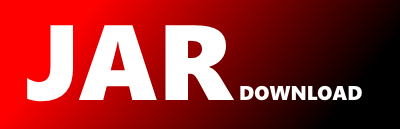
Model.ECheckConfigCommonProcessors Maven / Gradle / Ivy
/*
* CyberSource Merged Spec
* All CyberSource API specs merged together. These are available at https://developer.cybersource.com/api/reference/api-reference.html
*
* OpenAPI spec version: 0.0.1
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package Model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
/**
* Payment Processing connection used to support eCheck, aka ACH, payment methods. Example - \"bofaach\"
*/
@ApiModel(description = "Payment Processing connection used to support eCheck, aka ACH, payment methods. Example - \"bofaach\"")
public class ECheckConfigCommonProcessors {
@SerializedName("companyEntryDescription")
private String companyEntryDescription = null;
@SerializedName("companyId")
private String companyId = null;
@SerializedName("batchGroup")
private String batchGroup = null;
@SerializedName("enableAccuityForAvs")
private Boolean enableAccuityForAvs = true;
@SerializedName("accuityCheckType")
private Object accuityCheckType = null;
@SerializedName("setCompletedState")
private Boolean setCompletedState = false;
public ECheckConfigCommonProcessors companyEntryDescription(String companyEntryDescription) {
this.companyEntryDescription = companyEntryDescription;
return this;
}
/**
* *EXISTING* Company (merchant) defined description of entry to receive. For e.g. PAYROLL, GAS BILL, INS PREM. This field is alphanumeric
* @return companyEntryDescription
**/
@ApiModelProperty(required = true, value = "*EXISTING* Company (merchant) defined description of entry to receive. For e.g. PAYROLL, GAS BILL, INS PREM. This field is alphanumeric")
public String getCompanyEntryDescription() {
return companyEntryDescription;
}
public void setCompanyEntryDescription(String companyEntryDescription) {
this.companyEntryDescription = companyEntryDescription;
}
public ECheckConfigCommonProcessors companyId(String companyId) {
this.companyId = companyId;
return this;
}
/**
* *EXISTING* company ID assigned to merchant by Acquiring bank. This field is alphanumeric
* @return companyId
**/
@ApiModelProperty(value = "*EXISTING* company ID assigned to merchant by Acquiring bank. This field is alphanumeric")
public String getCompanyId() {
return companyId;
}
public void setCompanyId(String companyId) {
this.companyId = companyId;
}
public ECheckConfigCommonProcessors batchGroup(String batchGroup) {
this.batchGroup = batchGroup;
return this;
}
/**
* *EXISTING* Capture requests are grouped into a batch bound for your payment processor. The batch time can be identified by reading the last 2-digits as military time. E.g., <processor>_16 = your processing cutoff is 4PM PST. Please note if you are in a different location you may then need to convert time zone as well.
* @return batchGroup
**/
@ApiModelProperty(value = "*EXISTING* Capture requests are grouped into a batch bound for your payment processor. The batch time can be identified by reading the last 2-digits as military time. E.g., _16 = your processing cutoff is 4PM PST. Please note if you are in a different location you may then need to convert time zone as well.")
public String getBatchGroup() {
return batchGroup;
}
public void setBatchGroup(String batchGroup) {
this.batchGroup = batchGroup;
}
public ECheckConfigCommonProcessors enableAccuityForAvs(Boolean enableAccuityForAvs) {
this.enableAccuityForAvs = enableAccuityForAvs;
return this;
}
/**
* *NEW* Accuity is the original validation service that checks the account/routing number for formatting issues. Used by WF and set to \"Yes\" unless told otherwise
* @return enableAccuityForAvs
**/
@ApiModelProperty(value = "*NEW* Accuity is the original validation service that checks the account/routing number for formatting issues. Used by WF and set to \"Yes\" unless told otherwise")
public Boolean isEnableAccuityForAvs() {
return enableAccuityForAvs;
}
public void setEnableAccuityForAvs(Boolean enableAccuityForAvs) {
this.enableAccuityForAvs = enableAccuityForAvs;
}
public ECheckConfigCommonProcessors accuityCheckType(Object accuityCheckType) {
this.accuityCheckType = accuityCheckType;
return this;
}
/**
* *NEW*
* @return accuityCheckType
**/
@ApiModelProperty(value = "*NEW*")
public Object getAccuityCheckType() {
return accuityCheckType;
}
public void setAccuityCheckType(Object accuityCheckType) {
this.accuityCheckType = accuityCheckType;
}
public ECheckConfigCommonProcessors setCompletedState(Boolean setCompletedState) {
this.setCompletedState = setCompletedState;
return this;
}
/**
* *Moved* When set to Yes we will automatically update transactions to a completed status X-number of days after the transaction comes through; if no failure notification is received. When set to No means we will not update transaction status in this manner. For BAMS/Bank of America merchants, they should be set to No unless we are explicitly asked to set a merchant to YES.
* @return setCompletedState
**/
@ApiModelProperty(value = "*Moved* When set to Yes we will automatically update transactions to a completed status X-number of days after the transaction comes through; if no failure notification is received. When set to No means we will not update transaction status in this manner. For BAMS/Bank of America merchants, they should be set to No unless we are explicitly asked to set a merchant to YES.")
public Boolean isSetCompletedState() {
return setCompletedState;
}
public void setSetCompletedState(Boolean setCompletedState) {
this.setCompletedState = setCompletedState;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ECheckConfigCommonProcessors echeckConfigCommonProcessors = (ECheckConfigCommonProcessors) o;
return Objects.equals(this.companyEntryDescription, echeckConfigCommonProcessors.companyEntryDescription) &&
Objects.equals(this.companyId, echeckConfigCommonProcessors.companyId) &&
Objects.equals(this.batchGroup, echeckConfigCommonProcessors.batchGroup) &&
Objects.equals(this.enableAccuityForAvs, echeckConfigCommonProcessors.enableAccuityForAvs) &&
Objects.equals(this.accuityCheckType, echeckConfigCommonProcessors.accuityCheckType) &&
Objects.equals(this.setCompletedState, echeckConfigCommonProcessors.setCompletedState);
}
@Override
public int hashCode() {
return Objects.hash(companyEntryDescription, companyId, batchGroup, enableAccuityForAvs, accuityCheckType, setCompletedState);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class ECheckConfigCommonProcessors {\n");
sb.append(" companyEntryDescription: ").append(toIndentedString(companyEntryDescription)).append("\n");
sb.append(" companyId: ").append(toIndentedString(companyId)).append("\n");
sb.append(" batchGroup: ").append(toIndentedString(batchGroup)).append("\n");
sb.append(" enableAccuityForAvs: ").append(toIndentedString(enableAccuityForAvs)).append("\n");
sb.append(" accuityCheckType: ").append(toIndentedString(accuityCheckType)).append("\n");
sb.append(" setCompletedState: ").append(toIndentedString(setCompletedState)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy