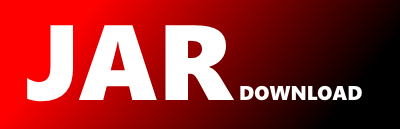
Model.PtsV2PaymentsPost201ResponseProcessingInformation Maven / Gradle / Ivy
/*
* CyberSource Merged Spec
* All CyberSource API specs merged together. These are available at https://developer.cybersource.com/api/reference/api-reference.html
*
* OpenAPI spec version: 0.0.1
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package Model;
import java.util.Objects;
import java.util.Arrays;
import Model.PtsV2PaymentsPost201ResponseProcessingInformationBankTransferOptions;
import Model.PtsV2PaymentsPost201ResponseProcessingInformationCaptureOptions;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
/**
* PtsV2PaymentsPost201ResponseProcessingInformation
*/
public class PtsV2PaymentsPost201ResponseProcessingInformation {
@SerializedName("bankTransferOptions")
private PtsV2PaymentsPost201ResponseProcessingInformationBankTransferOptions bankTransferOptions = null;
@SerializedName("paymentSolution")
private String paymentSolution = null;
@SerializedName("enhancedDataEnabled")
private Boolean enhancedDataEnabled = null;
@SerializedName("captureOptions")
private PtsV2PaymentsPost201ResponseProcessingInformationCaptureOptions captureOptions = null;
public PtsV2PaymentsPost201ResponseProcessingInformation bankTransferOptions(PtsV2PaymentsPost201ResponseProcessingInformationBankTransferOptions bankTransferOptions) {
this.bankTransferOptions = bankTransferOptions;
return this;
}
/**
* Get bankTransferOptions
* @return bankTransferOptions
**/
@ApiModelProperty(value = "")
public PtsV2PaymentsPost201ResponseProcessingInformationBankTransferOptions getBankTransferOptions() {
return bankTransferOptions;
}
public void setBankTransferOptions(PtsV2PaymentsPost201ResponseProcessingInformationBankTransferOptions bankTransferOptions) {
this.bankTransferOptions = bankTransferOptions;
}
public PtsV2PaymentsPost201ResponseProcessingInformation paymentSolution(String paymentSolution) {
this.paymentSolution = paymentSolution;
return this;
}
/**
* Type of digital payment solution for the transaction. Possible Values: - `visacheckout`: Visa Checkout. This value is required for Visa Checkout transactions. For details, see `payment_solution` field description in [Visa Checkout Using the REST API.](https://developer.cybersource.com/content/dam/docs/cybs/en-us/apifields/reference/all/rest/api-fields.pdf) - `001`: Apple Pay. - `004`: Cybersource In-App Solution. - `005`: Masterpass. This value is required for Masterpass transactions on OmniPay Direct. - `006`: Android Pay. - `007`: Chase Pay. - `008`: Samsung Pay. - `012`: Google Pay. - `013`: Cybersource P2PE Decryption - `014`: Mastercard credential on file (COF) payment network token. Returned in authorizations that use a payment network token associated with a TMS token. - `015`: Visa credential on file (COF) payment network token. Returned in authorizations that use a payment network token associated with a TMS token. - `027`: Click to Pay.
* @return paymentSolution
**/
@ApiModelProperty(value = "Type of digital payment solution for the transaction. Possible Values: - `visacheckout`: Visa Checkout. This value is required for Visa Checkout transactions. For details, see `payment_solution` field description in [Visa Checkout Using the REST API.](https://developer.cybersource.com/content/dam/docs/cybs/en-us/apifields/reference/all/rest/api-fields.pdf) - `001`: Apple Pay. - `004`: Cybersource In-App Solution. - `005`: Masterpass. This value is required for Masterpass transactions on OmniPay Direct. - `006`: Android Pay. - `007`: Chase Pay. - `008`: Samsung Pay. - `012`: Google Pay. - `013`: Cybersource P2PE Decryption - `014`: Mastercard credential on file (COF) payment network token. Returned in authorizations that use a payment network token associated with a TMS token. - `015`: Visa credential on file (COF) payment network token. Returned in authorizations that use a payment network token associated with a TMS token. - `027`: Click to Pay. ")
public String getPaymentSolution() {
return paymentSolution;
}
public void setPaymentSolution(String paymentSolution) {
this.paymentSolution = paymentSolution;
}
public PtsV2PaymentsPost201ResponseProcessingInformation enhancedDataEnabled(Boolean enhancedDataEnabled) {
this.enhancedDataEnabled = enhancedDataEnabled;
return this;
}
/**
* The possible values for the reply field are: - `true` : the airline data was included in the request to the processor. - `false` : the airline data was not included in the request to the processor. Returned by authorization, capture, or credit services.
* @return enhancedDataEnabled
**/
@ApiModelProperty(value = "The possible values for the reply field are: - `true` : the airline data was included in the request to the processor. - `false` : the airline data was not included in the request to the processor. Returned by authorization, capture, or credit services. ")
public Boolean isEnhancedDataEnabled() {
return enhancedDataEnabled;
}
public void setEnhancedDataEnabled(Boolean enhancedDataEnabled) {
this.enhancedDataEnabled = enhancedDataEnabled;
}
public PtsV2PaymentsPost201ResponseProcessingInformation captureOptions(PtsV2PaymentsPost201ResponseProcessingInformationCaptureOptions captureOptions) {
this.captureOptions = captureOptions;
return this;
}
/**
* Get captureOptions
* @return captureOptions
**/
@ApiModelProperty(value = "")
public PtsV2PaymentsPost201ResponseProcessingInformationCaptureOptions getCaptureOptions() {
return captureOptions;
}
public void setCaptureOptions(PtsV2PaymentsPost201ResponseProcessingInformationCaptureOptions captureOptions) {
this.captureOptions = captureOptions;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
PtsV2PaymentsPost201ResponseProcessingInformation ptsV2PaymentsPost201ResponseProcessingInformation = (PtsV2PaymentsPost201ResponseProcessingInformation) o;
return Objects.equals(this.bankTransferOptions, ptsV2PaymentsPost201ResponseProcessingInformation.bankTransferOptions) &&
Objects.equals(this.paymentSolution, ptsV2PaymentsPost201ResponseProcessingInformation.paymentSolution) &&
Objects.equals(this.enhancedDataEnabled, ptsV2PaymentsPost201ResponseProcessingInformation.enhancedDataEnabled) &&
Objects.equals(this.captureOptions, ptsV2PaymentsPost201ResponseProcessingInformation.captureOptions);
}
@Override
public int hashCode() {
return Objects.hash(bankTransferOptions, paymentSolution, enhancedDataEnabled, captureOptions);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class PtsV2PaymentsPost201ResponseProcessingInformation {\n");
sb.append(" bankTransferOptions: ").append(toIndentedString(bankTransferOptions)).append("\n");
sb.append(" paymentSolution: ").append(toIndentedString(paymentSolution)).append("\n");
sb.append(" enhancedDataEnabled: ").append(toIndentedString(enhancedDataEnabled)).append("\n");
sb.append(" captureOptions: ").append(toIndentedString(captureOptions)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy