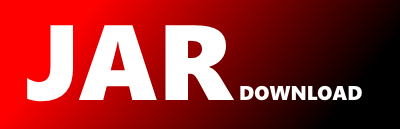
Model.Kmsegressv2keysasymKeyInformation Maven / Gradle / Ivy
/*
* CyberSource Merged Spec
* All CyberSource API specs merged together. These are available at https://developer.cybersource.com/api/reference/api-reference.html
*
* OpenAPI spec version: 0.0.1
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package Model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
/**
* Egress Asymmetric Key Information Request
*/
@ApiModel(description = "Egress Asymmetric Key Information Request ")
public class Kmsegressv2keysasymKeyInformation {
@SerializedName("provider")
private String provider = null;
@SerializedName("tenant")
private String tenant = null;
@SerializedName("keyType")
private String keyType = null;
@SerializedName("organizationId")
private String organizationId = null;
@SerializedName("pub")
private String pub = null;
@SerializedName("keyId")
private String keyId = null;
@SerializedName("pvt")
private String pvt = null;
@SerializedName("status")
private String status = null;
@SerializedName("expiryDuration")
private String expiryDuration = null;
public Kmsegressv2keysasymKeyInformation provider(String provider) {
this.provider = provider;
return this;
}
/**
* Provider name
* @return provider
**/
@ApiModelProperty(value = "Provider name ")
public String getProvider() {
return provider;
}
public void setProvider(String provider) {
this.provider = provider;
}
public Kmsegressv2keysasymKeyInformation tenant(String tenant) {
this.tenant = tenant;
return this;
}
/**
* Tenant name
* @return tenant
**/
@ApiModelProperty(value = "Tenant name ")
public String getTenant() {
return tenant;
}
public void setTenant(String tenant) {
this.tenant = tenant;
}
public Kmsegressv2keysasymKeyInformation keyType(String keyType) {
this.keyType = keyType;
return this;
}
/**
* Type of the key
* @return keyType
**/
@ApiModelProperty(value = "Type of the key ")
public String getKeyType() {
return keyType;
}
public void setKeyType(String keyType) {
this.keyType = keyType;
}
public Kmsegressv2keysasymKeyInformation organizationId(String organizationId) {
this.organizationId = organizationId;
return this;
}
/**
* Organization Id
* @return organizationId
**/
@ApiModelProperty(value = "Organization Id ")
public String getOrganizationId() {
return organizationId;
}
public void setOrganizationId(String organizationId) {
this.organizationId = organizationId;
}
public Kmsegressv2keysasymKeyInformation pub(String pub) {
this.pub = pub;
return this;
}
/**
* Public certificate with only base64 encoded payload and not the header (BEGIN CERTIFICATE) and footer (END CERTIFICATE)
* @return pub
**/
@ApiModelProperty(value = "Public certificate with only base64 encoded payload and not the header (BEGIN CERTIFICATE) and footer (END CERTIFICATE) ")
public String getPub() {
return pub;
}
public void setPub(String pub) {
this.pub = pub;
}
public Kmsegressv2keysasymKeyInformation keyId(String keyId) {
this.keyId = keyId;
return this;
}
/**
* Key Serial Number
* @return keyId
**/
@ApiModelProperty(value = "Key Serial Number ")
public String getKeyId() {
return keyId;
}
public void setKeyId(String keyId) {
this.keyId = keyId;
}
public Kmsegressv2keysasymKeyInformation pvt(String pvt) {
this.pvt = pvt;
return this;
}
/**
* Private certificate with only base64 encoded payload and not header (BEGIN CERTIFICATE) and footer (END CERTIFICATE)
* @return pvt
**/
@ApiModelProperty(value = "Private certificate with only base64 encoded payload and not header (BEGIN CERTIFICATE) and footer (END CERTIFICATE) ")
public String getPvt() {
return pvt;
}
public void setPvt(String pvt) {
this.pvt = pvt;
}
public Kmsegressv2keysasymKeyInformation status(String status) {
this.status = status;
return this;
}
/**
* The status of the key
* @return status
**/
@ApiModelProperty(value = "The status of the key ")
public String getStatus() {
return status;
}
public void setStatus(String status) {
this.status = status;
}
public Kmsegressv2keysasymKeyInformation expiryDuration(String expiryDuration) {
this.expiryDuration = expiryDuration;
return this;
}
/**
* Key expiry duration in days
* @return expiryDuration
**/
@ApiModelProperty(value = "Key expiry duration in days ")
public String getExpiryDuration() {
return expiryDuration;
}
public void setExpiryDuration(String expiryDuration) {
this.expiryDuration = expiryDuration;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Kmsegressv2keysasymKeyInformation kmsegressv2keysasymKeyInformation = (Kmsegressv2keysasymKeyInformation) o;
return Objects.equals(this.provider, kmsegressv2keysasymKeyInformation.provider) &&
Objects.equals(this.tenant, kmsegressv2keysasymKeyInformation.tenant) &&
Objects.equals(this.keyType, kmsegressv2keysasymKeyInformation.keyType) &&
Objects.equals(this.organizationId, kmsegressv2keysasymKeyInformation.organizationId) &&
Objects.equals(this.pub, kmsegressv2keysasymKeyInformation.pub) &&
Objects.equals(this.keyId, kmsegressv2keysasymKeyInformation.keyId) &&
Objects.equals(this.pvt, kmsegressv2keysasymKeyInformation.pvt) &&
Objects.equals(this.status, kmsegressv2keysasymKeyInformation.status) &&
Objects.equals(this.expiryDuration, kmsegressv2keysasymKeyInformation.expiryDuration);
}
@Override
public int hashCode() {
return Objects.hash(provider, tenant, keyType, organizationId, pub, keyId, pvt, status, expiryDuration);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Kmsegressv2keysasymKeyInformation {\n");
sb.append(" provider: ").append(toIndentedString(provider)).append("\n");
sb.append(" tenant: ").append(toIndentedString(tenant)).append("\n");
sb.append(" keyType: ").append(toIndentedString(keyType)).append("\n");
sb.append(" organizationId: ").append(toIndentedString(organizationId)).append("\n");
sb.append(" pub: ").append(toIndentedString(pub)).append("\n");
sb.append(" keyId: ").append(toIndentedString(keyId)).append("\n");
sb.append(" pvt: ").append(toIndentedString(pvt)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" expiryDuration: ").append(toIndentedString(expiryDuration)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy