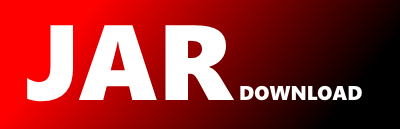
Model.CardProcessingConfigFeaturesCardNotPresent Maven / Gradle / Ivy
/*
* CyberSource Merged Spec
* All CyberSource API specs merged together. These are available at https://developer.cybersource.com/api/reference/api-reference.html
*
* OpenAPI spec version: 0.0.1
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package Model;
import java.util.Objects;
import java.util.Arrays;
import Model.CardProcessingConfigFeaturesCardNotPresentInstallment;
import Model.CardProcessingConfigFeaturesCardNotPresentProcessors;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* CardProcessingConfigFeaturesCardNotPresent
*/
public class CardProcessingConfigFeaturesCardNotPresent {
@SerializedName("processors")
private Map processors = null;
@SerializedName("ignoreAddressVerificationSystem")
private Boolean ignoreAddressVerificationSystem = null;
@SerializedName("visaStraightThroughProcessingOnly")
private Boolean visaStraightThroughProcessingOnly = null;
@SerializedName("amexTransactionAdviceAddendum1")
private String amexTransactionAdviceAddendum1 = null;
@SerializedName("installment")
private CardProcessingConfigFeaturesCardNotPresentInstallment installment = null;
public CardProcessingConfigFeaturesCardNotPresent processors(Map processors) {
this.processors = processors;
return this;
}
public CardProcessingConfigFeaturesCardNotPresent putProcessorsItem(String key, CardProcessingConfigFeaturesCardNotPresentProcessors processorsItem) {
if (this.processors == null) {
this.processors = new HashMap();
}
this.processors.put(key, processorsItem);
return this;
}
/**
* e.g. * amexdirect * barclays2 * CUP * EFTPOS * fdiglobal * gpx * smartfdc * tsys * vero * VPC For VPC, CUP and EFTPOS processors, replace the processor name from VPC or CUP or EFTPOS to the actual processor name in the sample request. e.g. replace VPC with <your vpc processor>
* @return processors
**/
@ApiModelProperty(value = "e.g. * amexdirect * barclays2 * CUP * EFTPOS * fdiglobal * gpx * smartfdc * tsys * vero * VPC For VPC, CUP and EFTPOS processors, replace the processor name from VPC or CUP or EFTPOS to the actual processor name in the sample request. e.g. replace VPC with <your vpc processor> ")
public Map getProcessors() {
return processors;
}
public void setProcessors(Map processors) {
this.processors = processors;
}
public CardProcessingConfigFeaturesCardNotPresent ignoreAddressVerificationSystem(Boolean ignoreAddressVerificationSystem) {
this.ignoreAddressVerificationSystem = ignoreAddressVerificationSystem;
return this;
}
/**
* Flag for a sale request that indicates whether to allow the capture service to run even when the authorization receives an AVS decline. Applicable for VPC, FDI Global (fdiglobal), GPX (gpx) and GPN (gpn) processors.
* @return ignoreAddressVerificationSystem
**/
@ApiModelProperty(value = "Flag for a sale request that indicates whether to allow the capture service to run even when the authorization receives an AVS decline. Applicable for VPC, FDI Global (fdiglobal), GPX (gpx) and GPN (gpn) processors.")
public Boolean isIgnoreAddressVerificationSystem() {
return ignoreAddressVerificationSystem;
}
public void setIgnoreAddressVerificationSystem(Boolean ignoreAddressVerificationSystem) {
this.ignoreAddressVerificationSystem = ignoreAddressVerificationSystem;
}
public CardProcessingConfigFeaturesCardNotPresent visaStraightThroughProcessingOnly(Boolean visaStraightThroughProcessingOnly) {
this.visaStraightThroughProcessingOnly = visaStraightThroughProcessingOnly;
return this;
}
/**
* Indicates if a merchant is enabled for Straight Through Processing - B2B invoice payments. Applicable for FDI Global (fdiglobal), TSYS (tsys), VPC and GPX (gpx) processors.
* @return visaStraightThroughProcessingOnly
**/
@ApiModelProperty(value = "Indicates if a merchant is enabled for Straight Through Processing - B2B invoice payments. Applicable for FDI Global (fdiglobal), TSYS (tsys), VPC and GPX (gpx) processors.")
public Boolean isVisaStraightThroughProcessingOnly() {
return visaStraightThroughProcessingOnly;
}
public void setVisaStraightThroughProcessingOnly(Boolean visaStraightThroughProcessingOnly) {
this.visaStraightThroughProcessingOnly = visaStraightThroughProcessingOnly;
}
public CardProcessingConfigFeaturesCardNotPresent amexTransactionAdviceAddendum1(String amexTransactionAdviceAddendum1) {
this.amexTransactionAdviceAddendum1 = amexTransactionAdviceAddendum1;
return this;
}
/**
* Advice addendum field. It is used to display descriptive information about a transaction on customer's American Express card statement. Applicable for TSYS (tsys), FDI Global (fdiglobal) and American Express Direct (amexdirect) processors.
* @return amexTransactionAdviceAddendum1
**/
@ApiModelProperty(value = "Advice addendum field. It is used to display descriptive information about a transaction on customer's American Express card statement. Applicable for TSYS (tsys), FDI Global (fdiglobal) and American Express Direct (amexdirect) processors.")
public String getAmexTransactionAdviceAddendum1() {
return amexTransactionAdviceAddendum1;
}
public void setAmexTransactionAdviceAddendum1(String amexTransactionAdviceAddendum1) {
this.amexTransactionAdviceAddendum1 = amexTransactionAdviceAddendum1;
}
public CardProcessingConfigFeaturesCardNotPresent installment(CardProcessingConfigFeaturesCardNotPresentInstallment installment) {
this.installment = installment;
return this;
}
/**
* Get installment
* @return installment
**/
@ApiModelProperty(value = "")
public CardProcessingConfigFeaturesCardNotPresentInstallment getInstallment() {
return installment;
}
public void setInstallment(CardProcessingConfigFeaturesCardNotPresentInstallment installment) {
this.installment = installment;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
CardProcessingConfigFeaturesCardNotPresent cardProcessingConfigFeaturesCardNotPresent = (CardProcessingConfigFeaturesCardNotPresent) o;
return Objects.equals(this.processors, cardProcessingConfigFeaturesCardNotPresent.processors) &&
Objects.equals(this.ignoreAddressVerificationSystem, cardProcessingConfigFeaturesCardNotPresent.ignoreAddressVerificationSystem) &&
Objects.equals(this.visaStraightThroughProcessingOnly, cardProcessingConfigFeaturesCardNotPresent.visaStraightThroughProcessingOnly) &&
Objects.equals(this.amexTransactionAdviceAddendum1, cardProcessingConfigFeaturesCardNotPresent.amexTransactionAdviceAddendum1) &&
Objects.equals(this.installment, cardProcessingConfigFeaturesCardNotPresent.installment);
}
@Override
public int hashCode() {
return Objects.hash(processors, ignoreAddressVerificationSystem, visaStraightThroughProcessingOnly, amexTransactionAdviceAddendum1, installment);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class CardProcessingConfigFeaturesCardNotPresent {\n");
sb.append(" processors: ").append(toIndentedString(processors)).append("\n");
sb.append(" ignoreAddressVerificationSystem: ").append(toIndentedString(ignoreAddressVerificationSystem)).append("\n");
sb.append(" visaStraightThroughProcessingOnly: ").append(toIndentedString(visaStraightThroughProcessingOnly)).append("\n");
sb.append(" amexTransactionAdviceAddendum1: ").append(toIndentedString(amexTransactionAdviceAddendum1)).append("\n");
sb.append(" installment: ").append(toIndentedString(installment)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy