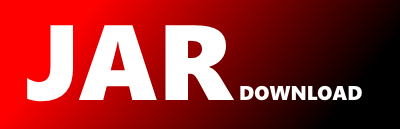
com.cybersource.flex.sdk.FlexService Maven / Gradle / Ivy
/**
* Copyright (c) 2017, 2019 by CyberSource
*/
package com.cybersource.flex.sdk;
import com.cybersource.flex.sdk.impl.ConnectedHttpClient;
import java.net.Proxy;
import java.security.PublicKey;
import java.util.Map;
public interface FlexService {
CaptureContext fromJwt(String captureContext);
TransientToken fromJwt(String cc, String tt);
CaptureContext createCaptureContext(CaptureContextIntent intent);
TransientToken createTransientToken(CaptureContext captureContext, Map data);
/**
* IF YOUR AIM IS TO ACHIEVE SAQ-A COMPLIANCE, USE THIS METHOD ON CONSUMER DEVICE ONLY.
*
* @param captureContext
* @param data to be tokenized
* @return Transient Token representing data stored on Secure VISA server.
*/
default TransientToken createTransientToken(final String captureContext, final Map data) {
final CaptureContext cc = fromJwt(captureContext);
return createTransientToken(cc, data);
}
/**
* Returns long living Flex API public key. This key can be used to validate CaptureContext signature. Some keys
* are distributed with this SDK. In future FlexService might connect to VISA to retrieve new keys (i.e. provisioned
* due to key rotation requirements).
*
* @param kid long term key name
* @return long living public Key or null if such key does not exist.
*/
PublicKey flexPublicKey(String kid);
static FlexService offline() {
return new FlexServiceImpl(null, null);
}
static FlexService standardClient(final Credentials credentials) {
return new Builder(credentials).build();
}
static Builder clientBuilder(final Credentials credentials) {
return new Builder(credentials);
}
final class Builder {
private final Credentials credentials;
private HttpClient httpClient = null;
private Proxy proxy = Proxy.NO_PROXY;
private Builder(Credentials credentials) {
this.credentials = credentials;
}
public Builder proxy(Proxy proxy) {
if (httpClient != null) {
throw new IllegalStateException("Cannot set proxy when http client is already set.");
}
this.proxy = proxy;
return this;
}
public Builder httpClient(HttpClient httpClient) {
if (proxy != Proxy.NO_PROXY) { // fine to compare references here
throw new IllegalStateException("Cannot set http client when proxy is already set.");
}
this.httpClient = httpClient;
return this;
}
public FlexService build() {
if (httpClient == null) {
httpClient = new ConnectedHttpClient(proxy);
}
return new FlexServiceImpl(httpClient, credentials);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy