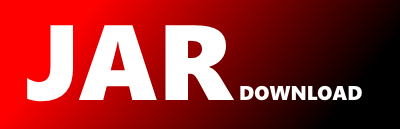
com.cyc.query.client.BindingsBackedQueryAnswer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cyc-query-client Show documentation
Show all versions of cyc-query-client Show documentation
Query API implementation for requesting and handling answers to arbitrarily complex questions
posed to a Cyc server.
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package com.cyc.query.client;
/*
* #%L
* File: BindingsBackedQueryAnswer.java
* Project: Query Client
* %%
* Copyright (C) 2013 - 2017 Cycorp, Inc.
* %%
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* #L%
*/
import com.cyc.kb.KbTerm;
import com.cyc.kb.Variable;
import com.cyc.kb.client.KbObjectImpl;
import com.cyc.kb.exception.CreateException;
import com.cyc.nl.Paraphrase;
import com.cyc.nl.ParaphraseImpl;
import com.cyc.nl.Paraphraser;
import com.cyc.query.InferenceAnswerIdentifier;
import com.cyc.query.ParaphrasedQueryAnswer;
import com.cyc.query.exception.QueryRuntimeException;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Set;
/**
*
* @author baxter
*/
class BindingsBackedQueryAnswer implements ParaphrasedQueryAnswer {
private final Map bindings;
private final Map paraphrasedBindings;
private final InferenceAnswerIdentifier id;
private final Paraphraser paraphraser;
public BindingsBackedQueryAnswer(Map bindings) {
this(bindings, null, null);
}
public BindingsBackedQueryAnswer(Map bindings,
InferenceAnswerIdentifier id) {
this(bindings, id, null);
}
public BindingsBackedQueryAnswer(Map bindings,
InferenceAnswerIdentifier id, Paraphraser paraphraser) {
this.bindings = bindings;
this.id = id;
this.paraphraser = paraphraser;
this.paraphrasedBindings = new HashMap<>();
}
@Override
public Set getVariables() {
return bindings.keySet();
}
@Override
public T getBinding(Variable var) {
try {
return KbObjectImpl.checkAndCastObject(bindings.get(var));
} catch (CreateException ex) {
throw new RuntimeException(ex);
}
}
@Override
public boolean hasBinding(Variable var) {
return (bindings.containsKey(var));
}
@Override
public Map getBindings() {
return Collections.unmodifiableMap(bindings);
}
@Override
public Paraphraser getParaphraser() {
return paraphraser;
}
@Override
public InferenceAnswerIdentifier getId() {
if (id == null) {
throw new UnsupportedOperationException();
} else {
return id;
}
}
@Override
public String toString() {
if (id == null) {
return "Query Answer with " + bindings.size() + " Binding"
+ ((bindings.size() == 1) ? "" : "s");
} else {
return id.toString();
}
}
@Override
public Set getSources() {
if (getId() == null) {
throw new QueryRuntimeException("Unable to get sources for BindingsBackedQueryAnswer without an inference answer id");
}
return new InferenceAnswerBackedQueryAnswer(getId()).getSources();
}
@Override
public Paraphrase getBindingParaphrase(Variable var) {
if (!(paraphraser instanceof Paraphraser)) {
throw new UnsupportedOperationException("Unable to paraphrase when no paraphraser has been provided.");
}
return paraphraser.paraphrase((getBinding(var)));
}
@Override
public Map getParaphrasedBindings() {
if (paraphrasedBindings.size() != bindings.size()) {
for (Map.Entry binding : bindings.entrySet()) {
if (!paraphrasedBindings.containsKey(binding.getKey())) {
Paraphrase paraphrase = (paraphraser == null) ? new ParaphraseImpl(null, binding.getValue()) : paraphraser.paraphrase(binding.getValue());
paraphrasedBindings.put(binding.getKey(), paraphrase);
}
}
}
return paraphrasedBindings;
}
@Override
public List toPrettyBindingsStrings() {
final List results = new ArrayList();
int longestVarStrLength = 0;
for (Variable var : getVariables()) {
final int varStrLength = var.toString().length();
longestVarStrLength = (varStrLength > longestVarStrLength)
? varStrLength
: longestVarStrLength;
}
for (Variable var : getVariables()) {
results.add(
String.format("%1$-" + longestVarStrLength + "s", var.toString())
+ " = " + getBinding(var));
}
return results;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy