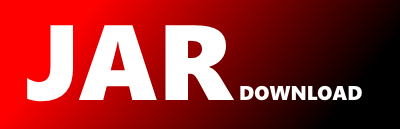
Model.CreatePlanResponse Maven / Gradle / Ivy
/*
* CyberSource Merged Spec
* All CyberSource API specs merged together. These are available at https://developer.cybersource.com/api/reference/api-reference.html
*
* OpenAPI spec version: 0.0.1
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package Model;
import java.util.Objects;
import java.util.Arrays;
import Model.CreatePlanResponsePlanInformation;
import Model.PtsV2IncrementalAuthorizationPatch201ResponseLinks;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
/**
* CreatePlanResponse
*/
public class CreatePlanResponse {
@SerializedName("_links")
private PtsV2IncrementalAuthorizationPatch201ResponseLinks links = null;
@SerializedName("id")
private String id = null;
@SerializedName("submitTimeUtc")
private String submitTimeUtc = null;
@SerializedName("status")
private String status = null;
@SerializedName("planInformation")
private CreatePlanResponsePlanInformation planInformation = null;
public CreatePlanResponse links(PtsV2IncrementalAuthorizationPatch201ResponseLinks links) {
this.links = links;
return this;
}
/**
* Get links
* @return links
**/
@ApiModelProperty(value = "")
public PtsV2IncrementalAuthorizationPatch201ResponseLinks getLinks() {
return links;
}
public void setLinks(PtsV2IncrementalAuthorizationPatch201ResponseLinks links) {
this.links = links;
}
public CreatePlanResponse id(String id) {
this.id = id;
return this;
}
/**
* An unique identification number generated by Cybersource to identify the submitted request. Returned by all services. It is also appended to the endpoint of the resource. On incremental authorizations, this value with be the same as the identification number returned in the original authorization response.
* @return id
**/
@ApiModelProperty(value = "An unique identification number generated by Cybersource to identify the submitted request. Returned by all services. It is also appended to the endpoint of the resource. On incremental authorizations, this value with be the same as the identification number returned in the original authorization response. ")
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public CreatePlanResponse submitTimeUtc(String submitTimeUtc) {
this.submitTimeUtc = submitTimeUtc;
return this;
}
/**
* Time of request in UTC. Format: `YYYY-MM-DDThh:mm:ssZ` **Example** `2016-08-11T22:47:57Z` equals August 11, 2016, at 22:47:57 (10:47:57 p.m.). The `T` separates the date and the time. The `Z` indicates UTC. Returned by Cybersource for all services.
* @return submitTimeUtc
**/
@ApiModelProperty(value = "Time of request in UTC. Format: `YYYY-MM-DDThh:mm:ssZ` **Example** `2016-08-11T22:47:57Z` equals August 11, 2016, at 22:47:57 (10:47:57 p.m.). The `T` separates the date and the time. The `Z` indicates UTC. Returned by Cybersource for all services. ")
public String getSubmitTimeUtc() {
return submitTimeUtc;
}
public void setSubmitTimeUtc(String submitTimeUtc) {
this.submitTimeUtc = submitTimeUtc;
}
public CreatePlanResponse status(String status) {
this.status = status;
return this;
}
/**
* The status of the submitted transaction. Possible values: - COMPLETED
* @return status
**/
@ApiModelProperty(value = "The status of the submitted transaction. Possible values: - COMPLETED ")
public String getStatus() {
return status;
}
public void setStatus(String status) {
this.status = status;
}
public CreatePlanResponse planInformation(CreatePlanResponsePlanInformation planInformation) {
this.planInformation = planInformation;
return this;
}
/**
* Get planInformation
* @return planInformation
**/
@ApiModelProperty(value = "")
public CreatePlanResponsePlanInformation getPlanInformation() {
return planInformation;
}
public void setPlanInformation(CreatePlanResponsePlanInformation planInformation) {
this.planInformation = planInformation;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
CreatePlanResponse createPlanResponse = (CreatePlanResponse) o;
return Objects.equals(this.links, createPlanResponse.links) &&
Objects.equals(this.id, createPlanResponse.id) &&
Objects.equals(this.submitTimeUtc, createPlanResponse.submitTimeUtc) &&
Objects.equals(this.status, createPlanResponse.status) &&
Objects.equals(this.planInformation, createPlanResponse.planInformation);
}
@Override
public int hashCode() {
return Objects.hash(links, id, submitTimeUtc, status, planInformation);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class CreatePlanResponse {\n");
sb.append(" links: ").append(toIndentedString(links)).append("\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" submitTimeUtc: ").append(toIndentedString(submitTimeUtc)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" planInformation: ").append(toIndentedString(planInformation)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy