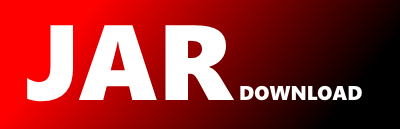
Model.SAConfigCheckout Maven / Gradle / Ivy
/*
* CyberSource Merged Spec
* All CyberSource API specs merged together. These are available at https://developer.cybersource.com/api/reference/api-reference.html
*
* OpenAPI spec version: 0.0.1
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package Model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
/**
* SAConfigCheckout
*/
public class SAConfigCheckout {
@SerializedName("displayTaxAmount")
private Boolean displayTaxAmount = null;
@SerializedName("templateType")
private String templateType = null;
@SerializedName("returnToMerchantSiteUrl")
private String returnToMerchantSiteUrl = null;
public SAConfigCheckout displayTaxAmount(Boolean displayTaxAmount) {
this.displayTaxAmount = displayTaxAmount;
return this;
}
/**
* Toggles whether or not the tax amount is displayed on the Hosted Checkout.
* @return displayTaxAmount
**/
@ApiModelProperty(value = "Toggles whether or not the tax amount is displayed on the Hosted Checkout.")
public Boolean isDisplayTaxAmount() {
return displayTaxAmount;
}
public void setDisplayTaxAmount(Boolean displayTaxAmount) {
this.displayTaxAmount = displayTaxAmount;
}
public SAConfigCheckout templateType(String templateType) {
this.templateType = templateType;
return this;
}
/**
* Specifies whether the Hosted Checkout is displayed as a single page form or multi page checkout. Valid values: `multi` `single`
* @return templateType
**/
@ApiModelProperty(value = "Specifies whether the Hosted Checkout is displayed as a single page form or multi page checkout. Valid values: `multi` `single` ")
public String getTemplateType() {
return templateType;
}
public void setTemplateType(String templateType) {
this.templateType = templateType;
}
public SAConfigCheckout returnToMerchantSiteUrl(String returnToMerchantSiteUrl) {
this.returnToMerchantSiteUrl = returnToMerchantSiteUrl;
return this;
}
/**
* URL of the website linked to from the Secure Acceptance receipt page. Only used if the profile does not have custom receipt pages configured.
* @return returnToMerchantSiteUrl
**/
@ApiModelProperty(value = "URL of the website linked to from the Secure Acceptance receipt page. Only used if the profile does not have custom receipt pages configured.")
public String getReturnToMerchantSiteUrl() {
return returnToMerchantSiteUrl;
}
public void setReturnToMerchantSiteUrl(String returnToMerchantSiteUrl) {
this.returnToMerchantSiteUrl = returnToMerchantSiteUrl;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
SAConfigCheckout saConfigCheckout = (SAConfigCheckout) o;
return Objects.equals(this.displayTaxAmount, saConfigCheckout.displayTaxAmount) &&
Objects.equals(this.templateType, saConfigCheckout.templateType) &&
Objects.equals(this.returnToMerchantSiteUrl, saConfigCheckout.returnToMerchantSiteUrl);
}
@Override
public int hashCode() {
return Objects.hash(displayTaxAmount, templateType, returnToMerchantSiteUrl);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class SAConfigCheckout {\n");
sb.append(" displayTaxAmount: ").append(toIndentedString(displayTaxAmount)).append("\n");
sb.append(" templateType: ").append(toIndentedString(templateType)).append("\n");
sb.append(" returnToMerchantSiteUrl: ").append(toIndentedString(returnToMerchantSiteUrl)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy