = reordered;
return this;
}
/**
* Indicates whether the cardholder is reordering previously purchased merchandise. This field can contain one of these values: - false: First time ordered - true: Reordered
* @return reordered
**/
@ApiModelProperty(value = "Indicates whether the cardholder is reordering previously purchased merchandise. This field can contain one of these values: - false: First time ordered - true: Reordered ")
public Boolean isReordered() {
return reordered;
}
public void setReordered(Boolean reordered) {
this.reordered = reordered;
}
public Riskv1decisionsOrderInformation shippingDetails(Riskv1decisionsOrderInformationShippingDetails shippingDetails) {
this.shippingDetails = shippingDetails;
return this;
}
/**
* Get shippingDetails
* @return shippingDetails
**/
@ApiModelProperty(value = "")
public Riskv1decisionsOrderInformationShippingDetails getShippingDetails() {
return shippingDetails;
}
public void setShippingDetails(Riskv1decisionsOrderInformationShippingDetails shippingDetails) {
this.shippingDetails = shippingDetails;
}
public Riskv1decisionsOrderInformation shipTo(Riskv1decisionsOrderInformationShipTo shipTo) {
this.shipTo = shipTo;
return this;
}
/**
* Get shipTo
* @return shipTo
**/
@ApiModelProperty(value = "")
public Riskv1decisionsOrderInformationShipTo getShipTo() {
return shipTo;
}
public void setShipTo(Riskv1decisionsOrderInformationShipTo shipTo) {
this.shipTo = shipTo;
}
public Riskv1decisionsOrderInformation returnsAccepted(Boolean returnsAccepted) {
this.returnsAccepted = returnsAccepted;
return this;
}
/**
* Boolean that indicates whether returns are accepted for this order. This field can contain one of the following values: - true: Returns are accepted for this order. - false: Returns are not accepted for this order.
* @return returnsAccepted
**/
@ApiModelProperty(value = "Boolean that indicates whether returns are accepted for this order. This field can contain one of the following values: - true: Returns are accepted for this order. - false: Returns are not accepted for this order. ")
public Boolean isReturnsAccepted() {
return returnsAccepted;
}
public void setReturnsAccepted(Boolean returnsAccepted) {
this.returnsAccepted = returnsAccepted;
}
public Riskv1decisionsOrderInformation lineItems(List lineItems) {
this.lineItems = lineItems;
return this;
}
public Riskv1decisionsOrderInformation addLineItemsItem(Riskv1decisionsOrderInformationLineItems lineItemsItem) {
if (this.lineItems == null) {
this.lineItems = new ArrayList();
}
this.lineItems.add(lineItemsItem);
return this;
}
/**
* This array contains detailed information about individual products in the order.
* @return lineItems
**/
@ApiModelProperty(value = "This array contains detailed information about individual products in the order.")
public List getLineItems() {
return lineItems;
}
public void setLineItems(List lineItems) {
this.lineItems = lineItems;
}
public Riskv1decisionsOrderInformation billTo(Riskv1decisionsOrderInformationBillTo billTo) {
this.billTo = billTo;
return this;
}
/**
* Get billTo
* @return billTo
**/
@ApiModelProperty(value = "")
public Riskv1decisionsOrderInformationBillTo getBillTo() {
return billTo;
}
public void setBillTo(Riskv1decisionsOrderInformationBillTo billTo) {
this.billTo = billTo;
}
public Riskv1decisionsOrderInformation totalOffersCount(String totalOffersCount) {
this.totalOffersCount = totalOffersCount;
return this;
}
/**
* Total number of articles/items in the order as a numeric decimal count. Possible values: 00 - 99
* @return totalOffersCount
**/
@ApiModelProperty(value = "Total number of articles/items in the order as a numeric decimal count. Possible values: 00 - 99 ")
public String getTotalOffersCount() {
return totalOffersCount;
}
public void setTotalOffersCount(String totalOffersCount) {
this.totalOffersCount = totalOffersCount;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Riskv1decisionsOrderInformation riskv1decisionsOrderInformation = (Riskv1decisionsOrderInformation) o;
return Objects.equals(this.amountDetails, riskv1decisionsOrderInformation.amountDetails) &&
Objects.equals(this.preOrder, riskv1decisionsOrderInformation.preOrder) &&
Objects.equals(this.preOrderDate, riskv1decisionsOrderInformation.preOrderDate) &&
Objects.equals(this.cutoffDateTime, riskv1decisionsOrderInformation.cutoffDateTime) &&
Objects.equals(this.reordered, riskv1decisionsOrderInformation.reordered) &&
Objects.equals(this.shippingDetails, riskv1decisionsOrderInformation.shippingDetails) &&
Objects.equals(this.shipTo, riskv1decisionsOrderInformation.shipTo) &&
Objects.equals(this.returnsAccepted, riskv1decisionsOrderInformation.returnsAccepted) &&
Objects.equals(this.lineItems, riskv1decisionsOrderInformation.lineItems) &&
Objects.equals(this.billTo, riskv1decisionsOrderInformation.billTo) &&
Objects.equals(this.totalOffersCount, riskv1decisionsOrderInformation.totalOffersCount);
}
@Override
public int hashCode() {
return Objects.hash(amountDetails, preOrder, preOrderDate, cutoffDateTime, reordered, shippingDetails, shipTo, returnsAccepted, lineItems, billTo, totalOffersCount);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Riskv1decisionsOrderInformation {\n");
sb.append(" amountDetails: ").append(toIndentedString(amountDetails)).append("\n");
sb.append(" preOrder: ").append(toIndentedString(preOrder)).append("\n");
sb.append(" preOrderDate: ").append(toIndentedString(preOrderDate)).append("\n");
sb.append(" cutoffDateTime: ").append(toIndentedString(cutoffDateTime)).append("\n");
sb.append(" reordered: ").append(toIndentedString(reordered)).append("\n");
sb.append(" shippingDetails: ").append(toIndentedString(shippingDetails)).append("\n");
sb.append(" shipTo: ").append(toIndentedString(shipTo)).append("\n");
sb.append(" returnsAccepted: ").append(toIndentedString(returnsAccepted)).append("\n");
sb.append(" lineItems: ").append(toIndentedString(lineItems)).append("\n");
sb.append(" billTo: ").append(toIndentedString(billTo)).append("\n");
sb.append(" totalOffersCount: ").append(toIndentedString(totalOffersCount)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy