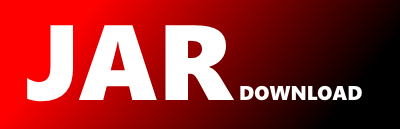
Model.SAConfigNotificationsCustomerNotifications Maven / Gradle / Ivy
/*
* CyberSource Merged Spec
* All CyberSource API specs merged together. These are available at https://developer.cybersource.com/api/reference/api-reference.html
*
* OpenAPI spec version: 0.0.1
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package Model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
/**
* Features relating to notifications being sent directly to the payer using the Hosted Checkout.
*/
@ApiModel(description = "Features relating to notifications being sent directly to the payer using the Hosted Checkout.")
public class SAConfigNotificationsCustomerNotifications {
@SerializedName("customReceiptPageEnabled")
private Boolean customReceiptPageEnabled = null;
@SerializedName("receiptEmailAddress")
private String receiptEmailAddress = null;
@SerializedName("customerReceiptEmailEnabled")
private Boolean customerReceiptEmailEnabled = null;
@SerializedName("customCancelPage")
private String customCancelPage = null;
@SerializedName("customReceiptPage")
private String customReceiptPage = null;
@SerializedName("customCancelPageEnabled")
private Boolean customCancelPageEnabled = null;
@SerializedName("notificationReceiptEmailEnabled")
private Boolean notificationReceiptEmailEnabled = null;
public SAConfigNotificationsCustomerNotifications customReceiptPageEnabled(Boolean customReceiptPageEnabled) {
this.customReceiptPageEnabled = customReceiptPageEnabled;
return this;
}
/**
* Toggles the custom receipt page, where merchants can receive the results of the transaction and display appropriate messaging. Usually set by web developers integrating to Secure Acceptance.
* @return customReceiptPageEnabled
**/
@ApiModelProperty(value = "Toggles the custom receipt page, where merchants can receive the results of the transaction and display appropriate messaging. Usually set by web developers integrating to Secure Acceptance.")
public Boolean isCustomReceiptPageEnabled() {
return customReceiptPageEnabled;
}
public void setCustomReceiptPageEnabled(Boolean customReceiptPageEnabled) {
this.customReceiptPageEnabled = customReceiptPageEnabled;
}
public SAConfigNotificationsCustomerNotifications receiptEmailAddress(String receiptEmailAddress) {
this.receiptEmailAddress = receiptEmailAddress;
return this;
}
/**
* Email address where a copy of the payer's receipt email is sent, when notificationReceiptEmailEnabled is true.
* @return receiptEmailAddress
**/
@ApiModelProperty(value = "Email address where a copy of the payer's receipt email is sent, when notificationReceiptEmailEnabled is true.")
public String getReceiptEmailAddress() {
return receiptEmailAddress;
}
public void setReceiptEmailAddress(String receiptEmailAddress) {
this.receiptEmailAddress = receiptEmailAddress;
}
public SAConfigNotificationsCustomerNotifications customerReceiptEmailEnabled(Boolean customerReceiptEmailEnabled) {
this.customerReceiptEmailEnabled = customerReceiptEmailEnabled;
return this;
}
/**
* Toggles an email receipt sent to the payer's email address on payment success.
* @return customerReceiptEmailEnabled
**/
@ApiModelProperty(value = "Toggles an email receipt sent to the payer's email address on payment success.")
public Boolean isCustomerReceiptEmailEnabled() {
return customerReceiptEmailEnabled;
}
public void setCustomerReceiptEmailEnabled(Boolean customerReceiptEmailEnabled) {
this.customerReceiptEmailEnabled = customerReceiptEmailEnabled;
}
public SAConfigNotificationsCustomerNotifications customCancelPage(String customCancelPage) {
this.customCancelPage = customCancelPage;
return this;
}
/**
* URL to which transaction results are POSTed when the payer clicks 'Cancel' on the Hosted Checkout. Triggered when customCancelPageEnabled is true. Usually set by web developers integrating to Secure Acceptance.
* @return customCancelPage
**/
@ApiModelProperty(value = "URL to which transaction results are POSTed when the payer clicks 'Cancel' on the Hosted Checkout. Triggered when customCancelPageEnabled is true. Usually set by web developers integrating to Secure Acceptance.")
public String getCustomCancelPage() {
return customCancelPage;
}
public void setCustomCancelPage(String customCancelPage) {
this.customCancelPage = customCancelPage;
}
public SAConfigNotificationsCustomerNotifications customReceiptPage(String customReceiptPage) {
this.customReceiptPage = customReceiptPage;
return this;
}
/**
* URL to which transaction results are POSTed when the payer requests a payment on the Hosted Checkout. Triggered when customCancelPageEnabled is true. Usually set by web developers integrating to Secure Acceptance.
* @return customReceiptPage
**/
@ApiModelProperty(value = "URL to which transaction results are POSTed when the payer requests a payment on the Hosted Checkout. Triggered when customCancelPageEnabled is true. Usually set by web developers integrating to Secure Acceptance.")
public String getCustomReceiptPage() {
return customReceiptPage;
}
public void setCustomReceiptPage(String customReceiptPage) {
this.customReceiptPage = customReceiptPage;
}
public SAConfigNotificationsCustomerNotifications customCancelPageEnabled(Boolean customCancelPageEnabled) {
this.customCancelPageEnabled = customCancelPageEnabled;
return this;
}
/**
* Toggles the custom cancel page, where merchants can receive notice that the payer has canceled, and display appropriate messaging and direction. Usually set by web developers integrating to Secure Acceptance.
* @return customCancelPageEnabled
**/
@ApiModelProperty(value = "Toggles the custom cancel page, where merchants can receive notice that the payer has canceled, and display appropriate messaging and direction. Usually set by web developers integrating to Secure Acceptance.")
public Boolean isCustomCancelPageEnabled() {
return customCancelPageEnabled;
}
public void setCustomCancelPageEnabled(Boolean customCancelPageEnabled) {
this.customCancelPageEnabled = customCancelPageEnabled;
}
public SAConfigNotificationsCustomerNotifications notificationReceiptEmailEnabled(Boolean notificationReceiptEmailEnabled) {
this.notificationReceiptEmailEnabled = notificationReceiptEmailEnabled;
return this;
}
/**
* Toggles whether merchant receives a copy of the payer's receipt email.
* @return notificationReceiptEmailEnabled
**/
@ApiModelProperty(value = "Toggles whether merchant receives a copy of the payer's receipt email.")
public Boolean isNotificationReceiptEmailEnabled() {
return notificationReceiptEmailEnabled;
}
public void setNotificationReceiptEmailEnabled(Boolean notificationReceiptEmailEnabled) {
this.notificationReceiptEmailEnabled = notificationReceiptEmailEnabled;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
SAConfigNotificationsCustomerNotifications saConfigNotificationsCustomerNotifications = (SAConfigNotificationsCustomerNotifications) o;
return Objects.equals(this.customReceiptPageEnabled, saConfigNotificationsCustomerNotifications.customReceiptPageEnabled) &&
Objects.equals(this.receiptEmailAddress, saConfigNotificationsCustomerNotifications.receiptEmailAddress) &&
Objects.equals(this.customerReceiptEmailEnabled, saConfigNotificationsCustomerNotifications.customerReceiptEmailEnabled) &&
Objects.equals(this.customCancelPage, saConfigNotificationsCustomerNotifications.customCancelPage) &&
Objects.equals(this.customReceiptPage, saConfigNotificationsCustomerNotifications.customReceiptPage) &&
Objects.equals(this.customCancelPageEnabled, saConfigNotificationsCustomerNotifications.customCancelPageEnabled) &&
Objects.equals(this.notificationReceiptEmailEnabled, saConfigNotificationsCustomerNotifications.notificationReceiptEmailEnabled);
}
@Override
public int hashCode() {
return Objects.hash(customReceiptPageEnabled, receiptEmailAddress, customerReceiptEmailEnabled, customCancelPage, customReceiptPage, customCancelPageEnabled, notificationReceiptEmailEnabled);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class SAConfigNotificationsCustomerNotifications {\n");
sb.append(" customReceiptPageEnabled: ").append(toIndentedString(customReceiptPageEnabled)).append("\n");
sb.append(" receiptEmailAddress: ").append(toIndentedString(receiptEmailAddress)).append("\n");
sb.append(" customerReceiptEmailEnabled: ").append(toIndentedString(customerReceiptEmailEnabled)).append("\n");
sb.append(" customCancelPage: ").append(toIndentedString(customCancelPage)).append("\n");
sb.append(" customReceiptPage: ").append(toIndentedString(customReceiptPage)).append("\n");
sb.append(" customCancelPageEnabled: ").append(toIndentedString(customCancelPageEnabled)).append("\n");
sb.append(" notificationReceiptEmailEnabled: ").append(toIndentedString(notificationReceiptEmailEnabled)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy