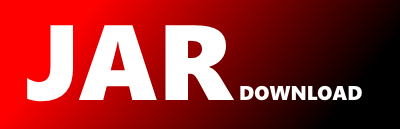
com.cyc.xml.query.ObjectFactory Maven / Gradle / Ivy
Show all versions of cyc-query-client Show documentation
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.2.7
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2017.07.20 at 01:27:16 PM CDT
//
package com.cyc.xml.query;
import javax.xml.bind.JAXBElement;
import javax.xml.bind.annotation.XmlElementDecl;
import javax.xml.bind.annotation.XmlRegistry;
import javax.xml.namespace.QName;
/**
* This object contains factory methods for each
* Java content interface and Java element interface
* generated in the com.cyc.xml.query package.
* An ObjectFactory allows you to programatically
* construct new instances of the Java representation
* for XML content. The Java representation of XML
* content can consist of schema derived interfaces
* and classes representing the binding of schema
* type definitions, element declarations and model
* groups. Factory methods for each of these are
* provided in this class.
*
*/
@XmlRegistry
public class ObjectFactory {
private final static QName _ValidationRequired_QNAME = new QName("http://www.opencyc.org/xml/formulaTemplate/", "validationRequired");
private final static QName _SingleEntry_QNAME = new QName("http://www.opencyc.org/xml/formulaTemplate/", "singleEntry");
private final static QName _Cycl_QNAME = new QName("http://www.opencyc.org/xml/proofView/", "cycl");
private final static QName _TemplateReplacementsInvisibleForPosition_QNAME = new QName("http://www.opencyc.org/xml/formulaTemplate/", "templateReplacementsInvisibleForPosition");
private final static QName _IsEditable_QNAME = new QName("http://www.opencyc.org/xml/formulaTemplate/", "isEditable");
private final static QName _MultipleEntry_QNAME = new QName("http://www.opencyc.org/xml/formulaTemplate/", "multipleEntry");
private final static QName _Silk_QNAME = new QName("http://www.opencyc.org/xml/proofView/", "silk");
/**
* Create a new ObjectFactory that can be used to create new instances of schema derived classes for package: com.cyc.xml.query
*
*/
public ObjectFactory() {
}
/**
* Create an instance of {@link ProofViewEntry }
*
*/
public ProofViewEntry createProofViewEntry() {
return new ProofViewEntry();
}
/**
* Create an instance of {@link Content }
*
*/
public Content createContent() {
return new Content();
}
/**
* Create an instance of {@link SubEntries }
*
*/
public SubEntries createSubEntries() {
return new SubEntries();
}
/**
* Create an instance of {@link ProofView }
*
*/
public ProofView createProofView() {
return new ProofView();
}
/**
* Create an instance of {@link ConstraintOnReplacement }
*
*/
public ConstraintOnReplacement createConstraintOnReplacement() {
return new ConstraintOnReplacement();
}
/**
* Create an instance of {@link GlossText }
*
*/
public GlossText createGlossText() {
return new GlossText();
}
/**
* Create an instance of {@link CandidateReplacementForPosition }
*
*/
public CandidateReplacementForPosition createCandidateReplacementForPosition() {
return new CandidateReplacementForPosition();
}
/**
* Create an instance of {@link ArgumentPositionDetail }
*
*/
public ArgumentPositionDetail createArgumentPositionDetail() {
return new ArgumentPositionDetail();
}
/**
* Create an instance of {@link ArgPosition }
*
*/
public ArgPosition createArgPosition() {
return new ArgPosition();
}
/**
* Create an instance of {@link Position }
*
*/
public Position createPosition() {
return new Position();
}
/**
* Create an instance of {@link Explanation }
*
*/
public Explanation createExplanation() {
return new Explanation();
}
/**
* Create an instance of {@link Ordering }
*
*/
public Ordering createOrdering() {
return new Ordering();
}
/**
* Create an instance of {@link ConstraintsOnReplacement }
*
*/
public ConstraintsOnReplacement createConstraintsOnReplacement() {
return new ConstraintsOnReplacement();
}
/**
* Create an instance of {@link CandidateReplacementsForPosition }
*
*/
public CandidateReplacementsForPosition createCandidateReplacementsForPosition() {
return new CandidateReplacementsForPosition();
}
/**
* Create an instance of {@link UnknownTermForTemplatePosition }
*
*/
public UnknownTermForTemplatePosition createUnknownTermForTemplatePosition() {
return new UnknownTermForTemplatePosition();
}
/**
* Create an instance of {@link ExcludeReplacementOptions }
*
*/
public ExcludeReplacementOptions createExcludeReplacementOptions() {
return new ExcludeReplacementOptions();
}
/**
* Create an instance of {@link ExcludeReplacementOption }
*
*/
public ExcludeReplacementOption createExcludeReplacementOption() {
return new ExcludeReplacementOption();
}
/**
* Create an instance of {@link TemplateId }
*
*/
public TemplateId createTemplateId() {
return new TemplateId();
}
/**
* Create an instance of {@link Example }
*
*/
public Example createExample() {
return new Example();
}
/**
* Create an instance of {@link FollowUp }
*
*/
public FollowUp createFollowUp() {
return new FollowUp();
}
/**
* Create an instance of {@link Connective }
*
*/
public Connective createConnective() {
return new Connective();
}
/**
* Create an instance of {@link LoadMt }
*
*/
public LoadMt createLoadMt() {
return new LoadMt();
}
/**
* Create an instance of {@link ArgPositions }
*
*/
public ArgPositions createArgPositions() {
return new ArgPositions();
}
/**
* Create an instance of {@link ReformulationSpecification }
*
*/
public ReformulationSpecification createReformulationSpecification() {
return new ReformulationSpecification();
}
/**
* Create an instance of {@link FormulaTemplate }
*
*/
public FormulaTemplate createFormulaTemplate() {
return new FormulaTemplate();
}
/**
* Create an instance of {@link Id }
*
*/
public Id createId() {
return new Id();
}
/**
* Create an instance of {@link CyclQuery }
*
*/
public CyclQuery createCyclQuery() {
return new CyclQuery();
}
/**
* Create an instance of {@link QueryID }
*
*/
public QueryID createQueryID() {
return new QueryID();
}
/**
* Create an instance of {@link QueryFormula }
*
*/
public QueryFormula createQueryFormula() {
return new QueryFormula();
}
/**
* Create an instance of {@link QueryMt }
*
*/
public QueryMt createQueryMt() {
return new QueryMt();
}
/**
* Create an instance of {@link QueryComment }
*
*/
public QueryComment createQueryComment() {
return new QueryComment();
}
/**
* Create an instance of {@link QueryInferenceProperties }
*
*/
public QueryInferenceProperties createQueryInferenceProperties() {
return new QueryInferenceProperties();
}
/**
* Create an instance of {@link QueryInferenceProperty }
*
*/
public QueryInferenceProperty createQueryInferenceProperty() {
return new QueryInferenceProperty();
}
/**
* Create an instance of {@link PropertySymbol }
*
*/
public PropertySymbol createPropertySymbol() {
return new PropertySymbol();
}
/**
* Create an instance of {@link PropertyValue }
*
*/
public PropertyValue createPropertyValue() {
return new PropertyValue();
}
/**
* Create an instance of {@link QueryIndexicals }
*
*/
public QueryIndexicals createQueryIndexicals() {
return new QueryIndexicals();
}
/**
* Create an instance of {@link QueryIndexical }
*
*/
public QueryIndexical createQueryIndexical() {
return new QueryIndexical();
}
/**
* Create an instance of {@link Formula }
*
*/
public Formula createFormula() {
return new Formula();
}
/**
* Create an instance of {@link Elmt }
*
*/
public Elmt createElmt() {
return new Elmt();
}
/**
* Create an instance of {@link FocalTerm }
*
*/
public FocalTerm createFocalTerm() {
return new FocalTerm();
}
/**
* Create an instance of {@link UsageExamples }
*
*/
public UsageExamples createUsageExamples() {
return new UsageExamples();
}
/**
* Create an instance of {@link FollowUps }
*
*/
public FollowUps createFollowUps() {
return new FollowUps();
}
/**
* Create an instance of {@link GlossForTemplate }
*
*/
public GlossForTemplate createGlossForTemplate() {
return new GlossForTemplate();
}
/**
* Create an instance of {@link ArgumentPositionDetails }
*
*/
public ArgumentPositionDetails createArgumentPositionDetails() {
return new ArgumentPositionDetails();
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Object }{@code >}}
*
*/
@XmlElementDecl(namespace = "http://www.opencyc.org/xml/formulaTemplate/", name = "validationRequired")
public JAXBElement