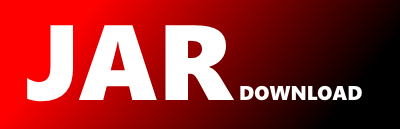
com.cyc.kb.client.KbFunctionImpl Maven / Gradle / Ivy
Show all versions of cyc-kb-client Show documentation
package com.cyc.kb.client;
/*
* #%L
* File: KbFunctionImpl.java
* Project: KB Client
* %%
* Copyright (C) 2013 - 2017 Cycorp, Inc
* %%
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* #L%
*/
import com.cyc.base.cycobject.CycList;
import com.cyc.base.cycobject.CycObject;
import com.cyc.base.cycobject.DenotationalTerm;
import com.cyc.base.cycobject.FormulaSentence;
import com.cyc.base.cycobject.Guid;
import com.cyc.base.cycobject.Nart;
import com.cyc.base.exception.CycConnectionException;
import com.cyc.baseclient.cycobject.CycArrayList;
import com.cyc.baseclient.cycobject.CycConstantImpl;
import com.cyc.baseclient.cycobject.NartImpl;
import com.cyc.baseclient.cycobject.NautImpl;
import com.cyc.kb.Context;
import com.cyc.kb.DefaultContext;
import com.cyc.kb.KbCollection;
import com.cyc.kb.KbFunction;
import com.cyc.kb.KbObject;
import com.cyc.kb.KbStatus;
import com.cyc.kb.client.config.KbConfiguration;
import com.cyc.kb.exception.CreateException;
import com.cyc.kb.exception.InvalidNameException;
import com.cyc.kb.exception.KbException;
import com.cyc.kb.exception.KbObjectNotFoundException;
import com.cyc.kb.exception.KbRuntimeException;
import com.cyc.kb.exception.KbTypeConflictException;
import com.cyc.kb.exception.KbTypeException;
import java.util.Collection;
/**
*
* A KBFunction
object is a facade for a #$Function-Denotational
* in the Cyc KB.
*
* A N-ary function is a many-one relation, between the N-tuple of the domain elements to
* a range element. Functions can be used to create "non-atomic terms"
* or #$CycLClosedNonAtomicTerm.
*
* The class provides a method to create new functional terms, which can in turn be used in
* other assertions, just as constants are.
*
* A new function is by default made a #$ReifiableFunction
in the underlying
* Cyc Knowledge Base. A future version of the API will support un-reifiable functions.
*
* @param type of CycObject core
*
* @author Vijay Raj
* @version $Id: KbFunctionImpl.java 173082 2017-07-28 15:36:55Z nwinant $
*/
// @TODO: Add examples
public class KbFunctionImpl extends RelationImpl implements KbFunction {
//private static final Logger LOG = LoggerFactory.getLogger(KbFunctionImpl.class.getName());
private static final DenotationalTerm TYPE_CORE =
new CycConstantImpl("Function-Denotational", new Guid("bd5c40b0-9c29-11b1-9dad-c379636f7270"));
private Boolean isUnreifiable = null;
static DenotationalTerm getClassTypeCore() {
return TYPE_CORE;
}
/**
* Not part of the KB API. This default constructor only has the effect of
* ensuring that there is access to a Cyc server.
*/
KbFunctionImpl() {
super ();
}
/**
* Not part of the KB API. An implementation-dependent constructor.
*
* It is used when the result of query is a CycObject and is known to be or
* requested to be cast as an instance of KBFunction.
*
* @param cycObject the CycObject wrapped by KBFunction
. The constructor
* verifies that the CycObject is an instance of #$Function-Denotational
*
* @throws KbTypeException if cycObject is not or could not be made
* an instance of #$Function-Denotational
*/
KbFunctionImpl(DenotationalTerm cycObject) throws KbTypeException {
super(cycObject);
}
/**
* This not part of the public, supported KB API. finds or creates an instance of #$Function-Denotational represented
* by funcStr in the underlying KB
*
*
* @param funcStr the string representing an #$Function-Denotational in the KB
*
* @throws CreateException if the #$Function-Denotational represented by funcStr is not found
* and could not be created
* @throws KbTypeException if the term represented by funcStr is not an instance
* of #$Function-Denotational and cannot be made into one.
*/
KbFunctionImpl(String funcStr) throws KbTypeException, CreateException {
super(funcStr);
}
/**
* This not part of the public, supported KB API. finds or creates; or finds an instance of #$Function-Denotational
* represented by funcStr in the underlying KB based on input ENUM
*
*
* @param funcStr the string representing an instance of #$Function-Denotational in the KB
* @param lookup the enum to specify LookupType: FIND or FIND_OR_CREATE
*
* @throws CreateException
* @throws KbTypeException
*
* @throws KbObjectNotFoundException if the #$Function-Denotational represented by funcStr
* is not found and could not be created
* @throws InvalidNameException if the string funcStr does not conform to Cyc constant-naming
* conventions
*
* @throws KbTypeException if the term represented by funcStr is not an instance of #$Function-Denotational and lookup is
* set to find only {@link LookupType#FIND}
* @throws KbTypeConflictException if the term represented by funcStr is not an instance of #$Function-Denotational,
* and lookup is set to find or create; and if the term cannot be made an instance #$Function-Denotational by asserting
* new knowledge.
*/
KbFunctionImpl(String funcStr, LookupType lookup) throws KbTypeException, CreateException {
super(funcStr, lookup);
}
protected KbFunctionImpl (DefaultContext c, KbFunction func) {
super();
this.setCore(func);
}
/**
* Get the
* KBFunction
with the name
* nameOrId
. Throws exceptions if there is no KB term by that
* name, or if it is not already an instance of #$Function-Denotational.
*
* @param nameOrId the string representation or the HLID of the #$Function-Denotational
*
* @return a new KBFunction
*
* @throws KbTypeException
* @throws CreateException
*/
public static KbFunctionImpl get(String nameOrId) throws KbTypeException, CreateException {
return KbObjectImplFactory.get(nameOrId, KbFunctionImpl.class);
}
/**
* Get the
* KBFunction
object that corresponds to
* cycObject
. Throws exceptions if the object isn't in the KB, or if
* it's not already an instance of
* #$Function-Denotational
.
*
* @param cycObject the CycObject wrapped by KBFunction. The method
* verifies that the CycObject is an instance of #$Function-Denotational
*
* @return a new KBFunction
*
* @throws CreateException
* @throws KbTypeException
*/
@Deprecated
public static KbFunctionImpl get(CycObject cycObject) throws KbTypeException, CreateException {
return KbObjectImplFactory.get(cycObject, KbFunctionImpl.class);
}
/**
* Find or create a
* KBFunction
object named
* nameOrId
. If no object exists in the KB with the name
* nameOrId
, one will be created, and it will be asserted to be
* an instance of
* #$Function-Denotational
. If there is already an object in the KB called
* nameOrId
, and it is already a
* #$Function-Denotational
, it will be returned. If it is not already a
* #$Function-Denotational
, but can be made into one by addition of
* assertions to the KB, such assertions will be made, and the object will be
* returned. If the object in the KB cannot be turned into a
* #$Function-Denotational
by adding assertions (i.e. some existing
* assertion prevents it from being a
* #$Function-Denotational
), a
* KBTypeConflictException
will be thrown.
*
* @param nameOrId the string representation or the HLID of the #$Function-Denotational
*
* @return a new KBFunction
*
* @throws KbTypeException
* @throws CreateException
*/
public static KbFunctionImpl findOrCreate(String nameOrId) throws CreateException, KbTypeException {
return KbObjectImplFactory.findOrCreate(nameOrId, KbFunctionImpl.class);
}
/**
* Find or create a KBFunction object from
* cycObject
. If
* cycObject
is already a
* #$Function-Denotational
, an appropriate
* KBFunction
object will be returned. If
* object
is not already a
* #$Function-Denotational
, but can be made into one by addition of
* assertions to the KB, such assertions will be made, and the relevant object
* will be returned. If
* cycObject
cannot be turned into a
* #$Function-Denotational
by adding assertions (i.e. some existing
* assertion prevents it from being a
* #$Function-Denotational
, a
* KBTypeConflictException
will be thrown.
*
* @param cycObject the CycObject wrapped by KBFunction. The method
* verifies that the CycObject is an #$Function-Denotational
*
* @return a new KBFunction
*
* @throws KbTypeException
* @throws CreateException
*/
@Deprecated
public static KbFunctionImpl findOrCreate(CycObject cycObject) throws CreateException, KbTypeException {
return KbObjectImplFactory.findOrCreate(cycObject, KbFunctionImpl.class);
}
/**
* Find or create a
* KBFunction
object named
* nameOrId
, and also make it an instance of
* constraintCol
in the default context specified by
* {@link KBAPIDefaultContext#forAssertion()}. If no object
* exists in the KB with the name
* nameOrId
, one will be created, and it will be asserted to be
* an instance of both
* #$Function-Denotational
and
* constraintCol
. If there is already an object in the
* KB called
* nameOrId
, and it is already both a
* #$Function-Denotational
and a
* constraintCol
, it will be returned. If it is not
* already both a
* #$Function-Denotational
and a
* constraintCol
, but can be made so by addition of
* assertions to the KB, such assertions will be made, and the object will be
* returned. If the object in the KB cannot be turned into both a
* #$Function-Denotational
and a
* constraintCol
by adding assertions, a
* KBTypeConflictException
will be thrown.
*
* @param nameOrId the string representation or the HLID of the #$Function-Denotational
* @param constraintCol the collection that this #$Function-Denotational will instantiate
*
* @return a new KBFunction
*
* @throws KbTypeException
* @throws CreateException
*/
public static KbFunctionImpl findOrCreate(String nameOrId, KbCollection constraintCol) throws CreateException, KbTypeException {
return KbObjectImplFactory.findOrCreate(nameOrId, constraintCol, KbFunctionImpl.class);
}
/**
* Find or create a
* KBFunction
object named
* nameOrId
, and also make it an instance of
* constraintCol
in the default context specified by
* {@link KBAPIDefaultContext#forAssertion()}. If no object
* exists in the KB with the name
* nameOrId
, one will be created, and it will be asserted to be
* an instance of both
* #$Function-Denotational
and
* constraintCol
. If there is already an object in the
* KB called
* nameOrId
, and it is already both a
* #$Function-Denotational
and a
* constraintCol
, it will be returned. If it is not
* already both a
* #$Function-Denotational
and a
* constraintCol
, but can be made so by addition of
* assertions to the KB, such assertions will be made, and the object will be
* returned. If the object in the KB cannot be turned into both a
* #$Function-Denotational
and a
* constraintCol
by adding assertions, a
* KBTypeConflictException
will be thrown.
*
* @param nameOrId the string representation or the HLID of the #$Function-Denotational
* @param constraintColStr the string representation of the collection that
* this #$Function-Denotational will instantiate
*
* @return a new KBFunction
*
* @throws KbTypeException
* @throws CreateException
*/
public static KbFunctionImpl findOrCreate(String nameOrId, String constraintColStr) throws CreateException, KbTypeException {
return KbObjectImplFactory.findOrCreate(nameOrId, constraintColStr, KbFunctionImpl.class);
}
/**
* Find or create a
* KBFunction
object named
* nameOrId
, and also make it an instance of
* constraintCol
in
* ctx
. If no object exists in the KB with the name
* nameOrId
, one will be created, and it will be asserted to be
* an instance of both
* #$Function-Denotational
and
* constraintCol
. If there is already an object in the
* KB called
* nameOrId
, and it is already both a
* #$Function-Denotational
and a
* constraintCol
, it will be returned. If it is not
* already both a
* #$Function-Denotational
and a
* constraintCol
, but can be made so by addition of
* assertions to the KB, such assertions will be made, and the object will be
* returned. If the object in the KB cannot be turned into both a
* #$Function-Denotational
and a
* constraintCol
by adding assertions, a
* KBTypeConflictException
will be thrown.
*
* @param nameOrId the string representation or the HLID of the #$Function-Denotational
* @param constraintCol the collection that this #$Function-Denotational will instantiate
* @param ctx the context in which the resulting object must be an instance of
* constraintCol
*
* @return a new KBFunction
*
* @throws KbTypeException
* @throws CreateException
*/
public static KbFunctionImpl findOrCreate(String nameOrId, KbCollection constraintCol, Context ctx)
throws CreateException, KbTypeException {
return KbObjectImplFactory.findOrCreate(nameOrId, constraintCol, ctx, KbFunctionImpl.class);
}
/**
* Find or create a
* KBFunction
object named
* nameOrId
, and also make it an instance of
* constraintCol
in
* ctx
. If no object exists in the KB with the name
* nameOrId
, one will be created, and it will be asserted to be
* an instance of both
* #$Function-Denotational
and
* constraintCol
. If there is already an object in the
* KB called
* nameOrId
, and it is already both a
* #$Function-Denotational
and a
* constraintCol
, it will be returned. If it is not
* already both a
* #$Function-Denotational
and a
* constraintCol
, but can be made so by addition of
* assertions to the KB, such assertions will be made, and the object will be
* returned. If the object in the KB cannot be turned into both a
* #$Function-Denotational
and a
* constraintCol
by adding assertions, a
* KBTypeConflictException
will be thrown.
*
* @param nameOrId the string representation or the HLID of the term
* @param constraintColStr the string representation of the collection that
* this #$Function-Denotational will instantiate
* @param ctxStr the context in which the resulting object must be an instance of
* constraintCol
*
* @return a new KBFunction
*
* @throws KbTypeException
* @throws CreateException
*/
public static KbFunctionImpl findOrCreate(String nameOrId, String constraintColStr, String ctxStr) throws CreateException, KbTypeException {
return KbObjectImplFactory.findOrCreate(nameOrId, constraintColStr, ctxStr, KbFunctionImpl.class);
}
/**
* Checks whether entity exists in KB and is an instance of #$Function-Denotational. If
* false, {@link #getStatus(String)} may yield more information. This method
* is equivalent to
* getStatus(nameOrId).equals(KBStatus.EXISTS_AS_TYPE)
.
*
* @param nameOrId either the name or HL ID of an entity in the KB
* @return true
if entity exists in KB and is an instance of
* #$Function-Denotational
*/
public static boolean existsAsType(String nameOrId) {
return getStatus(nameOrId).equals(KbStatus.EXISTS_AS_TYPE);
}
/**
* Checks whether entity exists in KB and is an instance of #$Function-Denotational. If
* false, {@link #getStatus(CycObject)} may yield more information. This
* method is equivalent to
* getStatus(object).equals(KBStatus.EXISTS_AS_TYPE)
.
*
* @param cycObject the CycObject representation of a KB entity
* @return true
if entity exists in KB and is an instance of
* #$Function-Denotational
*/
public static boolean existsAsType(CycObject cycObject) {
return getStatus(cycObject).equals(KbStatus.EXISTS_AS_TYPE);
}
/**
* Returns an KBStatus enum which describes whether
* nameOrId
exists in the KB and is an instance of
* #$Function-Denotational
.
*
* @param nameOrId either the name or HL ID of an entity in the KB
* @return an enum describing the existential status of the entity in the KB
*/
public static KbStatus getStatus(String nameOrId) {
return KbObjectImplFactory.getStatus(nameOrId, KbFunctionImpl.class);
}
/**
* Returns an KBStatus enum which describes whether
* cycObject
exists in the KB and is an instance of
* #$Function-Denotational
.
*
* @param cycObject the CycObject representation of a KB entity
* @return an enum describing the existential status of the entity in the KB
*/
public static KbStatus getStatus(CycObject cycObject) {
return KbObjectImplFactory.getStatus(cycObject, KbFunctionImpl.class);
}
@Override
@SuppressWarnings("deprecation")
public O findOrCreateFunctionalTerm(Class retType, Object... args)
throws KbTypeException, CreateException {
validateArgArity(args);
try {
final FormulaSentence fs = SentenceImpl.convertKBObjectArrayToCycFormulaSentence(args);
final CycList