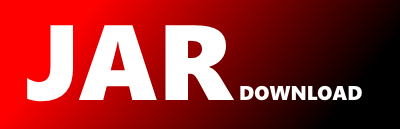
Model.Ptsv2paymentsBuyerInformation Maven / Gradle / Ivy
/*
* CyberSource Merged Spec
* All CyberSource API specs merged together. These are available at https://developer.cybersource.com/api/reference/api-reference.html
*
* OpenAPI spec version: 0.0.1
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package Model;
import java.util.Objects;
import java.util.Arrays;
import Model.Ptsv2paymentsBuyerInformationPersonalIdentification;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
/**
* Ptsv2paymentsBuyerInformation
*/
public class Ptsv2paymentsBuyerInformation {
@SerializedName("merchantCustomerId")
private String merchantCustomerId = null;
@SerializedName("dateOfBirth")
private String dateOfBirth = null;
@SerializedName("vatRegistrationNumber")
private String vatRegistrationNumber = null;
@SerializedName("companyTaxId")
private String companyTaxId = null;
@SerializedName("personalIdentification")
private List personalIdentification = null;
@SerializedName("hashedPassword")
private String hashedPassword = null;
@SerializedName("gender")
private String gender = null;
@SerializedName("language")
private String language = null;
@SerializedName("noteToSeller")
private String noteToSeller = null;
@SerializedName("mobilePhone")
private Integer mobilePhone = null;
@SerializedName("walletId")
private String walletId = null;
public Ptsv2paymentsBuyerInformation merchantCustomerId(String merchantCustomerId) {
this.merchantCustomerId = merchantCustomerId;
return this;
}
/**
* Your identifier for the customer. When a subscription or customer profile is being created, the maximum length for this field for most processors is 30. Otherwise, the maximum length is 100. #### Comercio Latino For recurring payments in Mexico, the value is the customer's contract number. Note Before you request the authorization, you must inform the issuer of the customer contract numbers that will be used for recurring transactions. #### Worldpay VAP For a follow-on credit with Worldpay VAP, CyberSource checks the following locations, in the order given, for a customer account ID value and uses the first value it finds: 1. `customer_account_id` value in the follow-on credit request 2. Customer account ID value that was used for the capture that is being credited 3. Customer account ID value that was used for the original authorization If a customer account ID value cannot be found in any of these locations, then no value is used.
* @return merchantCustomerId
**/
@ApiModelProperty(value = "Your identifier for the customer. When a subscription or customer profile is being created, the maximum length for this field for most processors is 30. Otherwise, the maximum length is 100. #### Comercio Latino For recurring payments in Mexico, the value is the customer's contract number. Note Before you request the authorization, you must inform the issuer of the customer contract numbers that will be used for recurring transactions. #### Worldpay VAP For a follow-on credit with Worldpay VAP, CyberSource checks the following locations, in the order given, for a customer account ID value and uses the first value it finds: 1. `customer_account_id` value in the follow-on credit request 2. Customer account ID value that was used for the capture that is being credited 3. Customer account ID value that was used for the original authorization If a customer account ID value cannot be found in any of these locations, then no value is used. ")
public String getMerchantCustomerId() {
return merchantCustomerId;
}
public void setMerchantCustomerId(String merchantCustomerId) {
this.merchantCustomerId = merchantCustomerId;
}
public Ptsv2paymentsBuyerInformation dateOfBirth(String dateOfBirth) {
this.dateOfBirth = dateOfBirth;
return this;
}
/**
* Recipient's date of birth. **Format**: `YYYYMMDD`. This field is a `pass-through`, which means that CyberSource ensures that the value is eight numeric characters but otherwise does not verify the value or modify it in any way before sending it to the processor. If the field is not required for the transaction, CyberSource does not forward it to the processor.
* @return dateOfBirth
**/
@ApiModelProperty(value = "Recipient's date of birth. **Format**: `YYYYMMDD`. This field is a `pass-through`, which means that CyberSource ensures that the value is eight numeric characters but otherwise does not verify the value or modify it in any way before sending it to the processor. If the field is not required for the transaction, CyberSource does not forward it to the processor. ")
public String getDateOfBirth() {
return dateOfBirth;
}
public void setDateOfBirth(String dateOfBirth) {
this.dateOfBirth = dateOfBirth;
}
public Ptsv2paymentsBuyerInformation vatRegistrationNumber(String vatRegistrationNumber) {
this.vatRegistrationNumber = vatRegistrationNumber;
return this;
}
/**
* Customer's government-assigned tax identification number. #### Tax Calculation Optional for international and value added taxes only. Not applicable to U.S. and Canadian taxes.
* @return vatRegistrationNumber
**/
@ApiModelProperty(value = "Customer's government-assigned tax identification number. #### Tax Calculation Optional for international and value added taxes only. Not applicable to U.S. and Canadian taxes. ")
public String getVatRegistrationNumber() {
return vatRegistrationNumber;
}
public void setVatRegistrationNumber(String vatRegistrationNumber) {
this.vatRegistrationNumber = vatRegistrationNumber;
}
public Ptsv2paymentsBuyerInformation companyTaxId(String companyTaxId) {
this.companyTaxId = companyTaxId;
return this;
}
/**
* Company's tax identifier. This is only used for eCheck service. ** TeleCheck ** Contact your TeleCheck representative to find out whether this field is required or optional. ** All Other Processors ** Not used.
* @return companyTaxId
**/
@ApiModelProperty(value = "Company's tax identifier. This is only used for eCheck service. ** TeleCheck ** Contact your TeleCheck representative to find out whether this field is required or optional. ** All Other Processors ** Not used. ")
public String getCompanyTaxId() {
return companyTaxId;
}
public void setCompanyTaxId(String companyTaxId) {
this.companyTaxId = companyTaxId;
}
public Ptsv2paymentsBuyerInformation personalIdentification(List personalIdentification) {
this.personalIdentification = personalIdentification;
return this;
}
public Ptsv2paymentsBuyerInformation addPersonalIdentificationItem(Ptsv2paymentsBuyerInformationPersonalIdentification personalIdentificationItem) {
if (this.personalIdentification == null) {
this.personalIdentification = new ArrayList();
}
this.personalIdentification.add(personalIdentificationItem);
return this;
}
/**
* Get personalIdentification
* @return personalIdentification
**/
@ApiModelProperty(value = "")
public List getPersonalIdentification() {
return personalIdentification;
}
public void setPersonalIdentification(List personalIdentification) {
this.personalIdentification = personalIdentification;
}
public Ptsv2paymentsBuyerInformation hashedPassword(String hashedPassword) {
this.hashedPassword = hashedPassword;
return this;
}
/**
* The merchant's password that CyberSource hashes and stores as a hashed password.
* @return hashedPassword
**/
@ApiModelProperty(value = "The merchant's password that CyberSource hashes and stores as a hashed password. ")
public String getHashedPassword() {
return hashedPassword;
}
public void setHashedPassword(String hashedPassword) {
this.hashedPassword = hashedPassword;
}
public Ptsv2paymentsBuyerInformation gender(String gender) {
this.gender = gender;
return this;
}
/**
* Customer's gender. Possible values are F (female), M (male),O (other).
* @return gender
**/
@ApiModelProperty(value = "Customer's gender. Possible values are F (female), M (male),O (other).")
public String getGender() {
return gender;
}
public void setGender(String gender) {
this.gender = gender;
}
public Ptsv2paymentsBuyerInformation language(String language) {
this.language = language;
return this;
}
/**
* language setting of the user
* @return language
**/
@ApiModelProperty(value = "language setting of the user")
public String getLanguage() {
return language;
}
public void setLanguage(String language) {
this.language = language;
}
public Ptsv2paymentsBuyerInformation noteToSeller(String noteToSeller) {
this.noteToSeller = noteToSeller;
return this;
}
/**
* Note to the recipient of the funds in this transaction
* @return noteToSeller
**/
@ApiModelProperty(value = "Note to the recipient of the funds in this transaction")
public String getNoteToSeller() {
return noteToSeller;
}
public void setNoteToSeller(String noteToSeller) {
this.noteToSeller = noteToSeller;
}
public Ptsv2paymentsBuyerInformation mobilePhone(Integer mobilePhone) {
this.mobilePhone = mobilePhone;
return this;
}
/**
* Cardholder's mobile phone number. **Important** Required for Visa Secure transactions in Brazil. Do not use this request field for any other types of transactions.
* @return mobilePhone
**/
@ApiModelProperty(value = "Cardholder's mobile phone number. **Important** Required for Visa Secure transactions in Brazil. Do not use this request field for any other types of transactions. ")
public Integer getMobilePhone() {
return mobilePhone;
}
public void setMobilePhone(Integer mobilePhone) {
this.mobilePhone = mobilePhone;
}
public Ptsv2paymentsBuyerInformation walletId(String walletId) {
this.walletId = walletId;
return this;
}
/**
* The one-time identification code of the Alipay wallet user. It is scanned from the barcode that is shown by the mobile application.
* @return walletId
**/
@ApiModelProperty(value = "The one-time identification code of the Alipay wallet user. It is scanned from the barcode that is shown by the mobile application. ")
public String getWalletId() {
return walletId;
}
public void setWalletId(String walletId) {
this.walletId = walletId;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Ptsv2paymentsBuyerInformation ptsv2paymentsBuyerInformation = (Ptsv2paymentsBuyerInformation) o;
return Objects.equals(this.merchantCustomerId, ptsv2paymentsBuyerInformation.merchantCustomerId) &&
Objects.equals(this.dateOfBirth, ptsv2paymentsBuyerInformation.dateOfBirth) &&
Objects.equals(this.vatRegistrationNumber, ptsv2paymentsBuyerInformation.vatRegistrationNumber) &&
Objects.equals(this.companyTaxId, ptsv2paymentsBuyerInformation.companyTaxId) &&
Objects.equals(this.personalIdentification, ptsv2paymentsBuyerInformation.personalIdentification) &&
Objects.equals(this.hashedPassword, ptsv2paymentsBuyerInformation.hashedPassword) &&
Objects.equals(this.gender, ptsv2paymentsBuyerInformation.gender) &&
Objects.equals(this.language, ptsv2paymentsBuyerInformation.language) &&
Objects.equals(this.noteToSeller, ptsv2paymentsBuyerInformation.noteToSeller) &&
Objects.equals(this.mobilePhone, ptsv2paymentsBuyerInformation.mobilePhone) &&
Objects.equals(this.walletId, ptsv2paymentsBuyerInformation.walletId);
}
@Override
public int hashCode() {
return Objects.hash(merchantCustomerId, dateOfBirth, vatRegistrationNumber, companyTaxId, personalIdentification, hashedPassword, gender, language, noteToSeller, mobilePhone, walletId);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Ptsv2paymentsBuyerInformation {\n");
sb.append(" merchantCustomerId: ").append(toIndentedString(merchantCustomerId)).append("\n");
sb.append(" dateOfBirth: ").append(toIndentedString(dateOfBirth)).append("\n");
sb.append(" vatRegistrationNumber: ").append(toIndentedString(vatRegistrationNumber)).append("\n");
sb.append(" companyTaxId: ").append(toIndentedString(companyTaxId)).append("\n");
sb.append(" personalIdentification: ").append(toIndentedString(personalIdentification)).append("\n");
sb.append(" hashedPassword: ").append(toIndentedString(hashedPassword)).append("\n");
sb.append(" gender: ").append(toIndentedString(gender)).append("\n");
sb.append(" language: ").append(toIndentedString(language)).append("\n");
sb.append(" noteToSeller: ").append(toIndentedString(noteToSeller)).append("\n");
sb.append(" mobilePhone: ").append(toIndentedString(mobilePhone)).append("\n");
sb.append(" walletId: ").append(toIndentedString(walletId)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy