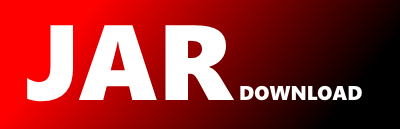
com.daioware.net.http.request.HttpPostRequest Maven / Gradle / Ivy
package com.daioware.net.http.request;
import java.io.File;
import java.io.IOException;
import java.io.UnsupportedEncodingException;
import java.net.MalformedURLException;
import java.net.URL;
import com.daioware.net.http.request.reader.FileReader;
import com.daioware.net.http.request.reader.Reader;
import com.daioware.net.http.request.reader.StringReader;
public class HttpPostRequest extends HttpRequest{
private File bodyFile;
private String bodyStr;
private Reader reader;
public HttpPostRequest(String url,String encoding) throws MalformedURLException {
this(new URL(url),encoding);
}
public HttpPostRequest(URL url, String encoding) {
super(url, encoding);
}
public HttpPostRequest(String url) throws MalformedURLException {
this(new URL(url));
}
public HttpPostRequest(URL url) throws MalformedURLException {
super(url);
}
public File getBodyFile() {
return bodyFile;
}
public String getBodyStr() {
return bodyStr;
}
public void setBodyFile(File bodyFile) {
this.bodyFile = bodyFile;
if(this.bodyFile!=null) {
bodyStr=null;
reader=new FileReader(bodyFile);
}
}
public void setBodyStr(String bodyStr) throws UnsupportedEncodingException {
this.bodyStr = bodyStr;
if(bodyStr!=null) {
bodyFile=null;
reader=new StringReader(bodyStr,getEncoding());
}
}
@Override
public boolean startReadingBody() throws IOException {
return getBodyStr()!=null || getBodyFile()!=null;
}
@Override
public void stopReadingBody() throws IOException {
reader.close();
}
@Override
public int readBody(byte[] bytes, int offset, int length) throws IOException {
return reader.read(bytes, offset, length);
}
@Override
public long getContentLength() {
return reader.size();
}
@Override
public String getMethod() {
return "POST";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy