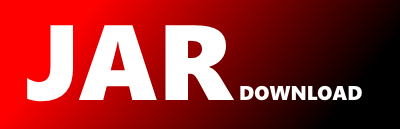
Model.Ptsv2paymentsidrefundsPaymentInformationBankAccount Maven / Gradle / Ivy
The newest version!
/*
* CyberSource Merged Spec
* All CyberSource API specs merged together. These are available at https://developer.cybersource.com/api/reference/api-reference.html
*
* OpenAPI spec version: 0.0.1
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package Model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
/**
* Ptsv2paymentsidrefundsPaymentInformationBankAccount
*/
public class Ptsv2paymentsidrefundsPaymentInformationBankAccount {
@SerializedName("type")
private String type = null;
@SerializedName("number")
private String number = null;
@SerializedName("encoderId")
private String encoderId = null;
@SerializedName("checkNumber")
private String checkNumber = null;
@SerializedName("checkImageReferenceNumber")
private String checkImageReferenceNumber = null;
public Ptsv2paymentsidrefundsPaymentInformationBankAccount type(String type) {
this.type = type;
return this;
}
/**
* Account type. Possible values: - **C**: Checking. - **G**: General ledger. This value is supported only on Wells Fargo ACH. - **S**: Savings (U.S. dollars only). - **X**: Corporate checking (U.S. dollars only).
* @return type
**/
@ApiModelProperty(value = "Account type. Possible values: - **C**: Checking. - **G**: General ledger. This value is supported only on Wells Fargo ACH. - **S**: Savings (U.S. dollars only). - **X**: Corporate checking (U.S. dollars only). ")
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
public Ptsv2paymentsidrefundsPaymentInformationBankAccount number(String number) {
this.number = number;
return this;
}
/**
* Account number. When processing encoded account numbers, use this field for the encoded account number.
* @return number
**/
@ApiModelProperty(value = "Account number. When processing encoded account numbers, use this field for the encoded account number. ")
public String getNumber() {
return number;
}
public void setNumber(String number) {
this.number = number;
}
public Ptsv2paymentsidrefundsPaymentInformationBankAccount encoderId(String encoderId) {
this.encoderId = encoderId;
return this;
}
/**
* Identifier for the bank that provided the customer's encoded account number. To obtain the bank identifier, contact your processor.
* @return encoderId
**/
@ApiModelProperty(value = "Identifier for the bank that provided the customer's encoded account number. To obtain the bank identifier, contact your processor. ")
public String getEncoderId() {
return encoderId;
}
public void setEncoderId(String encoderId) {
this.encoderId = encoderId;
}
public Ptsv2paymentsidrefundsPaymentInformationBankAccount checkNumber(String checkNumber) {
this.checkNumber = checkNumber;
return this;
}
/**
* Check number. Chase Paymentech Solutions - Optional. CyberSource ACH Service - Not used. RBS WorldPay Atlanta - Optional on debits. Required on credits. TeleCheck - Strongly recommended on debit requests. Optional on credits.
* @return checkNumber
**/
@ApiModelProperty(value = "Check number. Chase Paymentech Solutions - Optional. CyberSource ACH Service - Not used. RBS WorldPay Atlanta - Optional on debits. Required on credits. TeleCheck - Strongly recommended on debit requests. Optional on credits. ")
public String getCheckNumber() {
return checkNumber;
}
public void setCheckNumber(String checkNumber) {
this.checkNumber = checkNumber;
}
public Ptsv2paymentsidrefundsPaymentInformationBankAccount checkImageReferenceNumber(String checkImageReferenceNumber) {
this.checkImageReferenceNumber = checkImageReferenceNumber;
return this;
}
/**
* Image reference number associated with the check. You cannot include any special characters.
* @return checkImageReferenceNumber
**/
@ApiModelProperty(value = "Image reference number associated with the check. You cannot include any special characters. ")
public String getCheckImageReferenceNumber() {
return checkImageReferenceNumber;
}
public void setCheckImageReferenceNumber(String checkImageReferenceNumber) {
this.checkImageReferenceNumber = checkImageReferenceNumber;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Ptsv2paymentsidrefundsPaymentInformationBankAccount ptsv2paymentsidrefundsPaymentInformationBankAccount = (Ptsv2paymentsidrefundsPaymentInformationBankAccount) o;
return Objects.equals(this.type, ptsv2paymentsidrefundsPaymentInformationBankAccount.type) &&
Objects.equals(this.number, ptsv2paymentsidrefundsPaymentInformationBankAccount.number) &&
Objects.equals(this.encoderId, ptsv2paymentsidrefundsPaymentInformationBankAccount.encoderId) &&
Objects.equals(this.checkNumber, ptsv2paymentsidrefundsPaymentInformationBankAccount.checkNumber) &&
Objects.equals(this.checkImageReferenceNumber, ptsv2paymentsidrefundsPaymentInformationBankAccount.checkImageReferenceNumber);
}
@Override
public int hashCode() {
return Objects.hash(type, number, encoderId, checkNumber, checkImageReferenceNumber);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Ptsv2paymentsidrefundsPaymentInformationBankAccount {\n");
sb.append(" type: ").append(toIndentedString(type)).append("\n");
sb.append(" number: ").append(toIndentedString(number)).append("\n");
sb.append(" encoderId: ").append(toIndentedString(encoderId)).append("\n");
sb.append(" checkNumber: ").append(toIndentedString(checkNumber)).append("\n");
sb.append(" checkImageReferenceNumber: ").append(toIndentedString(checkImageReferenceNumber)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy