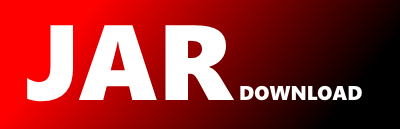
Model.InlineResponse2006Billing Maven / Gradle / Ivy
The newest version!
/*
* CyberSource Merged Spec
* All CyberSource API specs merged together. These are available at https://developer.cybersource.com/api/reference/api-reference.html
*
* OpenAPI spec version: 0.0.1
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package Model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
/**
* InlineResponse2006Billing
*/
public class InlineResponse2006Billing {
@SerializedName("nan")
private Integer nan = null;
@SerializedName("ned")
private Integer ned = null;
@SerializedName("acl")
private Integer acl = null;
@SerializedName("cch")
private Integer cch = null;
public InlineResponse2006Billing nan(Integer nan) {
this.nan = nan;
return this;
}
/**
* Get nan
* @return nan
**/
@ApiModelProperty(example = "1", value = "")
public Integer getNan() {
return nan;
}
public void setNan(Integer nan) {
this.nan = nan;
}
public InlineResponse2006Billing ned(Integer ned) {
this.ned = ned;
return this;
}
/**
* Get ned
* @return ned
**/
@ApiModelProperty(example = "1", value = "")
public Integer getNed() {
return ned;
}
public void setNed(Integer ned) {
this.ned = ned;
}
public InlineResponse2006Billing acl(Integer acl) {
this.acl = acl;
return this;
}
/**
* Get acl
* @return acl
**/
@ApiModelProperty(example = "1", value = "")
public Integer getAcl() {
return acl;
}
public void setAcl(Integer acl) {
this.acl = acl;
}
public InlineResponse2006Billing cch(Integer cch) {
this.cch = cch;
return this;
}
/**
* Get cch
* @return cch
**/
@ApiModelProperty(example = "1", value = "")
public Integer getCch() {
return cch;
}
public void setCch(Integer cch) {
this.cch = cch;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
InlineResponse2006Billing inlineResponse2006Billing = (InlineResponse2006Billing) o;
return Objects.equals(this.nan, inlineResponse2006Billing.nan) &&
Objects.equals(this.ned, inlineResponse2006Billing.ned) &&
Objects.equals(this.acl, inlineResponse2006Billing.acl) &&
Objects.equals(this.cch, inlineResponse2006Billing.cch);
}
@Override
public int hashCode() {
return Objects.hash(nan, ned, acl, cch);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class InlineResponse2006Billing {\n");
sb.append(" nan: ").append(toIndentedString(nan)).append("\n");
sb.append(" ned: ").append(toIndentedString(ned)).append("\n");
sb.append(" acl: ").append(toIndentedString(acl)).append("\n");
sb.append(" cch: ").append(toIndentedString(cch)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}