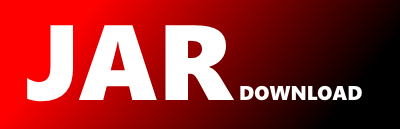
Model.GenerateCaptureContextRequest Maven / Gradle / Ivy
/*
* CyberSource Merged Spec
* All CyberSource API specs merged together. These are available at https://developer.cybersource.com/api/reference/api-reference.html
*
* OpenAPI spec version: 0.0.1
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package Model;
import java.util.Objects;
import java.util.Arrays;
import Model.Microformv2sessionsCheckoutApiInitialization;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
/**
* This is a server-to-server API request to generate the capture context that can be used to initiate instance of microform on a acceptance page. The capture context is a digitally signed JWT that provides authentication, one-time keys, and the target origin to the Microform Integration application.
*/
@ApiModel(description = "This is a server-to-server API request to generate the capture context that can be used to initiate instance of microform on a acceptance page. The capture context is a digitally signed JWT that provides authentication, one-time keys, and the target origin to the Microform Integration application. ")
public class GenerateCaptureContextRequest {
@SerializedName("targetOrigins")
private List targetOrigins = null;
@SerializedName("allowedCardNetworks")
private List allowedCardNetworks = null;
@SerializedName("clientVersion")
private String clientVersion = null;
@SerializedName("checkoutApiInitialization")
private Microformv2sessionsCheckoutApiInitialization checkoutApiInitialization = null;
public GenerateCaptureContextRequest targetOrigins(List targetOrigins) {
this.targetOrigins = targetOrigins;
return this;
}
public GenerateCaptureContextRequest addTargetOriginsItem(String targetOriginsItem) {
if (this.targetOrigins == null) {
this.targetOrigins = new ArrayList();
}
this.targetOrigins.add(targetOriginsItem);
return this;
}
/**
* The [target origin](https://developer.mozilla.org/en-US/docs/Glossary/Origin) of the website on which you will be launching Microform is defined by the scheme (protocol), hostname (domain) and port number (if used). You must use https://hostname (unless you use http://localhost) Wildcards are NOT supported. Ensure that subdomains are included. Any valid top-level domain is supported (e.g. .com, .co.uk, .gov.br etc) Examples: - https://example.com - https://subdomain.example.com - https://example.com:8080<br><br> If you are embedding within multiple nested iframes you need to specify the origins of all the browser contexts used, for example: targetOrigins: [ \"https://example.com\", \"https://basket.example.com\", \"https://ecom.example.com\" ]
* @return targetOrigins
**/
@ApiModelProperty(value = "The [target origin](https://developer.mozilla.org/en-US/docs/Glossary/Origin) of the website on which you will be launching Microform is defined by the scheme (protocol), hostname (domain) and port number (if used). You must use https://hostname (unless you use http://localhost) Wildcards are NOT supported. Ensure that subdomains are included. Any valid top-level domain is supported (e.g. .com, .co.uk, .gov.br etc) Examples: - https://example.com - https://subdomain.example.com - https://example.com:8080
If you are embedding within multiple nested iframes you need to specify the origins of all the browser contexts used, for example: targetOrigins: [ \"https://example.com\", \"https://basket.example.com\", \"https://ecom.example.com\" ] ")
public List getTargetOrigins() {
return targetOrigins;
}
public void setTargetOrigins(List targetOrigins) {
this.targetOrigins = targetOrigins;
}
public GenerateCaptureContextRequest allowedCardNetworks(List allowedCardNetworks) {
this.allowedCardNetworks = allowedCardNetworks;
return this;
}
public GenerateCaptureContextRequest addAllowedCardNetworksItem(String allowedCardNetworksItem) {
if (this.allowedCardNetworks == null) {
this.allowedCardNetworks = new ArrayList();
}
this.allowedCardNetworks.add(allowedCardNetworksItem);
return this;
}
/**
* The list of card networks you want to use for this Microform transaction. Microform currently supports the following card networks: - VISA - MAESTRO - MASTERCARD - AMEX - DISCOVER - DINERSCLUB - JCB - CUP - CARTESBANCAIRES - CARNET
* @return allowedCardNetworks
**/
@ApiModelProperty(value = "The list of card networks you want to use for this Microform transaction. Microform currently supports the following card networks: - VISA - MAESTRO - MASTERCARD - AMEX - DISCOVER - DINERSCLUB - JCB - CUP - CARTESBANCAIRES - CARNET ")
public List getAllowedCardNetworks() {
return allowedCardNetworks;
}
public void setAllowedCardNetworks(List allowedCardNetworks) {
this.allowedCardNetworks = allowedCardNetworks;
}
public GenerateCaptureContextRequest clientVersion(String clientVersion) {
this.clientVersion = clientVersion;
return this;
}
/**
* Specify the version of Microform that you want to use.
* @return clientVersion
**/
@ApiModelProperty(value = "Specify the version of Microform that you want to use. ")
public String getClientVersion() {
return clientVersion;
}
public void setClientVersion(String clientVersion) {
this.clientVersion = clientVersion;
}
public GenerateCaptureContextRequest checkoutApiInitialization(Microformv2sessionsCheckoutApiInitialization checkoutApiInitialization) {
this.checkoutApiInitialization = checkoutApiInitialization;
return this;
}
/**
* Get checkoutApiInitialization
* @return checkoutApiInitialization
**/
@ApiModelProperty(value = "")
public Microformv2sessionsCheckoutApiInitialization getCheckoutApiInitialization() {
return checkoutApiInitialization;
}
public void setCheckoutApiInitialization(Microformv2sessionsCheckoutApiInitialization checkoutApiInitialization) {
this.checkoutApiInitialization = checkoutApiInitialization;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
GenerateCaptureContextRequest generateCaptureContextRequest = (GenerateCaptureContextRequest) o;
return Objects.equals(this.targetOrigins, generateCaptureContextRequest.targetOrigins) &&
Objects.equals(this.allowedCardNetworks, generateCaptureContextRequest.allowedCardNetworks) &&
Objects.equals(this.clientVersion, generateCaptureContextRequest.clientVersion) &&
Objects.equals(this.checkoutApiInitialization, generateCaptureContextRequest.checkoutApiInitialization);
}
@Override
public int hashCode() {
return Objects.hash(targetOrigins, allowedCardNetworks, clientVersion, checkoutApiInitialization);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class GenerateCaptureContextRequest {\n");
sb.append(" targetOrigins: ").append(toIndentedString(targetOrigins)).append("\n");
sb.append(" allowedCardNetworks: ").append(toIndentedString(allowedCardNetworks)).append("\n");
sb.append(" clientVersion: ").append(toIndentedString(clientVersion)).append("\n");
sb.append(" checkoutApiInitialization: ").append(toIndentedString(checkoutApiInitialization)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy