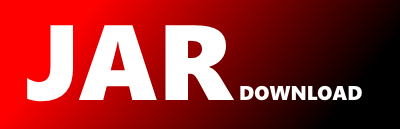
Model.PaymentsProductsCardProcessingSubscriptionInformation Maven / Gradle / Ivy
/*
* CyberSource Merged Spec
* All CyberSource API specs merged together. These are available at https://developer.cybersource.com/api/reference/api-reference.html
*
* OpenAPI spec version: 0.0.1
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package Model;
import java.util.Objects;
import java.util.Arrays;
import Model.PaymentsProductsCardProcessingSubscriptionInformationFeatures;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* PaymentsProductsCardProcessingSubscriptionInformation
*/
public class PaymentsProductsCardProcessingSubscriptionInformation {
@SerializedName("enabled")
private Boolean enabled = null;
@SerializedName("selfServiceability")
private String selfServiceability = "NOT_SELF_SERVICEABLE";
@SerializedName("features")
private Map features = null;
public PaymentsProductsCardProcessingSubscriptionInformation enabled(Boolean enabled) {
this.enabled = enabled;
return this;
}
/**
* Get enabled
* @return enabled
**/
@ApiModelProperty(value = "")
public Boolean isEnabled() {
return enabled;
}
public void setEnabled(Boolean enabled) {
this.enabled = enabled;
}
public PaymentsProductsCardProcessingSubscriptionInformation selfServiceability(String selfServiceability) {
this.selfServiceability = selfServiceability;
return this;
}
/**
* Indicates if the organization can enable this product using self service. Possible values: - SELF_SERVICEABLE - NOT_SELF_SERVICEABLE - SELF_SERVICE_ONLY
* @return selfServiceability
**/
@ApiModelProperty(value = "Indicates if the organization can enable this product using self service. Possible values: - SELF_SERVICEABLE - NOT_SELF_SERVICEABLE - SELF_SERVICE_ONLY")
public String getSelfServiceability() {
return selfServiceability;
}
public void setSelfServiceability(String selfServiceability) {
this.selfServiceability = selfServiceability;
}
public PaymentsProductsCardProcessingSubscriptionInformation features(Map features) {
this.features = features;
return this;
}
public PaymentsProductsCardProcessingSubscriptionInformation putFeaturesItem(String key, PaymentsProductsCardProcessingSubscriptionInformationFeatures featuresItem) {
if (this.features == null) {
this.features = new HashMap();
}
this.features.put(key, featuresItem);
return this;
}
/**
* This is a map. The allowed keys are below. Value should be an object containing a sole boolean property - enabled. <table> <tr> <td>cardPresent</td> </tr> <tr> <td>cardNotPresent</td> </tr> </table>
* @return features
**/
@ApiModelProperty(value = "This is a map. The allowed keys are below. Value should be an object containing a sole boolean property - enabled. cardPresent cardNotPresent
")
public Map getFeatures() {
return features;
}
public void setFeatures(Map features) {
this.features = features;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
PaymentsProductsCardProcessingSubscriptionInformation paymentsProductsCardProcessingSubscriptionInformation = (PaymentsProductsCardProcessingSubscriptionInformation) o;
return Objects.equals(this.enabled, paymentsProductsCardProcessingSubscriptionInformation.enabled) &&
Objects.equals(this.selfServiceability, paymentsProductsCardProcessingSubscriptionInformation.selfServiceability) &&
Objects.equals(this.features, paymentsProductsCardProcessingSubscriptionInformation.features);
}
@Override
public int hashCode() {
return Objects.hash(enabled, selfServiceability, features);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class PaymentsProductsCardProcessingSubscriptionInformation {\n");
sb.append(" enabled: ").append(toIndentedString(enabled)).append("\n");
sb.append(" selfServiceability: ").append(toIndentedString(selfServiceability)).append("\n");
sb.append(" features: ").append(toIndentedString(features)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy