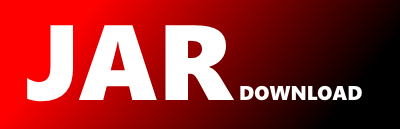
Model.PaymentsProductsPayoutsConfigurationInformationConfigurationsPushfunds Maven / Gradle / Ivy
/*
* CyberSource Merged Spec
* All CyberSource API specs merged together. These are available at https://developer.cybersource.com/api/reference/api-reference.html
*
* OpenAPI spec version: 0.0.1
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package Model;
import java.util.Objects;
import java.util.Arrays;
import Model.PaymentsProductsPayoutsConfigurationInformationConfigurationsProcessorAccount;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
/**
* Formatted as *{payoutsAcquirerName}. The property name field should be the same as the processor name for which the pull funds or push funds feature is being configured. Here is the list of valid processor names [TBD]
*/
@ApiModel(description = "Formatted as *{payoutsAcquirerName}. The property name field should be the same as the processor name for which the pull funds or push funds feature is being configured. Here is the list of valid processor names [TBD]")
public class PaymentsProductsPayoutsConfigurationInformationConfigurationsPushfunds {
@SerializedName("acquirerCountryCode")
private Integer acquirerCountryCode = null;
@SerializedName("acquiringBIN")
private Integer acquiringBIN = null;
@SerializedName("allowCryptoCurrencyPurchase")
private Boolean allowCryptoCurrencyPurchase = null;
@SerializedName("financialInstitutionId")
private String financialInstitutionId = null;
@SerializedName("networkOrder")
private String networkOrder = null;
@SerializedName("nationalReimbursementFee")
private String nationalReimbursementFee = null;
@SerializedName("originatorBusinessApplicationId")
private String originatorBusinessApplicationId = null;
@SerializedName("originatorPseudoAbaNumber")
private String originatorPseudoAbaNumber = null;
@SerializedName("processorAccount")
private List processorAccount = new ArrayList();
public PaymentsProductsPayoutsConfigurationInformationConfigurationsPushfunds acquirerCountryCode(Integer acquirerCountryCode) {
this.acquirerCountryCode = acquirerCountryCode;
return this;
}
/**
* TBD
* @return acquirerCountryCode
**/
@ApiModelProperty(required = true, value = "TBD")
public Integer getAcquirerCountryCode() {
return acquirerCountryCode;
}
public void setAcquirerCountryCode(Integer acquirerCountryCode) {
this.acquirerCountryCode = acquirerCountryCode;
}
public PaymentsProductsPayoutsConfigurationInformationConfigurationsPushfunds acquiringBIN(Integer acquiringBIN) {
this.acquiringBIN = acquiringBIN;
return this;
}
/**
* TBD
* @return acquiringBIN
**/
@ApiModelProperty(required = true, value = "TBD")
public Integer getAcquiringBIN() {
return acquiringBIN;
}
public void setAcquiringBIN(Integer acquiringBIN) {
this.acquiringBIN = acquiringBIN;
}
public PaymentsProductsPayoutsConfigurationInformationConfigurationsPushfunds allowCryptoCurrencyPurchase(Boolean allowCryptoCurrencyPurchase) {
this.allowCryptoCurrencyPurchase = allowCryptoCurrencyPurchase;
return this;
}
/**
* This configuration allows a transaction to be flagged for cryptocurrency funds transfer.
* @return allowCryptoCurrencyPurchase
**/
@ApiModelProperty(value = "This configuration allows a transaction to be flagged for cryptocurrency funds transfer.")
public Boolean isAllowCryptoCurrencyPurchase() {
return allowCryptoCurrencyPurchase;
}
public void setAllowCryptoCurrencyPurchase(Boolean allowCryptoCurrencyPurchase) {
this.allowCryptoCurrencyPurchase = allowCryptoCurrencyPurchase;
}
public PaymentsProductsPayoutsConfigurationInformationConfigurationsPushfunds financialInstitutionId(String financialInstitutionId) {
this.financialInstitutionId = financialInstitutionId;
return this;
}
/**
* TBD
* @return financialInstitutionId
**/
@ApiModelProperty(value = "TBD")
public String getFinancialInstitutionId() {
return financialInstitutionId;
}
public void setFinancialInstitutionId(String financialInstitutionId) {
this.financialInstitutionId = financialInstitutionId;
}
public PaymentsProductsPayoutsConfigurationInformationConfigurationsPushfunds networkOrder(String networkOrder) {
this.networkOrder = networkOrder;
return this;
}
/**
* TBD
* @return networkOrder
**/
@ApiModelProperty(value = "TBD")
public String getNetworkOrder() {
return networkOrder;
}
public void setNetworkOrder(String networkOrder) {
this.networkOrder = networkOrder;
}
public PaymentsProductsPayoutsConfigurationInformationConfigurationsPushfunds nationalReimbursementFee(String nationalReimbursementFee) {
this.nationalReimbursementFee = nationalReimbursementFee;
return this;
}
/**
* TBD
* @return nationalReimbursementFee
**/
@ApiModelProperty(value = "TBD")
public String getNationalReimbursementFee() {
return nationalReimbursementFee;
}
public void setNationalReimbursementFee(String nationalReimbursementFee) {
this.nationalReimbursementFee = nationalReimbursementFee;
}
public PaymentsProductsPayoutsConfigurationInformationConfigurationsPushfunds originatorBusinessApplicationId(String originatorBusinessApplicationId) {
this.originatorBusinessApplicationId = originatorBusinessApplicationId;
return this;
}
/**
* TBD
* @return originatorBusinessApplicationId
**/
@ApiModelProperty(required = true, value = "TBD")
public String getOriginatorBusinessApplicationId() {
return originatorBusinessApplicationId;
}
public void setOriginatorBusinessApplicationId(String originatorBusinessApplicationId) {
this.originatorBusinessApplicationId = originatorBusinessApplicationId;
}
public PaymentsProductsPayoutsConfigurationInformationConfigurationsPushfunds originatorPseudoAbaNumber(String originatorPseudoAbaNumber) {
this.originatorPseudoAbaNumber = originatorPseudoAbaNumber;
return this;
}
/**
* TBD
* @return originatorPseudoAbaNumber
**/
@ApiModelProperty(value = "TBD")
public String getOriginatorPseudoAbaNumber() {
return originatorPseudoAbaNumber;
}
public void setOriginatorPseudoAbaNumber(String originatorPseudoAbaNumber) {
this.originatorPseudoAbaNumber = originatorPseudoAbaNumber;
}
public PaymentsProductsPayoutsConfigurationInformationConfigurationsPushfunds processorAccount(List processorAccount) {
this.processorAccount = processorAccount;
return this;
}
public PaymentsProductsPayoutsConfigurationInformationConfigurationsPushfunds addProcessorAccountItem(PaymentsProductsPayoutsConfigurationInformationConfigurationsProcessorAccount processorAccountItem) {
this.processorAccount.add(processorAccountItem);
return this;
}
/**
* TBD
* @return processorAccount
**/
@ApiModelProperty(required = true, value = "TBD")
public List getProcessorAccount() {
return processorAccount;
}
public void setProcessorAccount(List processorAccount) {
this.processorAccount = processorAccount;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
PaymentsProductsPayoutsConfigurationInformationConfigurationsPushfunds paymentsProductsPayoutsConfigurationInformationConfigurationsPushfunds = (PaymentsProductsPayoutsConfigurationInformationConfigurationsPushfunds) o;
return Objects.equals(this.acquirerCountryCode, paymentsProductsPayoutsConfigurationInformationConfigurationsPushfunds.acquirerCountryCode) &&
Objects.equals(this.acquiringBIN, paymentsProductsPayoutsConfigurationInformationConfigurationsPushfunds.acquiringBIN) &&
Objects.equals(this.allowCryptoCurrencyPurchase, paymentsProductsPayoutsConfigurationInformationConfigurationsPushfunds.allowCryptoCurrencyPurchase) &&
Objects.equals(this.financialInstitutionId, paymentsProductsPayoutsConfigurationInformationConfigurationsPushfunds.financialInstitutionId) &&
Objects.equals(this.networkOrder, paymentsProductsPayoutsConfigurationInformationConfigurationsPushfunds.networkOrder) &&
Objects.equals(this.nationalReimbursementFee, paymentsProductsPayoutsConfigurationInformationConfigurationsPushfunds.nationalReimbursementFee) &&
Objects.equals(this.originatorBusinessApplicationId, paymentsProductsPayoutsConfigurationInformationConfigurationsPushfunds.originatorBusinessApplicationId) &&
Objects.equals(this.originatorPseudoAbaNumber, paymentsProductsPayoutsConfigurationInformationConfigurationsPushfunds.originatorPseudoAbaNumber) &&
Objects.equals(this.processorAccount, paymentsProductsPayoutsConfigurationInformationConfigurationsPushfunds.processorAccount);
}
@Override
public int hashCode() {
return Objects.hash(acquirerCountryCode, acquiringBIN, allowCryptoCurrencyPurchase, financialInstitutionId, networkOrder, nationalReimbursementFee, originatorBusinessApplicationId, originatorPseudoAbaNumber, processorAccount);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class PaymentsProductsPayoutsConfigurationInformationConfigurationsPushfunds {\n");
sb.append(" acquirerCountryCode: ").append(toIndentedString(acquirerCountryCode)).append("\n");
sb.append(" acquiringBIN: ").append(toIndentedString(acquiringBIN)).append("\n");
sb.append(" allowCryptoCurrencyPurchase: ").append(toIndentedString(allowCryptoCurrencyPurchase)).append("\n");
sb.append(" financialInstitutionId: ").append(toIndentedString(financialInstitutionId)).append("\n");
sb.append(" networkOrder: ").append(toIndentedString(networkOrder)).append("\n");
sb.append(" nationalReimbursementFee: ").append(toIndentedString(nationalReimbursementFee)).append("\n");
sb.append(" originatorBusinessApplicationId: ").append(toIndentedString(originatorBusinessApplicationId)).append("\n");
sb.append(" originatorPseudoAbaNumber: ").append(toIndentedString(originatorPseudoAbaNumber)).append("\n");
sb.append(" processorAccount: ").append(toIndentedString(processorAccount)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy