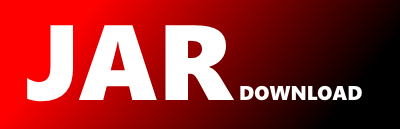
Model.PayerAuthConfigCardTypesVerifiedByVisaCurrencies Maven / Gradle / Ivy
/*
* CyberSource Merged Spec
* All CyberSource API specs merged together. These are available at https://developer.cybersource.com/api/reference/api-reference.html
*
* OpenAPI spec version: 0.0.1
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package Model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
/**
* PayerAuthConfigCardTypesVerifiedByVisaCurrencies
*/
public class PayerAuthConfigCardTypesVerifiedByVisaCurrencies {
@SerializedName("currencyCodes")
private List currencyCodes = null;
@SerializedName("acquirerId")
private String acquirerId = null;
@SerializedName("processorMerchantId")
private String processorMerchantId = null;
public PayerAuthConfigCardTypesVerifiedByVisaCurrencies currencyCodes(List currencyCodes) {
this.currencyCodes = currencyCodes;
return this;
}
public PayerAuthConfigCardTypesVerifiedByVisaCurrencies addCurrencyCodesItem(String currencyCodesItem) {
if (this.currencyCodes == null) {
this.currencyCodes = new ArrayList();
}
this.currencyCodes.add(currencyCodesItem);
return this;
}
/**
* Supported currency codes are numeric ISO 4217 codes, such as 840 for US Dollar and 978 for Euro. For backward compatibility, we also support the 'ALL' code, which represents all currencies. In the UI, 'ALL' is displayed as 'Default'.
* @return currencyCodes
**/
@ApiModelProperty(example = "[\"ALL\",\"840\",\"978\"]", value = "Supported currency codes are numeric ISO 4217 codes, such as 840 for US Dollar and 978 for Euro. For backward compatibility, we also support the 'ALL' code, which represents all currencies. In the UI, 'ALL' is displayed as 'Default'. ")
public List getCurrencyCodes() {
return currencyCodes;
}
public void setCurrencyCodes(List currencyCodes) {
this.currencyCodes = currencyCodes;
}
public PayerAuthConfigCardTypesVerifiedByVisaCurrencies acquirerId(String acquirerId) {
this.acquirerId = acquirerId;
return this;
}
/**
* The Acquirer ID value, often referred to as the Acquirer BIN, is specific to an Acquirer. The value is created by Cardinal in their system and the Acquirer may not know that the Acquirer ID is different from their Acquiring BIN. It is most often the Acquiring BIN + \"-1000\" but the trailing character can be different. **Note** We will need to double check with Cardinal before setting up the Portfolio Template in production.
* @return acquirerId
**/
@ApiModelProperty(value = "The Acquirer ID value, often referred to as the Acquirer BIN, is specific to an Acquirer. The value is created by Cardinal in their system and the Acquirer may not know that the Acquirer ID is different from their Acquiring BIN. It is most often the Acquiring BIN + \"-1000\" but the trailing character can be different. **Note** We will need to double check with Cardinal before setting up the Portfolio Template in production. ")
public String getAcquirerId() {
return acquirerId;
}
public void setAcquirerId(String acquirerId) {
this.acquirerId = acquirerId;
}
public PayerAuthConfigCardTypesVerifiedByVisaCurrencies processorMerchantId(String processorMerchantId) {
this.processorMerchantId = processorMerchantId;
return this;
}
/**
* Processor Merchant ID is the Merchant ID assigned by your acquiring bank. This Merchant ID should also be used by your bank to register your account to the card scheme Directory Server for processing Payer Authentication services.
* @return processorMerchantId
**/
@ApiModelProperty(value = "Processor Merchant ID is the Merchant ID assigned by your acquiring bank. This Merchant ID should also be used by your bank to register your account to the card scheme Directory Server for processing Payer Authentication services. ")
public String getProcessorMerchantId() {
return processorMerchantId;
}
public void setProcessorMerchantId(String processorMerchantId) {
this.processorMerchantId = processorMerchantId;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
PayerAuthConfigCardTypesVerifiedByVisaCurrencies payerAuthConfigCardTypesVerifiedByVisaCurrencies = (PayerAuthConfigCardTypesVerifiedByVisaCurrencies) o;
return Objects.equals(this.currencyCodes, payerAuthConfigCardTypesVerifiedByVisaCurrencies.currencyCodes) &&
Objects.equals(this.acquirerId, payerAuthConfigCardTypesVerifiedByVisaCurrencies.acquirerId) &&
Objects.equals(this.processorMerchantId, payerAuthConfigCardTypesVerifiedByVisaCurrencies.processorMerchantId);
}
@Override
public int hashCode() {
return Objects.hash(currencyCodes, acquirerId, processorMerchantId);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class PayerAuthConfigCardTypesVerifiedByVisaCurrencies {\n");
sb.append(" currencyCodes: ").append(toIndentedString(currencyCodes)).append("\n");
sb.append(" acquirerId: ").append(toIndentedString(acquirerId)).append("\n");
sb.append(" processorMerchantId: ").append(toIndentedString(processorMerchantId)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy