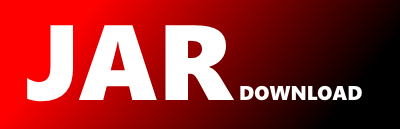
Model.Binv1binlookupProcessingInformationPayoutOptions Maven / Gradle / Ivy
/*
* CyberSource Merged Spec
* All CyberSource API specs merged together. These are available at https://developer.cybersource.com/api/reference/api-reference.html
*
* OpenAPI spec version: 0.0.1
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package Model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
/**
* Payout fields request parameters
*/
@ApiModel(description = "Payout fields request parameters ")
public class Binv1binlookupProcessingInformationPayoutOptions {
@SerializedName("payoutInquiry")
private Boolean payoutInquiry = null;
@SerializedName("networkId")
private String networkId = null;
@SerializedName("acquirerBin")
private String acquirerBin = null;
public Binv1binlookupProcessingInformationPayoutOptions payoutInquiry(Boolean payoutInquiry) {
this.payoutInquiry = payoutInquiry;
return this;
}
/**
* If `true` then provide attributes related to fund transfer/payouts. If payout information not found then response will have standard account lookup. Possible values: - true - false
* @return payoutInquiry
**/
@ApiModelProperty(value = "If `true` then provide attributes related to fund transfer/payouts. If payout information not found then response will have standard account lookup. Possible values: - true - false ")
public Boolean isPayoutInquiry() {
return payoutInquiry;
}
public void setPayoutInquiry(Boolean payoutInquiry) {
this.payoutInquiry = payoutInquiry;
}
public Binv1binlookupProcessingInformationPayoutOptions networkId(String networkId) {
this.networkId = networkId;
return this;
}
/**
* The networks specified in this field must be a subset of the information provided during program enrollment Possible values: - 0020 : Accel/Exchange - 0024 : CU24 - 0003 : Interlink - 0016 : Maestro - 0018 : NYCE - 0027 : NYCE - 0009 : Pulse - 0017 : Pulse - 0019 : Pulse - 0008 : Star - 0010 : Star - 0011 : Star - 0012 : Star - 0015 : Star - 0002 : Visa/PLUS
* @return networkId
**/
@ApiModelProperty(value = "The networks specified in this field must be a subset of the information provided during program enrollment Possible values: - 0020 : Accel/Exchange - 0024 : CU24 - 0003 : Interlink - 0016 : Maestro - 0018 : NYCE - 0027 : NYCE - 0009 : Pulse - 0017 : Pulse - 0019 : Pulse - 0008 : Star - 0010 : Star - 0011 : Star - 0012 : Star - 0015 : Star - 0002 : Visa/PLUS ")
public String getNetworkId() {
return networkId;
}
public void setNetworkId(String networkId) {
this.networkId = networkId;
}
public Binv1binlookupProcessingInformationPayoutOptions acquirerBin(String acquirerBin) {
this.acquirerBin = acquirerBin;
return this;
}
/**
* BIN under which the Funds Transfer application is registered. This must match the information provided during enrollment.
* @return acquirerBin
**/
@ApiModelProperty(value = "BIN under which the Funds Transfer application is registered. This must match the information provided during enrollment. ")
public String getAcquirerBin() {
return acquirerBin;
}
public void setAcquirerBin(String acquirerBin) {
this.acquirerBin = acquirerBin;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Binv1binlookupProcessingInformationPayoutOptions binv1binlookupProcessingInformationPayoutOptions = (Binv1binlookupProcessingInformationPayoutOptions) o;
return Objects.equals(this.payoutInquiry, binv1binlookupProcessingInformationPayoutOptions.payoutInquiry) &&
Objects.equals(this.networkId, binv1binlookupProcessingInformationPayoutOptions.networkId) &&
Objects.equals(this.acquirerBin, binv1binlookupProcessingInformationPayoutOptions.acquirerBin);
}
@Override
public int hashCode() {
return Objects.hash(payoutInquiry, networkId, acquirerBin);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Binv1binlookupProcessingInformationPayoutOptions {\n");
sb.append(" payoutInquiry: ").append(toIndentedString(payoutInquiry)).append("\n");
sb.append(" networkId: ").append(toIndentedString(networkId)).append("\n");
sb.append(" acquirerBin: ").append(toIndentedString(acquirerBin)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy