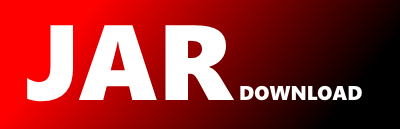
Model.CreateWebhookRequest Maven / Gradle / Ivy
/*
* CyberSource Merged Spec
* All CyberSource API specs merged together. These are available at https://developer.cybersource.com/api/reference/api-reference.html
*
* OpenAPI spec version: 0.0.1
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package Model;
import java.util.Objects;
import java.util.Arrays;
import Model.Notificationsubscriptionsv1webhooksRetryPolicy;
import Model.Notificationsubscriptionsv1webhooksSecurityPolicy1;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
/**
* CreateWebhookRequest
*/
public class CreateWebhookRequest {
@SerializedName("name")
private String name = null;
@SerializedName("description")
private String description = null;
@SerializedName("organizationId")
private String organizationId = null;
@SerializedName("productId")
private String productId = null;
@SerializedName("eventTypes")
private List eventTypes = null;
@SerializedName("webhookUrl")
private String webhookUrl = null;
@SerializedName("healthCheckUrl")
private String healthCheckUrl = null;
@SerializedName("notificationScope")
private String notificationScope = null;
@SerializedName("retryPolicy")
private Notificationsubscriptionsv1webhooksRetryPolicy retryPolicy = null;
@SerializedName("securityPolicy")
private Notificationsubscriptionsv1webhooksSecurityPolicy1 securityPolicy = null;
public CreateWebhookRequest name(String name) {
this.name = name;
return this;
}
/**
* Client friendly webhook name.
* @return name
**/
@ApiModelProperty(value = "Client friendly webhook name.")
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public CreateWebhookRequest description(String description) {
this.description = description;
return this;
}
/**
* Client friendly webhook description.
* @return description
**/
@ApiModelProperty(value = "Client friendly webhook description.")
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public CreateWebhookRequest organizationId(String organizationId) {
this.organizationId = organizationId;
return this;
}
/**
* Organization Identifier (OrgId) or Merchant Identifier (MID).
* @return organizationId
**/
@ApiModelProperty(value = "Organization Identifier (OrgId) or Merchant Identifier (MID).")
public String getOrganizationId() {
return organizationId;
}
public void setOrganizationId(String organizationId) {
this.organizationId = organizationId;
}
public CreateWebhookRequest productId(String productId) {
this.productId = productId;
return this;
}
/**
* To see the valid productId and eventTypes, call the \"Create and Manage Webhooks - Retrieve a list of event types\" endpoint.
* @return productId
**/
@ApiModelProperty(value = "To see the valid productId and eventTypes, call the \"Create and Manage Webhooks - Retrieve a list of event types\" endpoint.")
public String getProductId() {
return productId;
}
public void setProductId(String productId) {
this.productId = productId;
}
public CreateWebhookRequest eventTypes(List eventTypes) {
this.eventTypes = eventTypes;
return this;
}
public CreateWebhookRequest addEventTypesItem(String eventTypesItem) {
if (this.eventTypes == null) {
this.eventTypes = new ArrayList();
}
this.eventTypes.add(eventTypesItem);
return this;
}
/**
* Array of the different events for a given product id.
* @return eventTypes
**/
@ApiModelProperty(value = "Array of the different events for a given product id.")
public List getEventTypes() {
return eventTypes;
}
public void setEventTypes(List eventTypes) {
this.eventTypes = eventTypes;
}
public CreateWebhookRequest webhookUrl(String webhookUrl) {
this.webhookUrl = webhookUrl;
return this;
}
/**
* The client's endpoint (URL) to receive webhooks.
* @return webhookUrl
**/
@ApiModelProperty(value = "The client's endpoint (URL) to receive webhooks.")
public String getWebhookUrl() {
return webhookUrl;
}
public void setWebhookUrl(String webhookUrl) {
this.webhookUrl = webhookUrl;
}
public CreateWebhookRequest healthCheckUrl(String healthCheckUrl) {
this.healthCheckUrl = healthCheckUrl;
return this;
}
/**
* The client's health check endpoint (URL). This should be as close as possible to the actual webhookUrl. If the user does not provide the health check URL, it is the user's responsibility to re-activate the webhook if it is deactivated by calling the test endpoint.
* @return healthCheckUrl
**/
@ApiModelProperty(value = "The client's health check endpoint (URL). This should be as close as possible to the actual webhookUrl. If the user does not provide the health check URL, it is the user's responsibility to re-activate the webhook if it is deactivated by calling the test endpoint. ")
public String getHealthCheckUrl() {
return healthCheckUrl;
}
public void setHealthCheckUrl(String healthCheckUrl) {
this.healthCheckUrl = healthCheckUrl;
}
public CreateWebhookRequest notificationScope(String notificationScope) {
this.notificationScope = notificationScope;
return this;
}
/**
* The webhook scope. 1. SELF The Webhook is used to deliver webhooks for only this Organization (or Merchant). 2. DESCENDANTS The Webhook is used to deliver webhooks for this Organization and its children. 3. CUSTOM The Webhook is used to deliver webhooks for the OrgIds (or MiDs) explicitly listed in scopeData field.
* @return notificationScope
**/
@ApiModelProperty(value = "The webhook scope. 1. SELF The Webhook is used to deliver webhooks for only this Organization (or Merchant). 2. DESCENDANTS The Webhook is used to deliver webhooks for this Organization and its children. 3. CUSTOM The Webhook is used to deliver webhooks for the OrgIds (or MiDs) explicitly listed in scopeData field. ")
public String getNotificationScope() {
return notificationScope;
}
public void setNotificationScope(String notificationScope) {
this.notificationScope = notificationScope;
}
public CreateWebhookRequest retryPolicy(Notificationsubscriptionsv1webhooksRetryPolicy retryPolicy) {
this.retryPolicy = retryPolicy;
return this;
}
/**
* Get retryPolicy
* @return retryPolicy
**/
@ApiModelProperty(value = "")
public Notificationsubscriptionsv1webhooksRetryPolicy getRetryPolicy() {
return retryPolicy;
}
public void setRetryPolicy(Notificationsubscriptionsv1webhooksRetryPolicy retryPolicy) {
this.retryPolicy = retryPolicy;
}
public CreateWebhookRequest securityPolicy(Notificationsubscriptionsv1webhooksSecurityPolicy1 securityPolicy) {
this.securityPolicy = securityPolicy;
return this;
}
/**
* Get securityPolicy
* @return securityPolicy
**/
@ApiModelProperty(value = "")
public Notificationsubscriptionsv1webhooksSecurityPolicy1 getSecurityPolicy() {
return securityPolicy;
}
public void setSecurityPolicy(Notificationsubscriptionsv1webhooksSecurityPolicy1 securityPolicy) {
this.securityPolicy = securityPolicy;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
CreateWebhookRequest createWebhookRequest = (CreateWebhookRequest) o;
return Objects.equals(this.name, createWebhookRequest.name) &&
Objects.equals(this.description, createWebhookRequest.description) &&
Objects.equals(this.organizationId, createWebhookRequest.organizationId) &&
Objects.equals(this.productId, createWebhookRequest.productId) &&
Objects.equals(this.eventTypes, createWebhookRequest.eventTypes) &&
Objects.equals(this.webhookUrl, createWebhookRequest.webhookUrl) &&
Objects.equals(this.healthCheckUrl, createWebhookRequest.healthCheckUrl) &&
Objects.equals(this.notificationScope, createWebhookRequest.notificationScope) &&
Objects.equals(this.retryPolicy, createWebhookRequest.retryPolicy) &&
Objects.equals(this.securityPolicy, createWebhookRequest.securityPolicy);
}
@Override
public int hashCode() {
return Objects.hash(name, description, organizationId, productId, eventTypes, webhookUrl, healthCheckUrl, notificationScope, retryPolicy, securityPolicy);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class CreateWebhookRequest {\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" organizationId: ").append(toIndentedString(organizationId)).append("\n");
sb.append(" productId: ").append(toIndentedString(productId)).append("\n");
sb.append(" eventTypes: ").append(toIndentedString(eventTypes)).append("\n");
sb.append(" webhookUrl: ").append(toIndentedString(webhookUrl)).append("\n");
sb.append(" healthCheckUrl: ").append(toIndentedString(healthCheckUrl)).append("\n");
sb.append(" notificationScope: ").append(toIndentedString(notificationScope)).append("\n");
sb.append(" retryPolicy: ").append(toIndentedString(retryPolicy)).append("\n");
sb.append(" securityPolicy: ").append(toIndentedString(securityPolicy)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy