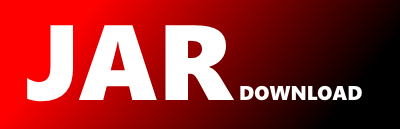
Api.PlansApi Maven / Gradle / Ivy
/*
* CyberSource Merged Spec
* All CyberSource API specs merged together. These are available at https://developer.cybersource.com/api/reference/api-reference.html
*
* OpenAPI spec version: 0.0.1
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package Api;
import Invokers.ApiCallback;
import Invokers.ApiClient;
import Invokers.ApiException;
import Invokers.ApiResponse;
import Invokers.Configuration;
import Invokers.Pair;
import Invokers.ProgressRequestBody;
import Invokers.ProgressResponseBody;
import com.google.gson.reflect.TypeToken;
import java.io.IOException;
import java.io.InputStream;
import Model.ActivateDeactivatePlanResponse;
import Model.CreatePlanRequest;
import Model.CreatePlanResponse;
import Model.DeletePlanResponse;
import Model.GetAllPlansResponse;
import Model.GetPlanCodeResponse;
import Model.GetPlanResponse;
import Model.InlineResponse4003;
import Model.InlineResponse404;
import Model.PtsV2PaymentsPost502Response;
import Model.UpdatePlanRequest;
import Model.UpdatePlanResponse;
import java.lang.reflect.Type;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
import utilities.tracking.SdkTracker;
public class PlansApi {
private static Logger logger = LogManager.getLogger(PlansApi.class);
private ApiClient apiClient;
public PlansApi() {
this(Configuration.getDefaultApiClient());
}
public PlansApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
public ApiClient getApiClient() {
return apiClient;
}
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* Build call for activatePlan
* @param id Plan Id (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public okhttp3.Call activatePlanCall(String id, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
SdkTracker sdkTracker = new SdkTracker();
Object localVarPostBody = null;
if ("POST".equalsIgnoreCase("POST")) {
localVarPostBody = "{}";
}
// create path and map variables
String localVarPath = "/rbs/v1/plans/{id}/activate"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json", "application/hal+json", "application/json;charset=utf-8", "application/hal+json;charset=utf-8"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json;charset=utf-8"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().newBuilder().addNetworkInterceptor(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call activatePlanValidateBeforeCall(String id, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'id' is set
if (id == null) {
logger.error("Missing the required parameter 'id' when calling activatePlan(Async)");
throw new ApiException("Missing the required parameter 'id' when calling activatePlan(Async)");
}
okhttp3.Call call = activatePlanCall(id, progressListener, progressRequestListener);
return call;
}
/**
* Activate a Plan
* Activate a Plan
* @param id Plan Id (required)
* @return ActivateDeactivatePlanResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ActivateDeactivatePlanResponse activatePlan(String id) throws ApiException {
logger.info("CALL TO METHOD 'activatePlan' STARTED");
this.apiClient.setComputationStartTime(System.nanoTime());
ApiResponse resp = activatePlanWithHttpInfo(id);
logger.info("CALL TO METHOD 'activatePlan' ENDED");
return resp.getData();
}
/**
* Activate a Plan
* Activate a Plan
* @param id Plan Id (required)
* @return ApiResponse<ActivateDeactivatePlanResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse activatePlanWithHttpInfo(String id) throws ApiException {
okhttp3.Call call = activatePlanValidateBeforeCall(id, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Activate a Plan (asynchronously)
* Activate a Plan
* @param id Plan Id (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public okhttp3.Call activatePlanAsync(String id, final ApiCallback callback) throws ApiException {
this.apiClient.setComputationStartTime(System.nanoTime());
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
okhttp3.Call call = activatePlanValidateBeforeCall(id, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for createPlan
* @param createPlanRequest (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public okhttp3.Call createPlanCall(CreatePlanRequest createPlanRequest, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
SdkTracker sdkTracker = new SdkTracker();
Object localVarPostBody = sdkTracker.insertDeveloperIdTracker(createPlanRequest, CreatePlanRequest.class.getSimpleName(), apiClient.merchantConfig.getRunEnvironment(), apiClient.merchantConfig.getDefaultDeveloperId());
// create path and map variables
String localVarPath = "/rbs/v1/plans";
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json", "application/hal+json", "application/json;charset=utf-8", "application/hal+json;charset=utf-8"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json;charset=utf-8"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().newBuilder().addNetworkInterceptor(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call createPlanValidateBeforeCall(CreatePlanRequest createPlanRequest, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'createPlanRequest' is set
if (createPlanRequest == null) {
logger.error("Missing the required parameter 'createPlanRequest' when calling createPlan(Async)");
throw new ApiException("Missing the required parameter 'createPlanRequest' when calling createPlan(Async)");
}
okhttp3.Call call = createPlanCall(createPlanRequest, progressListener, progressRequestListener);
return call;
}
/**
* Create a Plan
* The recurring billing service enables you to manage payment plans and subscriptions for recurring payment schedules. It securely stores your customer's payment information and personal data within secure Visa data centers, reducing storage risks and PCI DSS scope through the use of *Token Management* (*TMS*). The three key elements of *Cybersource* Recurring Billing are: - **Token**: stores customer billing, shipping, and payment details. - **Plan**: stores the billing schedule. - **Subscription**: combines the token and plan, and defines the subscription start date, name, and description. The APIs in this section demonstrate the management of the Plans and Subscriptions. For Tokens please refer to [Token Management](#token-management)
* @param createPlanRequest (required)
* @return CreatePlanResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public CreatePlanResponse createPlan(CreatePlanRequest createPlanRequest) throws ApiException {
logger.info("CALL TO METHOD 'createPlan' STARTED");
this.apiClient.setComputationStartTime(System.nanoTime());
ApiResponse resp = createPlanWithHttpInfo(createPlanRequest);
logger.info("CALL TO METHOD 'createPlan' ENDED");
return resp.getData();
}
/**
* Create a Plan
* The recurring billing service enables you to manage payment plans and subscriptions for recurring payment schedules. It securely stores your customer's payment information and personal data within secure Visa data centers, reducing storage risks and PCI DSS scope through the use of *Token Management* (*TMS*). The three key elements of *Cybersource* Recurring Billing are: - **Token**: stores customer billing, shipping, and payment details. - **Plan**: stores the billing schedule. - **Subscription**: combines the token and plan, and defines the subscription start date, name, and description. The APIs in this section demonstrate the management of the Plans and Subscriptions. For Tokens please refer to [Token Management](#token-management)
* @param createPlanRequest (required)
* @return ApiResponse<CreatePlanResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse createPlanWithHttpInfo(CreatePlanRequest createPlanRequest) throws ApiException {
okhttp3.Call call = createPlanValidateBeforeCall(createPlanRequest, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Create a Plan (asynchronously)
* The recurring billing service enables you to manage payment plans and subscriptions for recurring payment schedules. It securely stores your customer's payment information and personal data within secure Visa data centers, reducing storage risks and PCI DSS scope through the use of *Token Management* (*TMS*). The three key elements of *Cybersource* Recurring Billing are: - **Token**: stores customer billing, shipping, and payment details. - **Plan**: stores the billing schedule. - **Subscription**: combines the token and plan, and defines the subscription start date, name, and description. The APIs in this section demonstrate the management of the Plans and Subscriptions. For Tokens please refer to [Token Management](#token-management)
* @param createPlanRequest (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public okhttp3.Call createPlanAsync(CreatePlanRequest createPlanRequest, final ApiCallback callback) throws ApiException {
this.apiClient.setComputationStartTime(System.nanoTime());
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
okhttp3.Call call = createPlanValidateBeforeCall(createPlanRequest, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for deactivatePlan
* @param id Plan Id (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public okhttp3.Call deactivatePlanCall(String id, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
SdkTracker sdkTracker = new SdkTracker();
Object localVarPostBody = null;
if ("POST".equalsIgnoreCase("POST")) {
localVarPostBody = "{}";
}
// create path and map variables
String localVarPath = "/rbs/v1/plans/{id}/deactivate"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json", "application/hal+json", "application/json;charset=utf-8", "application/hal+json;charset=utf-8"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json;charset=utf-8"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().newBuilder().addNetworkInterceptor(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call deactivatePlanValidateBeforeCall(String id, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'id' is set
if (id == null) {
logger.error("Missing the required parameter 'id' when calling deactivatePlan(Async)");
throw new ApiException("Missing the required parameter 'id' when calling deactivatePlan(Async)");
}
okhttp3.Call call = deactivatePlanCall(id, progressListener, progressRequestListener);
return call;
}
/**
* Deactivate a Plan
* Deactivate a Plan
* @param id Plan Id (required)
* @return ActivateDeactivatePlanResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ActivateDeactivatePlanResponse deactivatePlan(String id) throws ApiException {
logger.info("CALL TO METHOD 'deactivatePlan' STARTED");
this.apiClient.setComputationStartTime(System.nanoTime());
ApiResponse resp = deactivatePlanWithHttpInfo(id);
logger.info("CALL TO METHOD 'deactivatePlan' ENDED");
return resp.getData();
}
/**
* Deactivate a Plan
* Deactivate a Plan
* @param id Plan Id (required)
* @return ApiResponse<ActivateDeactivatePlanResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse deactivatePlanWithHttpInfo(String id) throws ApiException {
okhttp3.Call call = deactivatePlanValidateBeforeCall(id, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Deactivate a Plan (asynchronously)
* Deactivate a Plan
* @param id Plan Id (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public okhttp3.Call deactivatePlanAsync(String id, final ApiCallback callback) throws ApiException {
this.apiClient.setComputationStartTime(System.nanoTime());
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
okhttp3.Call call = deactivatePlanValidateBeforeCall(id, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for deletePlan
* @param id Plan Id (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public okhttp3.Call deletePlanCall(String id, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
SdkTracker sdkTracker = new SdkTracker();
Object localVarPostBody = null;
if ("DELETE".equalsIgnoreCase("POST")) {
localVarPostBody = "{}";
}
// create path and map variables
String localVarPath = "/rbs/v1/plans/{id}"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json", "application/hal+json", "application/json;charset=utf-8", "application/hal+json;charset=utf-8"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json;charset=utf-8"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().newBuilder().addNetworkInterceptor(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "DELETE", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call deletePlanValidateBeforeCall(String id, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'id' is set
if (id == null) {
logger.error("Missing the required parameter 'id' when calling deletePlan(Async)");
throw new ApiException("Missing the required parameter 'id' when calling deletePlan(Async)");
}
okhttp3.Call call = deletePlanCall(id, progressListener, progressRequestListener);
return call;
}
/**
* Delete a Plan
* Delete a Plan is only allowed: - plan status is in `DRAFT` - plan status is in `ACTIVE`, and `INACTIVE` only allowed when no subscriptions attached to a plan in the lifetime of a plan
* @param id Plan Id (required)
* @return DeletePlanResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public DeletePlanResponse deletePlan(String id) throws ApiException {
logger.info("CALL TO METHOD 'deletePlan' STARTED");
this.apiClient.setComputationStartTime(System.nanoTime());
ApiResponse resp = deletePlanWithHttpInfo(id);
logger.info("CALL TO METHOD 'deletePlan' ENDED");
return resp.getData();
}
/**
* Delete a Plan
* Delete a Plan is only allowed: - plan status is in `DRAFT` - plan status is in `ACTIVE`, and `INACTIVE` only allowed when no subscriptions attached to a plan in the lifetime of a plan
* @param id Plan Id (required)
* @return ApiResponse<DeletePlanResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse deletePlanWithHttpInfo(String id) throws ApiException {
okhttp3.Call call = deletePlanValidateBeforeCall(id, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Delete a Plan (asynchronously)
* Delete a Plan is only allowed: - plan status is in `DRAFT` - plan status is in `ACTIVE`, and `INACTIVE` only allowed when no subscriptions attached to a plan in the lifetime of a plan
* @param id Plan Id (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public okhttp3.Call deletePlanAsync(String id, final ApiCallback callback) throws ApiException {
this.apiClient.setComputationStartTime(System.nanoTime());
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
okhttp3.Call call = deletePlanValidateBeforeCall(id, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for getPlan
* @param id Plan Id (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public okhttp3.Call getPlanCall(String id, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
SdkTracker sdkTracker = new SdkTracker();
Object localVarPostBody = null;
if ("GET".equalsIgnoreCase("POST")) {
localVarPostBody = "{}";
}
// create path and map variables
String localVarPath = "/rbs/v1/plans/{id}"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json", "application/hal+json", "application/json;charset=utf-8", "application/hal+json;charset=utf-8"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json;charset=utf-8"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().newBuilder().addNetworkInterceptor(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call getPlanValidateBeforeCall(String id, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'id' is set
if (id == null) {
logger.error("Missing the required parameter 'id' when calling getPlan(Async)");
throw new ApiException("Missing the required parameter 'id' when calling getPlan(Async)");
}
okhttp3.Call call = getPlanCall(id, progressListener, progressRequestListener);
return call;
}
/**
* Get a Plan
* Retrieve a Plan details by Plan Id.
* @param id Plan Id (required)
* @return GetPlanResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public GetPlanResponse getPlan(String id) throws ApiException {
logger.info("CALL TO METHOD 'getPlan' STARTED");
this.apiClient.setComputationStartTime(System.nanoTime());
ApiResponse resp = getPlanWithHttpInfo(id);
logger.info("CALL TO METHOD 'getPlan' ENDED");
return resp.getData();
}
/**
* Get a Plan
* Retrieve a Plan details by Plan Id.
* @param id Plan Id (required)
* @return ApiResponse<GetPlanResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getPlanWithHttpInfo(String id) throws ApiException {
okhttp3.Call call = getPlanValidateBeforeCall(id, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get a Plan (asynchronously)
* Retrieve a Plan details by Plan Id.
* @param id Plan Id (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public okhttp3.Call getPlanAsync(String id, final ApiCallback callback) throws ApiException {
this.apiClient.setComputationStartTime(System.nanoTime());
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
okhttp3.Call call = getPlanValidateBeforeCall(id, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for getPlanCode
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public okhttp3.Call getPlanCodeCall(final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
SdkTracker sdkTracker = new SdkTracker();
Object localVarPostBody = null;
if ("GET".equalsIgnoreCase("POST")) {
localVarPostBody = "{}";
}
// create path and map variables
String localVarPath = "/rbs/v1/plans/code";
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json", "application/hal+json", "application/json;charset=utf-8", "application/hal+json;charset=utf-8"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json;charset=utf-8"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().newBuilder().addNetworkInterceptor(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call getPlanCodeValidateBeforeCall(final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
okhttp3.Call call = getPlanCodeCall(progressListener, progressRequestListener);
return call;
}
/**
* Get a Plan Code
* Get a Unique Plan Code
* @return GetPlanCodeResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public GetPlanCodeResponse getPlanCode() throws ApiException {
logger.info("CALL TO METHOD 'getPlanCode' STARTED");
this.apiClient.setComputationStartTime(System.nanoTime());
ApiResponse resp = getPlanCodeWithHttpInfo();
logger.info("CALL TO METHOD 'getPlanCode' ENDED");
return resp.getData();
}
/**
* Get a Plan Code
* Get a Unique Plan Code
* @return ApiResponse<GetPlanCodeResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getPlanCodeWithHttpInfo() throws ApiException {
okhttp3.Call call = getPlanCodeValidateBeforeCall(null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get a Plan Code (asynchronously)
* Get a Unique Plan Code
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public okhttp3.Call getPlanCodeAsync(final ApiCallback callback) throws ApiException {
this.apiClient.setComputationStartTime(System.nanoTime());
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
okhttp3.Call call = getPlanCodeValidateBeforeCall(progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for getPlans
* @param offset Page offset number. (optional)
* @param limit Number of items to be returned. Default - `20`, Max - `100` (optional)
* @param code Filter by Plan Code (optional)
* @param status Filter by Plan Status (optional)
* @param name Filter by Plan Name. (First sub string or full string) **[Not Recommended]** (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public okhttp3.Call getPlansCall(Integer offset, Integer limit, String code, String status, String name, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
SdkTracker sdkTracker = new SdkTracker();
Object localVarPostBody = null;
if ("GET".equalsIgnoreCase("POST")) {
localVarPostBody = "{}";
}
// create path and map variables
String localVarPath = "/rbs/v1/plans";
List localVarQueryParams = new ArrayList();
if (offset != null)
localVarQueryParams.addAll(apiClient.parameterToPairs("", "offset", offset));
if (limit != null)
localVarQueryParams.addAll(apiClient.parameterToPairs("", "limit", limit));
if (code != null)
localVarQueryParams.addAll(apiClient.parameterToPairs("", "code", code));
if (status != null)
localVarQueryParams.addAll(apiClient.parameterToPairs("", "status", status));
if (name != null)
localVarQueryParams.addAll(apiClient.parameterToPairs("", "name", name));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json", "application/hal+json", "application/json;charset=utf-8", "application/hal+json;charset=utf-8"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json;charset=utf-8"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().newBuilder().addNetworkInterceptor(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call getPlansValidateBeforeCall(Integer offset, Integer limit, String code, String status, String name, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
okhttp3.Call call = getPlansCall(offset, limit, code, status, name, progressListener, progressRequestListener);
return call;
}
/**
* Get a List of Plans
* Retrieve Plans by Plan Code & Plan Status.
* @param offset Page offset number. (optional)
* @param limit Number of items to be returned. Default - `20`, Max - `100` (optional)
* @param code Filter by Plan Code (optional)
* @param status Filter by Plan Status (optional)
* @param name Filter by Plan Name. (First sub string or full string) **[Not Recommended]** (optional)
* @return GetAllPlansResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public GetAllPlansResponse getPlans(Integer offset, Integer limit, String code, String status, String name) throws ApiException {
logger.info("CALL TO METHOD 'getPlans' STARTED");
this.apiClient.setComputationStartTime(System.nanoTime());
ApiResponse resp = getPlansWithHttpInfo(offset, limit, code, status, name);
logger.info("CALL TO METHOD 'getPlans' ENDED");
return resp.getData();
}
/**
* Get a List of Plans
* Retrieve Plans by Plan Code & Plan Status.
* @param offset Page offset number. (optional)
* @param limit Number of items to be returned. Default - `20`, Max - `100` (optional)
* @param code Filter by Plan Code (optional)
* @param status Filter by Plan Status (optional)
* @param name Filter by Plan Name. (First sub string or full string) **[Not Recommended]** (optional)
* @return ApiResponse<GetAllPlansResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getPlansWithHttpInfo(Integer offset, Integer limit, String code, String status, String name) throws ApiException {
okhttp3.Call call = getPlansValidateBeforeCall(offset, limit, code, status, name, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get a List of Plans (asynchronously)
* Retrieve Plans by Plan Code & Plan Status.
* @param offset Page offset number. (optional)
* @param limit Number of items to be returned. Default - `20`, Max - `100` (optional)
* @param code Filter by Plan Code (optional)
* @param status Filter by Plan Status (optional)
* @param name Filter by Plan Name. (First sub string or full string) **[Not Recommended]** (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public okhttp3.Call getPlansAsync(Integer offset, Integer limit, String code, String status, String name, final ApiCallback callback) throws ApiException {
this.apiClient.setComputationStartTime(System.nanoTime());
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
okhttp3.Call call = getPlansValidateBeforeCall(offset, limit, code, status, name, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for updatePlan
* @param id Plan Id (required)
* @param updatePlanRequest (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public okhttp3.Call updatePlanCall(String id, UpdatePlanRequest updatePlanRequest, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
SdkTracker sdkTracker = new SdkTracker();
Object localVarPostBody = sdkTracker.insertDeveloperIdTracker(updatePlanRequest, UpdatePlanRequest.class.getSimpleName(), apiClient.merchantConfig.getRunEnvironment(), apiClient.merchantConfig.getDefaultDeveloperId());
// create path and map variables
String localVarPath = "/rbs/v1/plans/{id}"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json", "application/hal+json", "application/json;charset=utf-8", "application/hal+json;charset=utf-8"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json;charset=utf-8"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().newBuilder().addNetworkInterceptor(new okhttp3.Interceptor() {
@Override
public okhttp3.Response intercept(okhttp3.Interceptor.Chain chain) throws IOException {
okhttp3.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "PATCH", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call updatePlanValidateBeforeCall(String id, UpdatePlanRequest updatePlanRequest, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'id' is set
if (id == null) {
logger.error("Missing the required parameter 'id' when calling updatePlan(Async)");
throw new ApiException("Missing the required parameter 'id' when calling updatePlan(Async)");
}
// verify the required parameter 'updatePlanRequest' is set
if (updatePlanRequest == null) {
logger.error("Missing the required parameter 'updatePlanRequest' when calling updatePlan(Async)");
throw new ApiException("Missing the required parameter 'updatePlanRequest' when calling updatePlan(Async)");
}
okhttp3.Call call = updatePlanCall(id, updatePlanRequest, progressListener, progressRequestListener);
return call;
}
/**
* Update a Plan
* Update a Plan Plan in `DRAFT` status - All updates are allowed on Plan with `DRAFT` status Plan in `ACTIVE` status [Following fields are **Not Updatable**] - `planInformation.billingPeriod` - `planInformation.billingCycles` [Update is only allowed to **increase** billingCycles] - `orderInformation.amountDetails.currency`
* @param id Plan Id (required)
* @param updatePlanRequest (required)
* @return UpdatePlanResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public UpdatePlanResponse updatePlan(String id, UpdatePlanRequest updatePlanRequest) throws ApiException {
logger.info("CALL TO METHOD 'updatePlan' STARTED");
this.apiClient.setComputationStartTime(System.nanoTime());
ApiResponse resp = updatePlanWithHttpInfo(id, updatePlanRequest);
logger.info("CALL TO METHOD 'updatePlan' ENDED");
return resp.getData();
}
/**
* Update a Plan
* Update a Plan Plan in `DRAFT` status - All updates are allowed on Plan with `DRAFT` status Plan in `ACTIVE` status [Following fields are **Not Updatable**] - `planInformation.billingPeriod` - `planInformation.billingCycles` [Update is only allowed to **increase** billingCycles] - `orderInformation.amountDetails.currency`
* @param id Plan Id (required)
* @param updatePlanRequest (required)
* @return ApiResponse<UpdatePlanResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse updatePlanWithHttpInfo(String id, UpdatePlanRequest updatePlanRequest) throws ApiException {
okhttp3.Call call = updatePlanValidateBeforeCall(id, updatePlanRequest, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Update a Plan (asynchronously)
* Update a Plan Plan in `DRAFT` status - All updates are allowed on Plan with `DRAFT` status Plan in `ACTIVE` status [Following fields are **Not Updatable**] - `planInformation.billingPeriod` - `planInformation.billingCycles` [Update is only allowed to **increase** billingCycles] - `orderInformation.amountDetails.currency`
* @param id Plan Id (required)
* @param updatePlanRequest (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public okhttp3.Call updatePlanAsync(String id, UpdatePlanRequest updatePlanRequest, final ApiCallback callback) throws ApiException {
this.apiClient.setComputationStartTime(System.nanoTime());
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
okhttp3.Call call = updatePlanValidateBeforeCall(id, updatePlanRequest, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy